Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial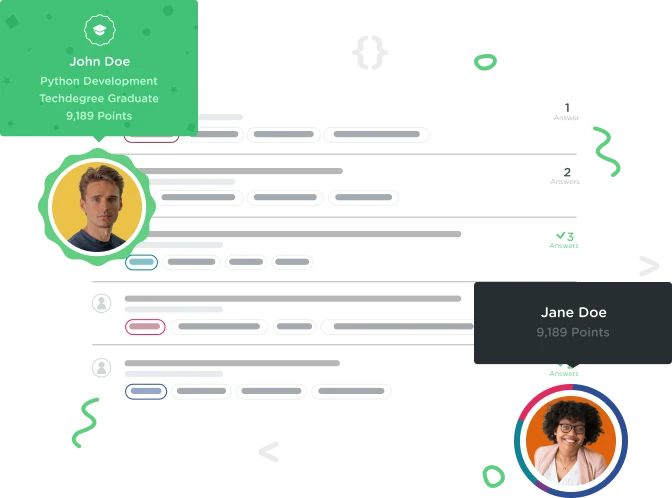
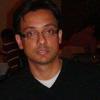
Mohammad Aslam
6,053 PointsChanging a string of words into a dictionary of words and word frequency.
What am I doing wrong?
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
list_string = string.split(" ")
for word in list_string:
dictionary = {}
key = word.lower()
value = list_string.count(word)
dictionary[key] = value
return dictionary
2 Answers

Wade Williams
24,476 PointsYou need to define your dictionary variable outside of your loop. The first thing your loop does is overwrite your dictionary with an empty dictionary.
def word_count(string):
list_string = string.split(" ")
dictionary = {}
for word in list_string:
key = word.lower()
value = list_string.count(word)
dictionary[key] = value
return dictionary
Some optimizations you might consider is lowercasing the entire string from the start, so you don't have to lower it within your loop or create a key variable
list_string = string.lower().split(" ")
Instead of calling the count function I would keep a running count, this is because the count function goes through the entire list to count the number of times that letter is in the list everytime. On a really large list this would be very time consuming. A suggested alternative would be:
def word_count(string):
words = string.lower().split(" ")
dictionary = {}
for word in words:
if word in dictionary:
dictionary[word] += 1
else:
dictionary[word] = 1
return dictionary
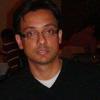
Mohammad Aslam
6,053 PointsThanks, really appreciate it
Mohammad Aslam
6,053 PointsMohammad Aslam
6,053 PointsCan you please explain the use of the "+=" like where you use it, its function?
Wade Williams
24,476 PointsWade Williams
24,476 PointsThe "+=" is short-hand for adding a value to a variable and assigning the result back to the original variable.
For instance, in our problem we're counting the number of words in a string, so every time we come across a word already in our dictionary we want to add 1 to the count, which we could do by:
dictionary[word] = dictionary[word] + 1
This is a very common operation, so most programming languages have come up the short-hand "+=" for this operation. There is also "-=" to subtract, "*=" to multiply and "/=" to divide.