Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial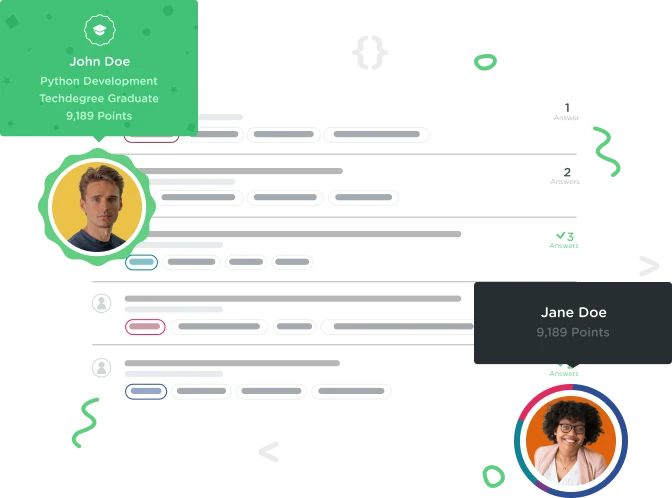

Justo Montoya
3,799 PointsChecking the value of an index in a list and skipping the iteration depending on the value of that index.
Hey guys, I'm having trouble on the coding challenge exercise where I use keyword CONTINUE to skip the 0-th index of a list if it is "a". The exercise says its similar to the one before this one where if a list index equals "STOP" then we BREAK the loop so I assumed I would use a FOR-loop but i'm having trouble.
def loopy(items):
for item in items:
if item=="a":
continue
print(item)
else:
print(item)
I used this loop think that since it would stat iterating at the 0-th index it would check its value and skip it if it were "a" but I realize it would also skip other "a"-values at non 0-th index in the list.
def loopy(items):
i=0
while(i!=len(items)):
if items,index("a")==0:
continue
i+=1
print(items[i])
else:
print(items[i])
i+=1
I thought using a WHILE-loop might help to specify to skip the 0-th index if it is "a" but this code is also wrong.
Not sure how to do this especially if its supposed to be similar to the BREAK code challenge.
2 Answers
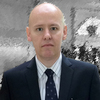
Nathan Tallack
22,160 PointsYeah, and that is how we SHOULD do it for the challenge, because it is teaching us to think of how continue works. But it is not actually needed, and adds complexity which you should avoid in the normal world.
It would look like this.
def loopy(items): # Start my loop over the list
for item in items: # Grab each item out of the list
if item[0] == "a": # If the item starts with the letter a
continue # Restart the for loop with the next item
print(item) # Print it out
So what we are doing differently is evaluating if it DOES start with a and restarting the loop if it does. This avoids printing it out.
You could also do it this way:
def loopy(items): # Start my loop over the list
for item in items: # Grab each item out of the list
if item[0] == "a": # If the item starts with the letter a
continue # Restart the for loop with the next item
else: # Else if it does not start with the letter a
print(item) # Print it out
But these ways of using continue would only make sense if there were other instructions inside the for loop that you would not want executed. If it was just the print, then you could do the above and leave the continue out because it would skip the print in the else and that would be the end of the loop.
One thing to remember is there is always MANY MANY ways of doing things. It is just a matter of understanding what all those different ways are so that you can choose the best way when you are writing your own code and recognise what someone else has done when reading their code. :)
Be patient. I am only a few weeks ahead of you and the reward of sticking it out and absorbing all the content here on treehouse is HUGE. I would still consider myself a beginner programmer but I am already able to do so much. It blows my mind how much I will be able to do once I get past these beginner stages. :)
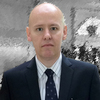
Nathan Tallack
22,160 PointsI imagine there are quite a few ways to do this, but here is what I did.
I figured "Well, I want to focus on the printing." So here is what it looks like.
def loopy(items): # Start my loop over the list
for item in items: # Grab each item out of the list
if item[0] != "a": # If the item does NOT start with the letter a
print(item) # Print it out
No need for the continue then because if it will loop back all by itself. In this way the items starting with the letter a will not be printed, and everything else will be. ;)

Justo Montoya
3,799 Pointsthank you, this worked. I'm still pretty frustrated by the coding challenge though because the instructions of the exercise led me to believe that it wanted me to practice using the CONTINUE keyword.
"Same idea as the last one. My loopy function needs to skip an item this time, though. Loop through every item in items. If the current item's index 0 is the letter "a", continue to the next one. Otherwise, print out every member of items."
Is there a way to do it using the CONTINUE keyword?
Justo Montoya
3,799 PointsJusto Montoya
3,799 PointsThank you for this explanation.
I would not have known to do this because I would've thought that doing " item[0]=="a" " was not correct since the "item" in the for loop is the variable assigned to each of the indexes of the "items" list.
so in this case "item" is also the name of a list?
Nathan Tallack
22,160 PointsNathan Tallack
22,160 PointsYeah. That is another conceptual leap that once you make it will come naturally later.
In the case of this challenge, the function loopy takes in a list of strings, assigning it to the variable items.
So imagine the list of items we are passing in look like this.
[ "tree", "apple", "dog", "house", "arm" ]
So if this list was passed into your loopy function you would end up with apple and arm not being printed out but tree dog and house being printed out.
One of the great thing about strings in Python is they are indexable. So each time your for loop runs though, we take one of those strings and consider its first character via the index 0.
"tree"[0] == "t"
Each time the for loop goes through and gets each item (string) from items (list of strings) we consider the first character (index 0) in each of those strings.
As with all things there are LOTS of ways you could do this other than matching that first index character. For example, the String type in Python comes with a method (that is a built in function) called startswith. You could use that to do the same thing I would imagine.
Rather than this:
item[0] == "a"
You could do this:
item.startswith("a")
I am sure my excitable babble is only confusing you more than helping. But what I am trying to get across here is the crazy power in having so many ways to do things. That is what treehouse is trying to teach you here. All the different ways of doing things. Not because the way they show you is the ONLY way or the BEST way. Just that it is A WAY to do it. :)