Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial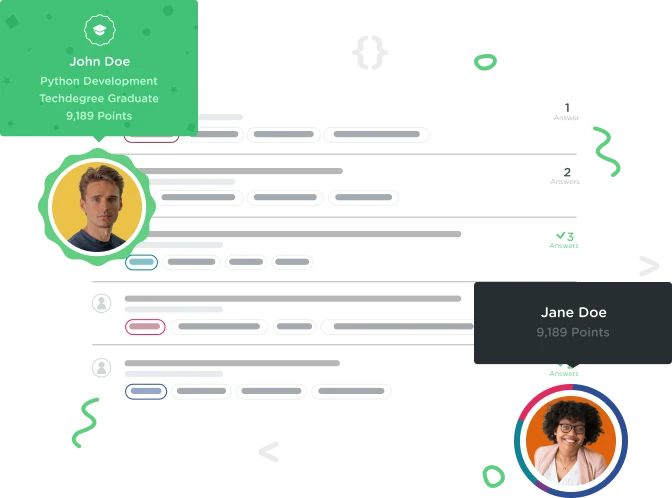

Johannes Scribante
19,175 PointsCode challenge: am I missing a part of the question requirements?
Here is the code challenge: Alright, this one might be a bit challenging but you've been doing great so far, so I'm sure you can manage it. I need you to make a function named word_count. It should accept a single argument which will be a string. The function needs to return a dictionary. The keys in the dictionary will be each of the words in the string, lowercased. The values will be how many times that particular word appears in the string. Check the comments below for an example.
E.g. word_count("I do not like it Sam I Am") gets back a dictionary like: {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1} Lowercase the string to make it easier.
When I run the function locally with these inputs: print(word_count("I do not like it Sam I Am")) print(word_count("Where Did aLl the bEArs go bears bears bears"))
The output is: {'i': 2, 'do': 1, 'not': 1, 'like': 1, 'it': 1, 'sam': 1, 'am': 1} {'where': 1, 'did': 1, 'all': 1, 'the': 1, 'bears': 4, 'go': 1}
So my question is, hard to define, but I am not sure why the code challenge is not getting the expected output.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(unit_string):
word_list = unit_string.lower().split(' ')
word_count_dict = {}
# default to 0
for word in word_list:
word_count_dict[word] = 0
# loop again to add
for word in word_list:
word_count_dict[word] += 1
return word_count_dict
2 Answers

Afloarei Andrei
5,163 Pointsremove the single quotes from split(' ') leave just split()

Afloarei Andrei
5,163 Points'split()' is in its default state and by default it spleats white spaces.
Johannes Scribante
19,175 PointsJohannes Scribante
19,175 PointsIt worked! Thank you! Would you perhaps be able to explain why this works? Or better yet, what is the difference between the following (python console)?
a = 'this is a string'
a.split()
['this', 'is', 'a', 'string']
a.split(' ')
['this', 'is', 'a', 'string']
In (a) I don't see much difference here
b = 'this;is;a;string'
b.split()
['this;is;a;string']
b.split(';')
['this', 'is', 'a', 'string']
In (b) it makes sense to me
I would just like to wrap my head around why there is this difference