Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial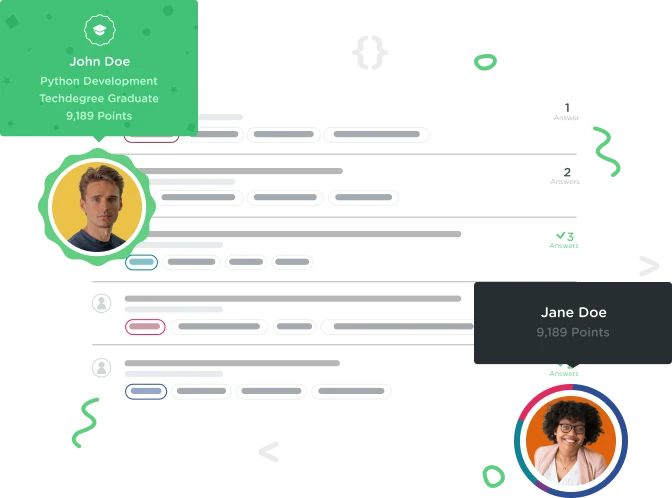

Rodney Vese
Courses Plus Student 1,112 PointsCode Challenge not working.
Not sure why this isn't working in the code challenge. Tested in workspaces and get correct string returned from list.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(teachers):
newlist = []
for teacher in teachers:
classes = [teacher,len(teachers[teacher])]
newlist.append(classes)
for i in newlist:
values = []
num = i[1]
values.append(num)
high = max(values)
for sublist in newlist:
if sublist[1] == high:
return sublist[0]
break
1 Answer

Fenimore Cooper
3,591 PointsThe first problem that I see is that you initialize the 'values' list within your for loop. This means that the list value will be reset for each list iteration -- which in turn means that the last item on the list will always be mistaken for the max & get stored in the high variable.... that is, it would be a problem if the loop looped which at this point, it doesn't.
It doesn't loop because you create a second for loop to iterate over the same list, (which, by the way, probably means it should all be within the same loop) what that second loop does (because it starts after one iteration of the first loop) is iterate over only the first item in the list, & then, since it will match the high value so far it returns it.
In short, you have created a function that returns the name of the first teacher in the dictionary.
Your code does work, with some minor modifications... notice the new placement of values, and the changed indentation of your second for loop, also the 'break' is not needed.
All this can be done in a more straightforward way, so I'll show you that below as well.
def most_classes(teachers):
newlist, values = [], []
for teacher in teachers:
classes = [teacher,len(teachers[teacher])]
newlist.append(classes)
for i in newlist:
num = i[1]
values.append(num)
high = max(values)
for sublist in newlist:
if sublist[1] == high:
return sublist[0]
# this is a more straightforward solution
def most_classes(dict_in):
most_classes = 0
busiest_teacher = ''
for entry in dict_in:
if len(dict_in[entry]) > most_classes:
most_classes = len(dict_in[entry])
busiest_teacher = entry
else:
continue
return busiest_teacher