Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial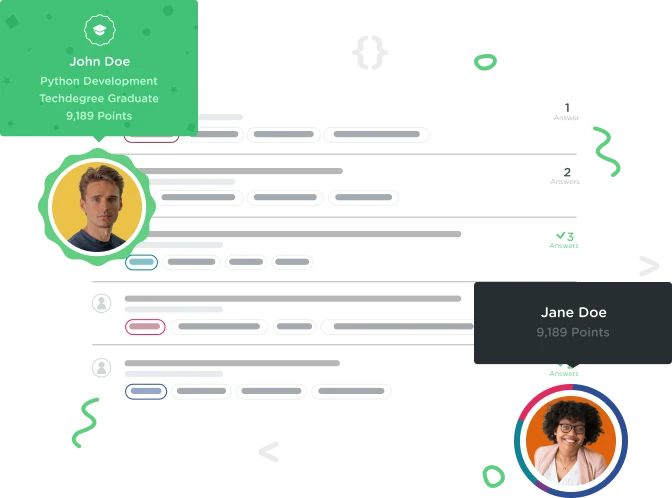

William J. Terrell
17,403 PointsCode Challenge: Refactor Using a Loop
The question asks to do the following:
The code below logs all of the even numbers from 2 to 24 to the JavaScript console. However, there's a lot of redundant code here. Re-write this using a loop.
console.log(2);
console.log(4);
console.log(6);
console.log(8);
console.log(10);
console.log(12);
console.log(14);
console.log(16);
console.log(18);
console.log(20);
console.log(22);
console.log(24);
My code is posted below.
Any help is appreciated.
Thanks!
for ( var i = 1; i <= 24; i * 2 ) {
console.log( i );
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
6 Answers

John Magee
Courses Plus Student 9,058 PointsTwo things
- You don't have to use var in a loop definition
- The third portion of your loop declaration needs to increment i - you need to INCREASE the value of i - the code you have written is not actually increasing i - it just returns the same number every time

Stephen Minotti
4,114 PointsThis gave me trouble also. I went back in my notes to the first lesson on for loops and found the answer. The correct code is...
for ( i = 2; i <= 24; i += 2 ) { console.log ( i ); }

John Magee
Courses Plus Student 9,058 PointsI don't want to give you the entire answer, but my suggestion is to reread what the output should be and then take a look at what your function will output. The answer should become apparent.

William J. Terrell
17,403 PointsWell, I changed it to:
for (var i = 2; i <= 24; i + 2) {
console.log(i)
}
And it killed Google when I tried running it in the console; so, is there something wrong with the < = 24 ? But I thought that part of the code told it to run until i < = 24 (meaning that, once i > 24, the code would stop).

Roberto Cardenas
10,478 Pointsi + 2 is missing something.

William J. Terrell
17,403 PointsI got it to work. I forget exactly the code I used; I think I changed 2 to a 1, but I was using i + number when I should have been using i += number. Once I changed it to +=, it worked!
Thanks!
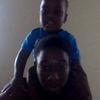
kmorrison
5,090 Pointsthats the wrong answer

Caden Ingram
6,482 PointsNo it's not, he was clever enough to find that out.