Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial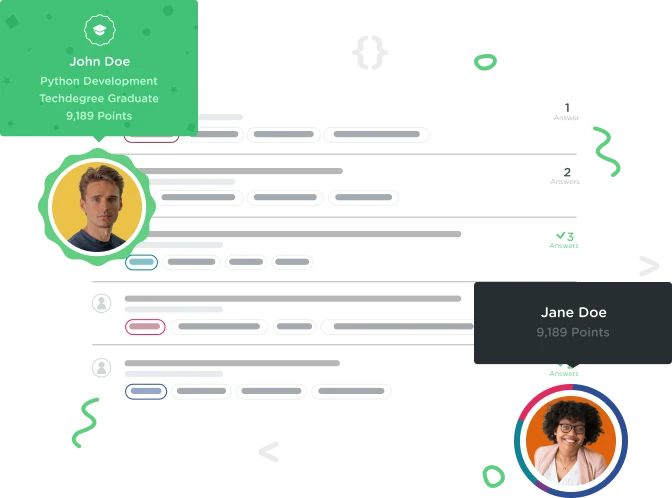

matthias papsdorf
Courses Plus Student 4,152 PointsCode does not run always without error
When testing this code in pyCharm or elsewhere the first time the code runs fine. How ever, when rerunning the script if gives out an error: Key Error: 3.
I am not quite sure why that is happening.
cheers, Matthias
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(teach_dictp):
max_count = 0
new_dict = {}
for k in teach_dictp:
course_count = len(teach_dictp[k])
if course_count > max_count:
max_count = course_count
new_dict = {course_count : k}
print(new_dict)
teacher = new_dict[max_count]
return(teacher)
3 Answers
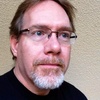
Chris Freeman
Treehouse Moderator 68,441 PointsA KeyError occurs when looking up a value in a dictionary with a key that does not exist, as shown in ipython output below:
In [1]: somedict = { 'life' : '42' }
In [2]: somedict['meaning']
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
<ipython-input-2-e7ec203b08f3> in <module>()
----> 1 somedict['meaning']
KeyError: 'meaning'
Your KeyError: 3
means your new_dict
did not have the key 3
when the lookup occurred.
Inspecting your code, if I'm reading the indent of your code correctly, it appears you assign new_dict
regardless of the outcome of the previous if statement. Perhaps you want to do:
def most_classes(teach_dictp):
max_count = 0
new_dict = {}
for k in teach_dictp:
course_count = len(teach_dictp[k])
if course_count > max_count:
max_count = course_count
new_dict = {course_count : k} # <-- Added indentation
print(new_dict)
teacher = new_dict[max_count]
return teacher # <--- parens are not needed
Alternatively, since you only need to return the name of the teacher, you could simply track their name and not use a dictionary:
def most_classes(teach_dictp):
max_count = 0
max_teacher = None
for k in teach_dictp:
course_count = len(teach_dictp[k])
if course_count > max_count:
max_count = course_count
max_teacher = k
return teacher

matthias papsdorf
Courses Plus Student 4,152 PointsHi Chris,
thanks a lot for the answer. I originally intended to have new_assigned all the times, but assign max_count only if len(teach_dictp[k]). But seeing your answer, that is actually smarter than my approach since there you don't need another dict.
One thing though I did not understand was, that first time the code ran fine, then when rerunning the code (no changes) it came back with an error and then again it would run fine.
Cheers, Matthias
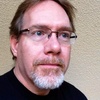
Chris Freeman
Treehouse Moderator 68,441 PointsIt depends on how your are rerunning it and may depend on the data used in testing.
As stated above, the KeyError
is caused solely by a using a nonexistent value as a dictionary key. Your code isn't changing, so that leaves variations in your test data or the initialized state of your code on iterations of testing to cause the KeyError. Not knowing your data or whether PyCharm is reseting completely between runs, I can't know for sure. If you were using the exact same argument value for in your call to most_classes(teacher_dict)
then the behavior should be consistent.
In your original code new_dict
was assigned every loop iteration. Any teacher checked after the previous maximum would always get assigned to new_dict
and their possibly-less-than-max value would be the only key in the dict. Assigning teacher
on each iteration would then fail because the max-value key would no longer be in the dict.

matthias papsdorf
Courses Plus Student 4,152 PointsGood point, I'll add a test for each loop to see if something odd is happening to the new dict.