Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial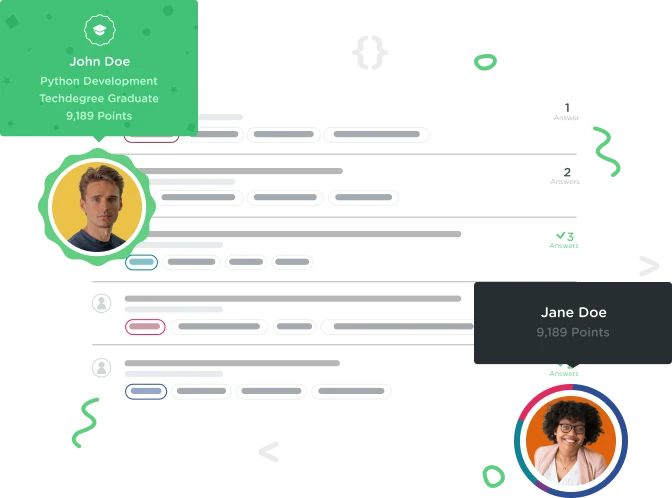

Moe Himed
1,244 PointsCode not working?
Here is my code:
var correct = 0;
var guess1 = prompt('How many days are in November? Use words')
if (guess1.toUpperCase() === 'THIRTY' ); {
correct += 1;
}
var guess2 = prompt('How many days are in July? Use words')
if (guess2.toUpperCase() === 'THIRTYONE' ); {
correct += 1;
}
var guess3 = prompt('How many days are in February? Use words')
if (guess3.toUpperCase() === 'TWENTYEIGHT' ); {
correct += 1;
}
var guess4= prompt('How many days are in August? Use words')
if (guess4.toUpperCase() === 'THIRTYONE' ); {
correct += 1;
}
var guess5= prompt('How many days are in April? Use words')
if (guess4.toUpperCase() === 'THIRTY' ); {
correct += 1;
}
//Results
document.write("<p>You got " + correct + " out of 5 questions right.</p>");
if ( correct === 5) {
document.write("<p><strong> You earned a gold medal!</strong> </p>");
}
else if ( correct >= 2) {
document.write("<p><strong> You earned a silver medal!</strong> </p>");
}
else (correct <= 2) {
document.write("<p> <strong> You earned a bronze medal!</strong> </p>");
}
If I delete everything under //Results, the code works and the pop-up window comes up. But if the code is there, I get no pop-ups. Any help is appreciated.
MOD EDIT: added markdown for code readability.
2 Answers
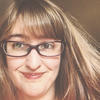
Melissa Austin
3,383 PointsThe if statement should end in an else with no condition as this statement will run if all other statements are false. See https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/if...else
//Results
document.write("<p>You got " + correct + " out of 5 questions right.</p>");
if ( correct === 5) {
document.write("<p><strong> You earned a gold medal!</strong> </p>");
} else if ( correct >= 2) {
document.write("<p><strong> You earned a silver medal!</strong> </p>");
} else {
document.write("<p> <strong> You earned a bronze medal!</strong> </p>");
}
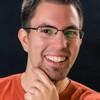
Robert Szabo
7,999 PointsAlso in your 'if statement' when you define if an answer is true or false, you should lose the semicolon before the braces. I see you've put semicolons in each 'if statement'. I don't know exactly why but this messes up the number of the total correct answer. So even if your answer is false to the questions, the program thinks your answer is true and gives you a point. Here is what I mean:
var correct = 0;
var guess1 = prompt('How many days are in November? Use words')
if (guess1.toUpperCase() === 'THIRTY' ); { //Here the correct line would be: if (guess1.toUpperCase() === 'THIRTY') {
correct += 1;
}
//Once again the full statement in the correct form
var correct = 0;
var guess1 = prompt('How many days are in November? Use words')
if (guess1.toUpperCase() === 'THIRTY' ) {
correct += 1;
}