Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial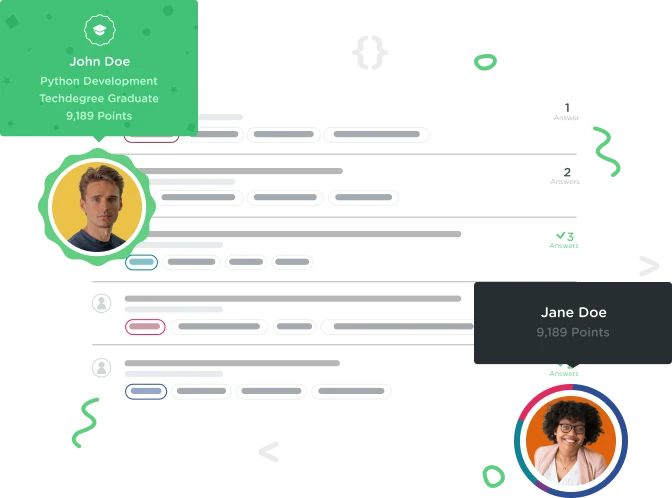

Stanley Hataria
1,168 PointsCode not working in console.
Task 1 of the code challenge.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(dicts):
max_count=0
teacher=''
for key in dicts:
for value in dicts.values():
y=len(value)
teacher=key
if max_count<y:
max_count=y
teacher=key
else:
continue
return teacher
5 Answers
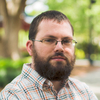
Kenneth Love
Treehouse Guest TeacherYour two for
loops are, likely, going through two different orders of the dict. Remember, dictionaries aren't guaranteed to come out in the same order every time. You're also reassigning teacher
every time, whether they have a larger count of courses or not. These two things are probably what's having the most impact on your output being wrong.

Rodrigue Loredon
1,338 PointsI have the same problem but I can't figure out what's wrong with my code. What is there to do to avoid the dictionary order pitfall? The functions returns someone different than Jason Seifer: Dave MacFarland or other people. I've been on this for 24 hours, I would appreciate some help please.
teachers = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(teachers):
max_count = 0
course_number = 0
best_teacher =''
for teacher in teachers:
for courses in teachers.values():
course_number = len(courses)
if max_count < course_number:
max_count = course_number
best_teacher = teacher
return best_teacher
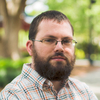
Kenneth Love
Treehouse Guest TeacherJust a bit before this challenge, we talked about getting out two variables for each iteration through the dict. We had to use the .items()
method, too. Do you remember how we did that?

Rodrigue Loredon
1,338 PointsKenneth, I watched again the videos preceding that challenge and unless I'm mistaken, we haven't talked about the .items() method at this point of the course.
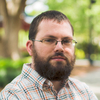
Kenneth Love
Treehouse Guest TeacherYou're right, that comes later.
Anyway, you don't need multiple for
loops. In your for teacher for teachers:
loop, you already have the teacher. You can use that teacher to look up that teacher's courses from the dict. Then you can len()
that and you'll know how many courses that teacher has. No second loop needed.

Stanley Hataria
1,168 PointsYou are looping over two dictionaries. Try using a for loop for a dictionary and a list which the values() gives out.

Rodrigue Loredon
1,338 PointsWhere you able to pass that challenge?

Stanley Hataria
1,168 PointsYes I was able to pass the challenge. I was making the mistake of looping over the dictionary twice. As you must have seen that dictionaries order is not specific. I think you should remove the first for loop for teachers and add a for loop below the values() Such that it iterates the list. I think this should make it work.

Rodrigue Loredon
1,338 PointsThanks Stanley, in the meantime I was able to pass the challenge with some help, thanks a lot. Take care.

Stanley Hataria
1,168 PointsNo problem. Glad you got through.
Stanley Hataria
1,168 PointsStanley Hataria
1,168 PointsThanks for that, I forgot about the order for dictionary.