Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial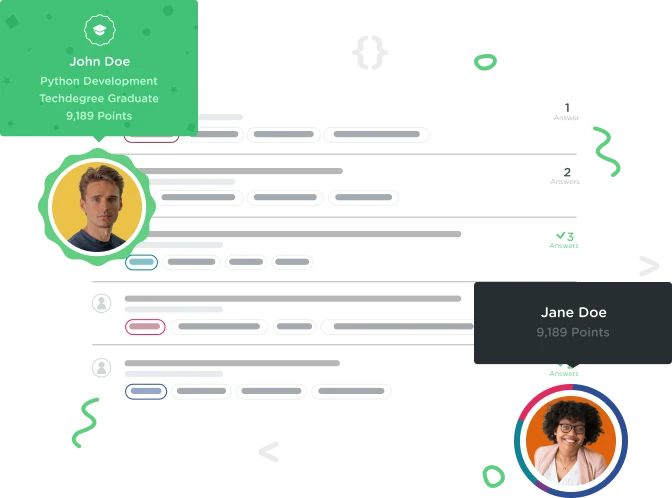

William Lapp
426 PointsCode works, but the answer is incorrect
I've tried to answer the question about using a "try:," "except ValueError:," and "else:" blocks and actually ran several different versions of this problem that work well in python. The easiest one is to input the objects as floating point variables; for example, first=float(input("First number: ")). However, the question asks us to perform the error trapping routine before the final step in which the string inputs are transformed into floating point numbers. One way to do this and to get feedback as soon as a non-number is input is to use the attached code. However, I am being told that step 1 no longer works; it does in fact, but the program that evaluates the code does not seem to "think so." So, I am wondering if anyone can steer me in the right direction with respect to this item and thereby allow me to continue on with the tutorial. Thanks, Bill
def add(first, second):
try:
first=input("What is the first number? ")
third=float(first)
second=input("What is the second number? ")
fourth=float(second)
except ValueError:
print("Not a number!")
return None
else:
first=float(first)
second=float(second)
return first + second
3 Answers
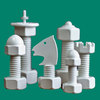
Steven Parker
240,449 PointsThe issue is that the function should not ask for input since the values it needs are passed to it as arguments.
I'd guess you had no input statements when you passed tasks 1 and 2.
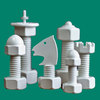
Steven Parker
240,449 PointsYou only need to remove the input statements, they don't need to be replaced with anything. The code already converts the arguments from string using float.

William Lapp
426 PointsWell Steven, It turned out that the input statements did need to be replaced with something; in particular, first=float(first), and second=float(second). One may never have guessed it, based on the structure of the question that asks you to perform the error trapping routine before the variables are transformed into floating point variables, but that seemed to be the case because the answer was then marked as correct. However, your answer was the best. Thank you for your input! Bill
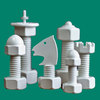
Steven Parker
240,449 PointsYou could optionally make the code more "DRY" by re-using the same names and then you wouldn't need to do it again in the "else" section. But just removing the inputs is enough to pass the challenge, since the current conversions are all that is needed for the "try" to work.

William Lapp
426 PointsWell Steven,
I tried just removing the input statements, but the code didn't pass. So, I simply put in the statements: (a) first=float(first) and (b) second=float(second) in the try statement before the except statement. That should not have worked, based on the structure of the problem wherein it is stated that the error trapping should occur before the variables are transformed into floating point numbers. However, just taking out the input statements left nothing for the try statement to try. That is why, previously, I tried to make two new variables that I could use in a numerical format to try the test (e.g., third=int(first), fourth=int(second), return third + fourth; that actually worked and solved the problem as stated, but it was not accepted as an answer. I thought maybe I could do the same thing with the original variables, but realized that if they were in a floating point format, I would destroy it using the int statement, and fool myself into thinking that I had preserved all of the potential information in the variable when I transformed the variables into floating point numbers in the final step. I also thought that maybe I should assume that the numbers might already be in floating point or some other numerical form, so I tried the simple code of: (a) try:, and (b) indented "return first + second. That was not correct. In desperation, or just plain resignation, I tried transforming the variables into floating point numbers in the try step before the except step and to my complete surprise, the code was marked as correct and I could finally move on. Of course, the answer is not correct relative to the stipulations of the question, but I was glad to move forward!
The first question in the next section asked me to write a for loop that printed all of the items in a list. Okay, I tried, and, oh, "Bummer!" So, intuitively using the (il)logic that I had learned from the previous section, I simply inserted an indented "print(items)" (not in quotes in the code; the quotes were used here to let the reader know that this was the code) and what do you know, that was marked as the correct answer! Of course, the next question involved putting in a break point, so some kind of a "for loop" or maybe a "while loop" would be useful. I haven't found the combination that works yet; albeit, I have written code that works like a charm, I cannot get one that is marked as "correct." Therefore, I am unsubscribing from the treehouse and seeking other sources.
This is not to say that I did not gain some really useful knowledge about python from this tutorial; I clearly did, and I really liked the videos! Kevin Love has a great depth of knowledge about this subject and I am sorry that I will not be able to learn more from him, but I really want to learn how to do it, right now, so that I can program the cluster analysis algorithm program that I have in mind. What I have seen is that python is pretty much another form of BASIC, several versions of which I learned long ago; "quick BASIC" was a great advance because we didn't have to use line numbers for goto and gosub commands, and you could label your subroutines with catchy names that identified what was transpiring. All of the concepts of loops, arrays, inputs, outputs, mathematical operations, etc. are completely transferable and it is just a question of learning the syntax. I can and have programmed advanced statistical programs like "maximal decomposition" (a kind of "anti"-factor analysis in which the first item of each factor are "cherry-picked" to form a minimal set of maximally predictive variables), but my project requires python and I really want to program in python because it helps me reenter the conversation with more contemporary developers. I just cannot go on in this forum because I am pretty sure I cannot skip to a new section before I complete the challenge questions; whereas, for me, I would be quite satisfied with writing code that works instead of code that conforms to one programmer's way of solving the problem. So, I wish you all good luck, and thank you for sharing your thoughts and community with me.
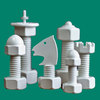
Steven Parker
240,449 PointsI'm unable to replicate your results. I pasted in your original code and then removed the two input statements, leaving this:
def add(first, second):
try:
third=float(first)
fourth=float(second)
except ValueError:
print("Not a number!")
return None
else:
first=float(first)
second=float(second)
return first + second
Then doing nothing else but clicking on "Check Work" and "Next Task ->", it passed all 3 steps of the challenge.
And you can skip past a challenge without solving it just by using the buttons over the video window or by going back to the course index page.
And lastly, remember that the challenges have specific performance requirements, so code that "works like a charm" outside of the challenge might not be accomplishing the same objective as the challenge requires. If you change your mind about continuing, you can always ask additional questions here when you run into those issues.
William Lapp
426 PointsWilliam Lapp
426 PointsThanks for the quick reply! So, what I think you are saying is that if I omit the input statements, then the code may run, though I worry about what I should replace "it" with...one idea is to declare the transformation of a possible string variable into a mathematically calculable commodity; that is, first=int(first). Do you agree that this code will permit the use of the return statement to perform the mathematical operation of addition? I'd like to hear more from you about your conceptualization on how we might conceptualize the problem and orchestrate an informational solution.