Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial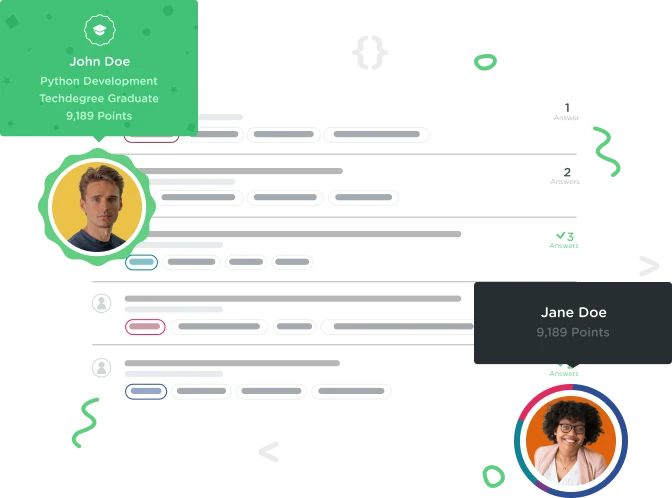

Kelly Walsh
3,642 PointsCombining variables?
What I find most difficult is that I don't break the problem down enough and think that I can combine several steps into one. For example, in this challenge, I tried to use parseInt() as part of the first variable prompt even though we didn't learn any syntax that looked this:
var userNumOne = prompt (parseInt("Please input a number"));
Eventually I broke it into two and came to:
var userNumOne = prompt("Please input a number");
var firstNum = parseInt(userNumOne);
BUT, now i'm just curious; is there is way to use parseInt with the prompt within userNumOne?
I hope I explained this correctly.
Thanks! :-)
2 Answers
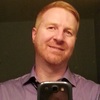
Ion Jones
11,299 PointsYes, you can totally use parseInt() with a prompt. The only thing missing from your example above is the radix. It should look like this:
var userNumOne = prompt (parseInt("Please input a number"),10);
Here's a link to more information regarding parseInt and the radix: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/parseInt

Kelly Walsh
3,642 PointsThank you both for the responses. parseInt does need to go before the prompt; otherwise, the prompt pops up with an NaN error in it. I had to think about this for a minute because it seems like it should work the other way around. However, you can't have letters immediately following parseInt. parseInt doesn't have a way to know that that is your actual prompt.
In my effort to simplify and cut down on the number of variables used in the problem, I ended up with the following:
var input1 = parseInt(prompt("Please type a starting number.")); var input2 = parseInt(prompt("Please type an ending number.")); var random = Math.floor(Math.random() * (input2 - input1 + 1)) + input1; document.write(random + " is a number between " + input1 + " and " + input2 + ".");
Ryan Field
Courses Plus Student 21,242 PointsRyan Field
Courses Plus Student 21,242 PointsActually, in this case, it's not because he's missing the radix, but because he's mixing up the order. It should look like this:
var userNumOne = parseInt(prompt("Please input a number"),10);
According to the MDN, though, as you point out, it is a good idea to include the radix; although none of the courses I've taken here thus far have included it.