Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial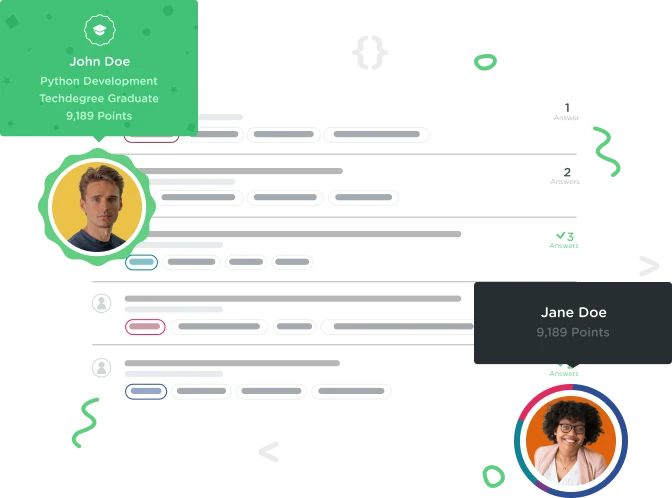

Yi Ho Wong
6,377 PointsComment on extra challenges
My code can work to print more than one student in case and can alert if no student named XXX was found. I would like to see if anything I could do to improve the code. Thanks. :)
var message = '';
var student;
var search;
var studentGroup = [];
var html = [];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
report += '<p>';
return report;
}
while( true ) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.toLowerCase() === "quit") {
break;
}
for (var i = 0; i < students.length; i++) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()){
studentGroup.push(student);
}
}
for (var i = 0; i < studentGroup.length; i++) {
html += getStudentReport(studentGroup[i]);
print(html);
}
if (studentGroup.length === 0) {
alert("We don't have that student in our system.");
}
}
2 Answers

Ryan Zimmerman
3,854 PointsI would take your logic and wrap it in a function for a few reasons:
- You won't need a while true, you could just invoke the function and when done or needs to finish you could just "return"
- The other is that when you have any business logic that is executing on the front end you want to keep it in the scope of a function so when the operation is over someone will not have access to it.
- The less global variables you have the better.
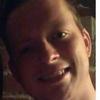
David Kanwisher
9,751 PointsGood idea with the array, definitely using the tools we have learned so far! I bet you could use true false boolean to make this work without arrays.
Yi Ho Wong
6,377 PointsYi Ho Wong
6,377 Pointsthanks for your comment:)