Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial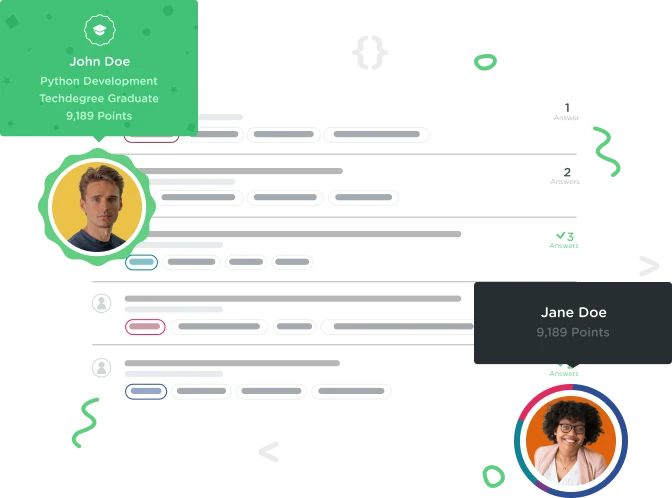

Leo Marco Corpuz
18,975 PointsCommon courses challenge
Here's my code. Any advice? Thanks!
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(topics):
common_list=[]
for subject in COURSES:
common_subject=topics.intersection(subject)
common_list.append(common_subject)
return common_list
1 Answer
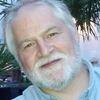
Jeff Muday
Treehouse Moderator 28,726 PointsYour approach definitely shows you know something about Python sets. Let's build on that.
- I see you wanted common_list to be all courses matching your topics set.
Great idea, keep it.
- You iterate through the COURSES set with a "for" loop.
Keep this idea-- but let's mod it slightly.
modification: you will want to use the COURSES.items() iterator. It's not the only way to do things, but it is advantageous because it returns BOTH the course_name and the course_topics. This is an important pattern Kenneth would like you to remember.
- I like that you look for an intersection of topics -- well played, but let's change it a little.
modification: look for an intersection with course_topics instead.
- I like you are doing the common_list.append. This is good, but we need to change it a little.
modification: Let's change it to ONLY append the course name when the length of the common_subject is greater than zero (which indicates our topics matched/intersected with course_topics. This will avoid us having empty sets appended to our list.
- After the loop you return the common list. Well played, this is right.
def covers(topics):
common_list=[]
for course_name, course_topics in COURSES.items():
common_subject=topics.intersection(course_topics)
if len(common_subject) > 0:
common_list.append(course_name)
return common_list
Good luck on your Python journey. It can be a little frustrating at times, but pays off well!