Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial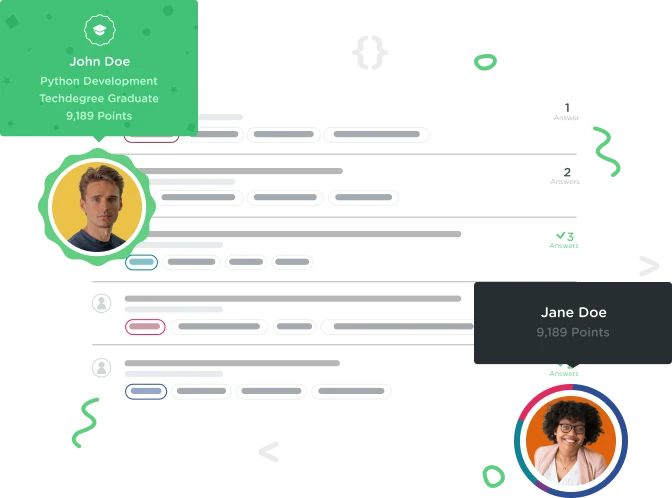

Rachel Heneault
12,401 PointsComparious
const laws = document.getElementsByTagName('li'); const indexText = document.getElementById('boldIndex'); const button = document.getElementById('embolden');
button.addEventListener('click', (e) => { const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (index === indexText.value) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
My answer isn't' correct, stuck on this but I am also wonder what the 10 on: const index = parseInt(indexText.value, 10);
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (index == indexText.value) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>Newton's Laws</title>
</head>
<body>
<h1>Newton's Laws of Motion</h1>
<ul>
<li>An object in motion tends to stay in motion, unless acted on by an outside force.</li>
<li>Acceleration is dependent on the forces acting upon an object and the mass of the object.</li>
<li>For every action, there is an equal and opposite reaction.</li>
</ul>
<input type="text" id="boldIndex">
<button id="embolden">Embolden</button>
<script src="app.js"></script>
</body>
</html>
2 Answers
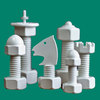
Steven Parker
231,275 Points
You are comparing a number to a string.
indexText.value is a string, but you are comparing it to the number index. Also, remember that index is the number that the string was converted into. What you want to compare here is the numeric value index against the loop's index value (i).
The value 10
given as the second argument to parseInt is the conversion radix. Since 10
is the default, this second argument could be omitted without affecting the program.

andren
28,558 PointsYour answer is quite close but the index variable holds the int version of indexText.value
so you are actually comparing the same value against itself. But there is another index value at play in the loop that you might want to compare against instead...
The second argument passed to the parseInt
function determines the radix, or put in simpler terms, the number system that you want the int to be parsed as. 10 means to treat it as Base-10 which is more commonly known as the decimal system (the system most humans use). If you don't specify a radix it usually defaults to 10 anyway so this second parameter is usually not supplied. Though supplying it guarantees that your code won't be messed up if the radix is defaulted to something else in some odd implementation of JavaScript.
If the hint about the solution I gave above does not help you then I have posted the solution for you to look at here.