Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial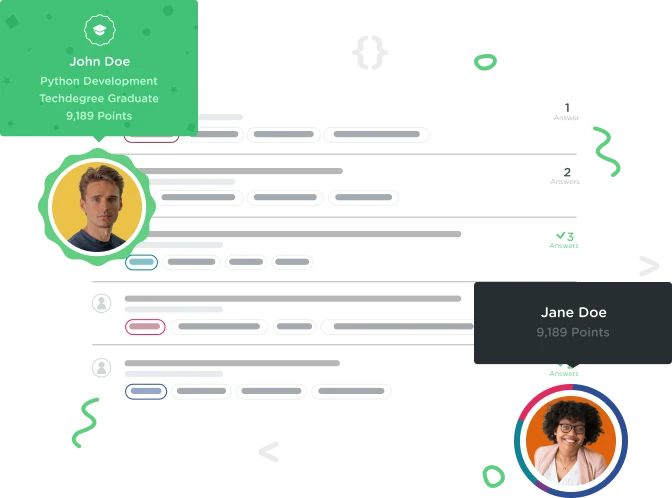

Keith Branch
7,717 Points"completed_at" showing the wrong date and time
Running this code:
<table class="todo_items">
<% @todo_list.todo_items.each do |todo_item| %>
<tr id="<%= dom_id(todo_item) %>">
<td><%= todo_item.completed_at %></td>
<td><%= todo_item.content %></td>
<td>
<% if todo_item.completed_at.blank? %>
<%= link_to "Mark Complete", complete_todo_list_todo_item_path(todo_item), method: :patch %>
<% end %>
<%= link_to "Edit", edit_todo_list_todo_item_path(todo_item) %>
<%= link_to "Delete", todo_list_todo_item_path(todo_item), method: :delete, data: { confirm: "Are you sure?" } %>
</td>
</tr>
<% end %>
</table>
on this spec:
context "with completed items" do
let!(:completed_todo_item) { todo_list.todo_items.create(content: "Eggs", completed_at: 5.minutes.ago) }
it "shows completed items as complete" do
visit_todo_list todo_list
within dom_id_for(completed_todo_item) do
expect(page).to have_content(completed_todo_item.completed_at)
end
end
it "does not give the option to mark complete" do
visit_todo_list todo_list
within dom_id_for(completed_todo_item) do
expect(page).to_not have_content("Mark Complete")
end
end
end
And I'm getting this error:
1) Editing todo items with completed items shows completed items as complete
Failure/Error: expect(page).to have_content(completed_todo_item.completed_at)
expected to find text "2016-05-17 02:40:07 UTC" in "2000-01-01 02:40:07 UTC Eggs Edit Delete"
# ./spec/features/todo_items/complete_spec.rb:23:in `block (4 levels) in <top (required)>'
# ./spec/features/todo_items/complete_spec.rb:22:in `block (3 levels) in <top (required)>
2 Answers
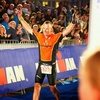
Steve Hunter
57,712 PointsHi Keith,
Did you get round this issue?
The 'defaulting to the year 2000' thing is often caused with date and time columns. Often when a Time gets changed to a DateTime.
There's some comment on the issue in this and this post. It'll often show as being correct in the database, but when you pull the data into the view, the date will appear incorrectly.
Steve.

Montalvo Miguelo
24,789 PointsLook at your migration... correct type :datetime
class AddCompleteAtToTodoItems < ActiveRecord::Migration
def change
add_column :todo_items, :completed_at, :datetime
end
end