Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial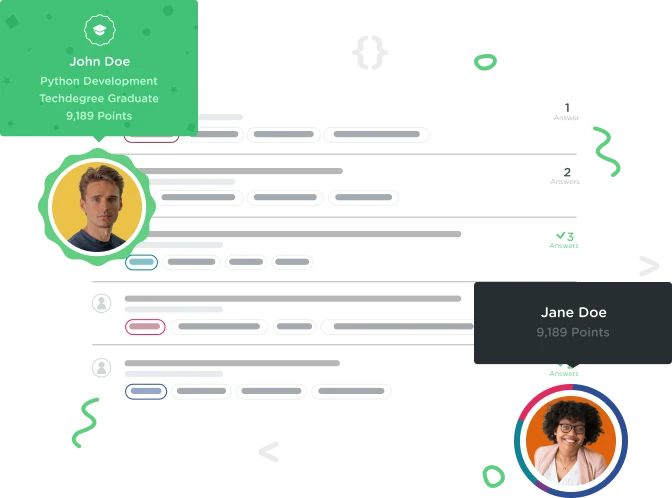
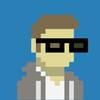
Zach Perry
1,303 PointsCompletely lost on Scope and Functions? Any other way to explain them?
I've been becoming more and more lost at the concepts of C. I completely do not understand the concepts of Scope and Functions. It's very confusing. Could someone possibly explain them and their code in a better way? Any help is greatly appreciated. Thank you for your time!
3 Answers

Jeremy Hayden
1,740 PointsZack,
Lets break it down a bit.
First lets talk about functions and their purpose. A function is a block of code that has a name. This block of code can be called upon to perform a specific task over and over.
In the video, he used the following as a function.
void scope_it_out (){
YOUR CODE TO RUN GOES HERE
}
scope_it_out is now a block of code that can be run anytime it is referenced later in the program. For example you could run the function simply by calling its name: scope_it_out();
Now for Scope
You have probably learned already that variables once set, can be referenced or changed. for example:
int x = 5;
int y = 10;
int z = x*y; //we know z=50
x=10; // we changed x to 10
z = x * y; // we know z now equals 100
This works because all these variables were declared in the same scope. If x were to be changed outside this scope it would not affect the x in this scope. It can be demonstrated in the following example:
int x = 5;
int y;
for (y = 0; y < 3; y++)
{
int x = 25;
NSLog (@"Varible x in for loop is %i", x); // this will print out that x is equal to 25
}
NSLog (@"Varible x in for loop is %i", x); // this will print out that x is equal to 5
Here we see two instances of the variable x being declared. First in the main body where int x=5.
And second in the for loop where x=25.
Even though both variables are called x, they are actually two separate variables. This is because the second variable x is declared inside the scope of the for loop. It only exists inside the loop.
Scope refers to variables only affecting the "container" that they are declared in.
How do functions and scope relate?
Functions are their own "containers". They have their own scope. So variables declared inside a function do not affect or change a variable outside the function even if it has the same name. That is why we have to pass the value of the variable into and out of functions if we want to use it.
I hope this helps a little
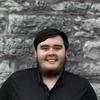
Michael Hulet
47,913 PointsI'll start with functions. These are essentially blocks of code that can be run by typing a single line, instead of typing the block over and over again. For example, the C code you'd write for a program is in a function named main()
. Below is an example of a function that would take in 2 int
s, add them together, and send back the sum:
int addTwoNumbers(int first, int second){
int sum = first + second;
return sum;
}
Note that all functions are declared outside of any other functions, such as main
. The way that you would run that code in the function is something like this:
int main(){
int one = 1;
int two = 2;
int sum = addTwoNumbers(one, two);
printf("The sum of %d and %d is %d", one, two, sum);
//The main function always returns 0, just to tell the operating system that everything ran properly
return 0;
}
In this case, the numbers 1 and 2 were added together by the addTwoNumbers()
function I previously defined, and the sum (returned by my function) was assigned to the variable sum
.
You an think of functions as a way to have the computer copy and paste your code in a certain spot.
Now onto the concepts of scope. Did you notice how I defined two variables named sum
in my previous example? That's perfectly legal. This is because they're each only in their own function, and they're therefore only visible to the function they're defined in. In other words, the sum
in the function main()
is totally different from the sum
in the function addTwoNumbers()
. They can equal different things, and even be different data types. They're totally independent of each other.
Now let's say that I defined the sum
variable outside of any function, like this:
int sum = 0;
int addTwoNumbers(int first, int second){
//Notice how I don't write "int" before "sum"
sum = first + second;
return sum;
}
int main(){
int one = 1;
int two = 2;
//Same as above, notice the lack of "int"
sum = addTwoNumbers(one, two);
printf("The sum of %d and %d is %d", one, two, sum);
return 0;
}
Although this example should end in the same result as the first, what's happening is different. Instead of each function having its own unique variable named sum
, each function is operating on the same variable. I could write another function that uses the sum
variable, and it'd be operating on the same variable as the other 2 functions. That because in this case, sum
is declared a the Global scope. That means any part of your code anywhere in that file can see it and change it. This is as opposed to the Local scope, like in the first function, where each sum
variable was only visible to its own respective function.
Loops have their own scope, too. Any variable defined in a loop is only available to that loop. Take this code for example:
//This statement is only necessary if you want to copy and paste this verbatim. You'll need this if you do the same with any of the rest of my examples
#include <stdio.h>
int main(){
int number = 0;
for(int i = 0; i < 10; i++){
//If you're not familiar with the "++" operator, all it does is add one to the variable it's next to
number++;
int incremented = number;
printf("number + 1 = %d. This is iteration %d", incremented, i);
}
return 0;
}
Go ahead and copy and paste this code into a blank C file and run it.
In that example, incremented
is only available inside that loop. If I tried to refer to it outside the loop (even if it was after the loop), the compiler wouldn't know what I was talking about, and the code wouldn't compile. However, notice that I was allowed to refer to number
just fine inside the loop, even though it was defined before the loop. This is just fine, because it's still defined in the same function as the loop. It would also be available in the loop if it was a Global variable. Also, on a side note, notice how I was allowed to use i
in the loop (not outside, however, because it's technically defined in the loop). Although it may look like it has its own scope (because it's defined in the parentheses), it's really at the same scope as incremented
.
It's not just for(){}
loops that have their own scope. This is true in while(){}
and do{}while():
loops, too.
Essentially, scope gets more specific with each nested block of code. That means that variables defined in a certain block of code are only available to that block and other blocks inside of said block, like loops within functions, like above. You can access any variable defined in your scope or higher, but not lower than your scope.
On a final note, this means that you must also be careful about your variable names as you get more specific in scope. Because every variable at a higher scope is available to any lower scope, you can't define a variable in a lower scope that has already been defined in a higher one. This, for example, is illegal:
int number = 0;
int main(){
//The following definition is illegal, because of the "int" keyword
int number = 1;
for(int i = 0; i < 10; i++){
//This next declaration is illegal for the same reason as the previous one
float number = 3.5;
printf("This program will have crashed by now");
}
return 0;
}
I hope that all made sense. Just reply to this if you have any questions

Jeremy Hayden
1,740 PointsLol, we had very similar train of thought
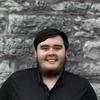
Michael Hulet
47,913 PointsI know. I started typing this before yours was posted, too (though you finished 25 minutes before me)

Jeremy Hayden
1,740 PointsI like how you explained that not only functions and loops have their own scope, but also while and do/while loops.
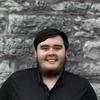
Michael Hulet
47,913 PointsFor what it's worth, yours was a pretty great answer, too. I tend to try to be really verbose and cover everything possible, but it sometimes ends up being a lot to read. You got the point across a lot faster than me (also, kudos for having read and successfully utilized the Markdown Cheatsheet! I don't see that too often)
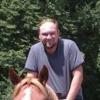
Paul Yorde
10,497 PointsHi Zach,
A short answer would be:
A function is a programming concept/tool that allows us to manipulate words and numbers (data).
Next, think of the scope on a rifle. It allows you to see a limited, but precise view of area.
That's what Scope is.
When we apply the idea of Scope to variables, we get words and numbers(data) that can only be seen at certain times and in certain ways.
Cheers ~