Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial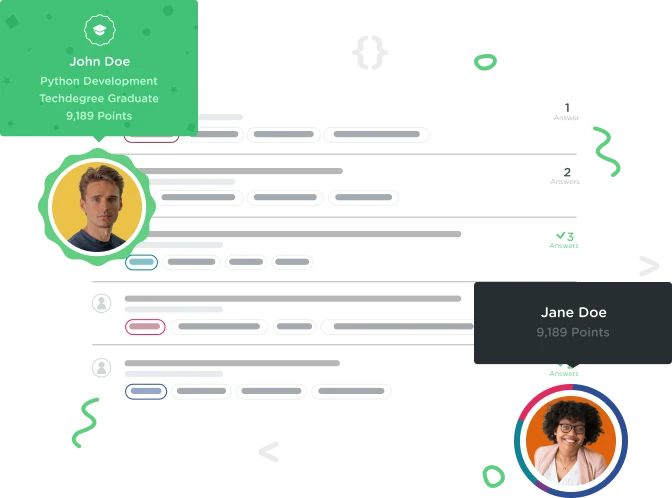

Omar Shehadeh
1,683 PointsComputing value of sum
Now that we have the while loop set up, it's time to compute the sum! Using the value of counter as an index value, retrieve each value from the array and add it to the value of sum.
For example: sum = sum + newValue. Or you could use the compound addition operator sum += newValue where newValue is the value retrieved from the array.
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
[counter += 1] = sum + 2 + 8 + 1 + 16 + 4 + 3 + 9
}
1 Answer
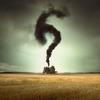
Jordan Barr
21,335 PointsHi Omar,
Your code is not quite right, as with each iteration (while the counter is less than the number of items in the array) you are performing that massive sum of numbers. The challenge is asking to iterate over the array and with each iteration you will add another number from the next index value of the array to the sum of all values.
So part 1 of the challenge asks to set up the while loop, and it looks like you did that correctly but then have modified it in the part 2 so it is not right. Here is what the part 1 while loop should look like (setting up the condition of the while loop and the counter inside)
while counter < numbers.count {
counter += 1 // remember this is the same as writing "counter = counter + 1"
}
So with each increment of the loop +1 will be added to the counter and the loop will stop when the counter is 1 less than the number of items in the array (remember the index of an array starts at 0)
part 2 requires you to compute the sum of the array, to get the value from an array at a specific index we use:
numbers[0] //this retrieves the first value
numbers[1] // the second value
so we can actually use the value of the counter variable as the index of the array to retrieve the values which will look like this:
while counter < numbers.count {
sum += numbers[counter] // sum += numbers[counter] is the same as writing "sum = sum + numbers[counter]"
counter += 1
}
just remember to compute the sum before incrementing the counter variable, otherwise you will miss the first value at index 0
hope this helps =]