Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial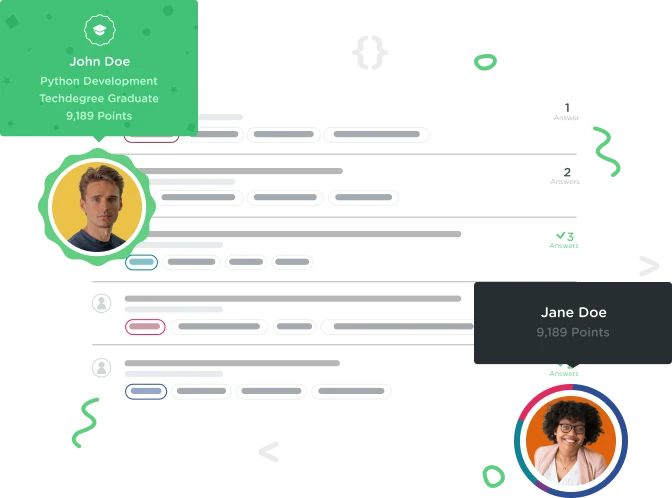

Lenka Zajic
3,970 PointsConditional Challenge question
var money = 9; var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' || money < 9 ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' || money < 9 ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>

Lenka Zajic
3,970 PointsOops, sorry forgot that part. Here's the challenge and then my response. It keeps telling me to check the last else if clause but maybe there's more wrong than that. Point is, I can't figure it out :(
Something's wrong with this script. The value in the variable money is only 9. But if you preview this script you'll see the "Time to go to the theater" message. Fix this script so that it correctly tests the money and today variables and prints out the proper alert message: "It's Friday, but I don't have enough money to go out"
var money = 9; var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' && money > 9) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}

Lenka Zajic
3,970 PointsWeird! All I had to do was add the = sign next to the < sign and it worked. Why? The second else if clause didn't include the = sign next to the > sign... Thanks though! :)

Jason Anello
Courses Plus Student 94,610 PointsHi Lenka,
I know you have this solved now but I wanted to point out that it's easier if you don't put a money condition on the last else if. If you've reached that point then you know you don't have enough money. There's no point in checking to make sure it's not enough. You only need to check that it's Friday.
If you do want to have a money condition in there then the correct one would check for money less than or equal to 10. You want to make sure that there are no gaps in the money value. The previous condition checks for money greater than 10 so you have to cover everything less than or equal to 10.
} else if ( today === 'Friday' && money <= 10 ) {
Rather than taking a chance there and making a mistake it's better to not put it in. Only check that it's Friday.
In general, you should only put in as many conditions as you need to properly implement the logic. Each new piece of code that you add to your program is another chance to make a mistake.
" If debugging is the process of removing software bugs, then programming must be the process of putting them in. " - Edsger W. Dijkstra
3 Answers

Garrett Mutchler
6,749 PointsAssuming your question is what's wrong with the code, here's a hint: the conditions in the code are all set up to trigger if you have a certain amount of money "OR" it's Friday. It looks like you need the conditions to ask if you have a certain amount of money "AND" it's Friday.

Lenka Zajic
3,970 PointsHere's the question. Your help got me closer but it keeps saying to check the last else if clause...
"Something's wrong with this script. The value in the variable money is only 9. But if you preview this script you'll see the "Time to go to the theater" message. Fix this script so that it correctly tests the money and today variables and prints out the proper alert message: "It's Friday, but I don't have enough money to go out""
var money = 9; var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' && money > 9) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}

Garrett Mutchler
6,749 PointsIt looks like your last else-if statement now says to trigger when money > 9, when in your original post it said less-than.
Though considering that the variable 'money' is exactly 9 in this instance, you'll probably want to use a different value or something like less-than-or-equal-to anyway.

Falk Schwiefert
8,706 PointsThe problem is not the last else, its the last else if. Because you are checking if money is more than 9. But money is 9 so that statement is not true. That means the final condition is triggered and the alert would be "it's not Friday". But since it is Friday that isn't the alert the check mechanism looks for.
I have done this exercise a while ago, so I don't remember the exact solution but try money less than or equal to 9 and see what happens.
Falk Schwiefert
8,706 PointsFalk Schwiefert
8,706 PointsI am curious what your question is. :)