Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial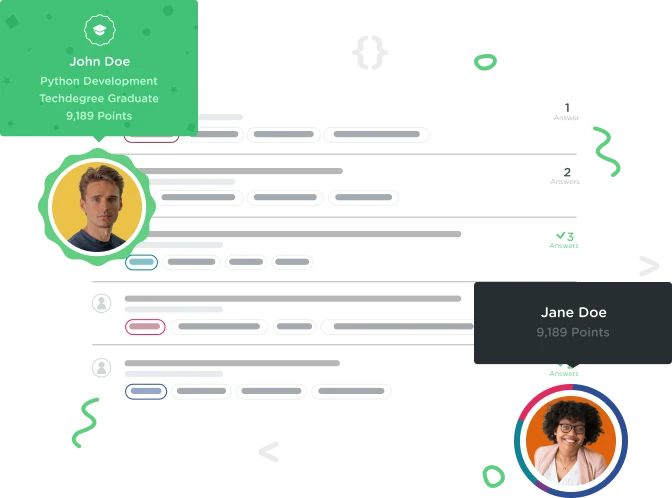

Aaron Mesh
1,001 PointsConfused about the error issue?
setup
Alright, last step but it's a big one. Make a while loop that runs until start is falsey. Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99. If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number. Finally, decrement start by 1. I know it's a lot, but I know you can do it!
There Error message I'm getting is "Wrong number of prints". But the problems say to print for even and then print again for odds. am I getting this error because all of this is in a while loop and it just keeps printing continuously? If so how can I fix this? Do i just need to add a "continue" under the print statements?
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start == True:
rnum = random.randint(1, 99)
if even_odd(rnum) == True:
print("{} is even".format(rnum))
else:
print("{} is odd".format(rnum))
start -= 1
1 Answer
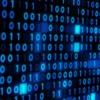
Alexander Davison
65,469 PointsI found that if you remove the == True
part of the while
condition will fix the case.
This is because start
is truthy, not true
itself. When you compare a plain old number with a boolean, they are always different. But since start
is truthy, it will be treated as true
when you just use it itself as a condition.
I know it is a little confusing... I used to get so frustrated on this too :)
I hope you understand. If you got any questions, please ask below
~Alex
Aaron Mesh
1,001 PointsAaron Mesh
1,001 PointsSo it should look like 'while start = true"? Because I tried that and got a new error saying task 1 is no longer being completed
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsIt should be just
while start
. I know it's weird :)Here's the right code: