Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial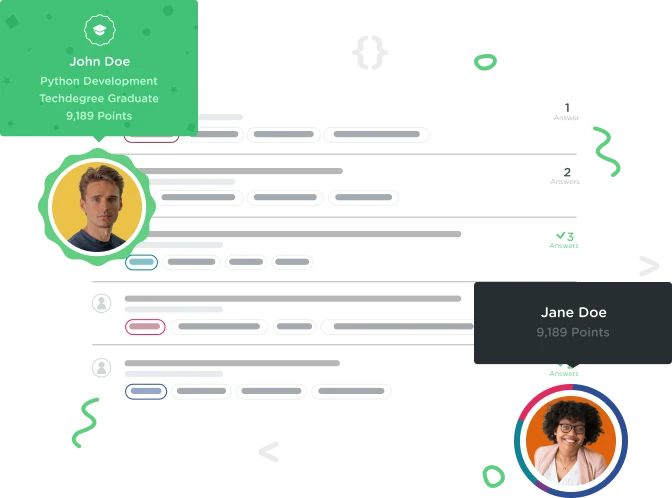

Devon Thompson
4,301 PointsConsole.log Loops
I'm not sure what I am doing wrong. It states that only 11 numbers were logged and I know it's probably an easy fix but I'm not sure what to do. Help, please!
for ( var i = 2; i >= 2 && i < 24; i += 2) { console.log(); }
for ( var i = 2; i >= 2 && i < 24; i += 2) {
console.log();
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
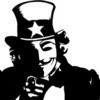
behar
10,799 PointsYou should be console.log(i). And the reason its only printning 11 numbers is because you told the loop to stop When i is 24, but you want to log 24, so change it to i < 25 or i <= 24. Hope this helps!
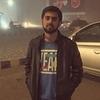
zainsra7
3,237 PointsLet's try to understand this with a simple example first:
Print/log numbers from 1 to 10 using for loop?
for( var i = 1 ; i <=10 ; i++) {
console.log(i);
}
A for loop has 3 parts :
- Initialization of counter variable (i) // We do this only at the start of for loop i = 1;
- Check condition of loop , i < = 10 that is execute for loop body till counter variable is less than or equal to 10. Or in other words stop executing loop once counter variable becomes 11.
- Increment counter variable. i ++ in this case increment by 1 , like 1 , 2 , 3 ,4 , 5 till 10.
Now in your problem, you need to print numbers from 2 to 24 and you already know how to print numbers from 1 to 10 so use the same strategy:
- Initialize counter variable with 2
- As you need to cover all even numbers smaller than 24 and also including 24 so you will use , while i <=24 or i != 26
- As you need to print even numbers like 2,4,6,8,10 ... so you will increment by 2 which is i+=2 or i = i + 2;
Final Solution :
for( var i = 2; i < = 24 ; i +=2){
console.log(i);
}
OR
for( var i = 2; i != 26 ; i +=2){
console.log(i);
}
why 26 tho ? I'll leave that for you to figure out :)
I hope it clears your question , Happy Coding !