Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial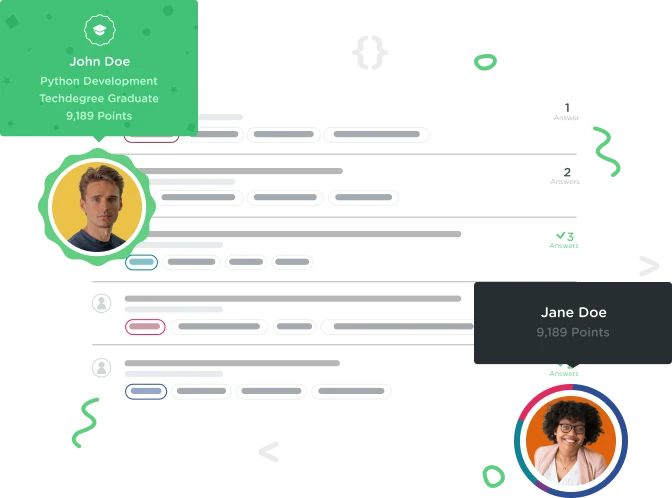

Brandyn Lordi
17,778 PointsConstructor method? When/why?
Im trying to figure out constructor methods mentioned in the teachers notes and when/why to use them. In the video, Alena creates the following method in a class :
public function setTitle($title) {
$this->title = ucwords($title);
}
Is this a constructor method or am i required to use the function __construct(){}
syntax for it to be a constructor?
1 Answer
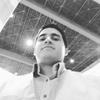
Victor Avelar
3,393 PointsThe constructor is called by the PHP interpreter every time you create a new instance of a class, the function you share is called setter and it has to be called by the programmer in the code like $class->setter($value)
, and has to be called by the user, a setter and the __contruct function can also play together and nor of them are required by the other to work.
I think this example can make things clear.
<?php
namespace Foo\Pet;
class Pet
{
/**
* @var string
*/
protected $name;
/**
* Pet class constructor.
*
* @param string $name
*/
public function __construct(string $name)
{
$this->name = $name;
}
/**
* @param string $name
*/
public function setName(string $name)
{
$this->name = $name;
}
/**
* @return string
*/
public function getName(): string
{
return $this->name;
}
}
Now using the example:
<?php
// Let's create a new pet Instance.
$pet = new Pet("Rufus");
// Now here if we call
$pet->getName();
// This will return "Rufus" because when we created the pet instance the __constructor function was called by PHP and the value passed was assigned to the protected $name attribute.
// Now if we do
$pet->setName("Snowball");
// And call again
$pet->getName();
// This will return "Snowball" because we are programatically asking PHP to set the $name attribute to "Snowball".
Brandyn Lordi
17,778 PointsBrandyn Lordi
17,778 PointsThis makes a little bit more sense.
Does it have to be
__construct()
like :Or can i use this instead..?
Victor Avelar
3,393 PointsVictor Avelar
3,393 PointsI guess you will find out when you dig a little bit deeper in PHP that the "magic" methods (as it is called by the PHP community) start with __.
If you don't use the 'construct' magic method, you will need to call the function programmatically. Spoiler alert, you will also find stuff like 'destruct()',' call()', and 'invoke()'.
Hope this helps :)