Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial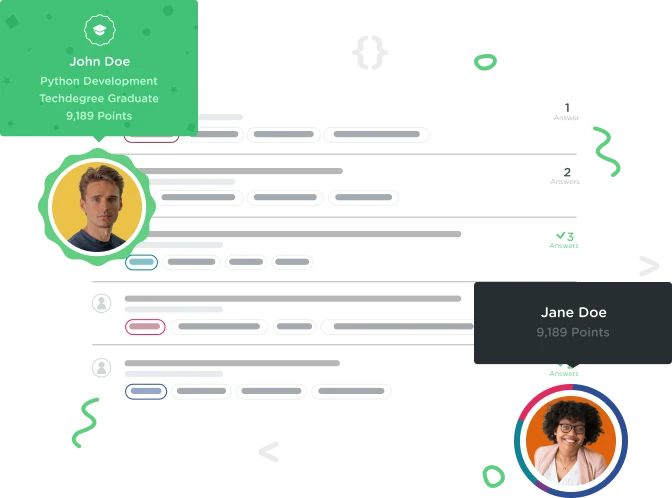

Ted Hayali
5,403 Pointscontinue problem
def loopy(items): # Code goes here i = 0 for item in items: if items[0] == 'a': continue else: print(item)
loopy(items)
def loopy(items):
# Code goes here
1 Answer

andren
28,558 PointsThis task does not require you to use continue (which skips an item), but break (which stops the loop), that is not actually the main problem with your code though, there are three big problems I can see right away:
You don't quite understand how for in loops work.
You are (trying to) compare all of the items to the letter "a", which is not what the task is asking you to do.
You create an index variable to access the items but you don't actually use it, (you hardcoded 0 as the index) and you don't increase the index within the loop either.
In a for in loop each item in a list is assigned to a variable inside of the loop (in your code you named the variable item, and the list is items) the first time though the loop the first object in the items list will be assigned to item, the second time though the loop the second object will be assigned to item, and so on.
Here is an illustration of how that works:
nameList = ["Tom", "Andy", "Peter", "Mona", "Lisa", "Elizabeth"]
for name in nameList:
print("Hello there " + name)
'''
The above code will generate this:
Hello there Tom
Hello there Andy
Hello there Peter
Hello there Mona
Hello there Lisa
Hello there Elizabeth
'''
So as you can see each name in the nameList was passed in to the code block and executed, once the loop had gone through all of the items it exits automatically. This means that you don't actually need to create or update an index variable manually.
The challenge request that when an item contains the string "STOP" that you should end the loop entirely, as I said above this require you to use the keyword break, not the keywoard continue, it also requires you to compare items to the string "STOP" not to the string "a" which you are doing in the above code.
The solution looks like this:
def loopy(items):
for item in items:
if item == "STOP":
break
else:
print(item)
It should also be noted that the challenge only asks you to complete the function, not to call it as well, doing that will actually cause the challenge to fail, even if the function's code is correct.
Ted Hayali
5,403 PointsTed Hayali
5,403 Pointsbut the question states you must use 'continue' statement and must be of index 0
andren
28,558 Pointsandren
28,558 PointsIn that case you must have linked to the wrong challenge, because the challenge you are currently linking and embedding in your question states this:
"I need you to help me finish my loopy function. Inside of the function, I need a for loop that prints each thing in items. But, if the current thing is the string "STOP", break the loop."
Which states neither of those things.
andren
28,558 Pointsandren
28,558 PointsI decided to look through some of the other challenges and found the one you meant to link to, the solution to that challenge is pretty similar:
Instead of checking if the item itself contains a string we are checking if the first letter of the item equals "a", if it does we continue.