Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial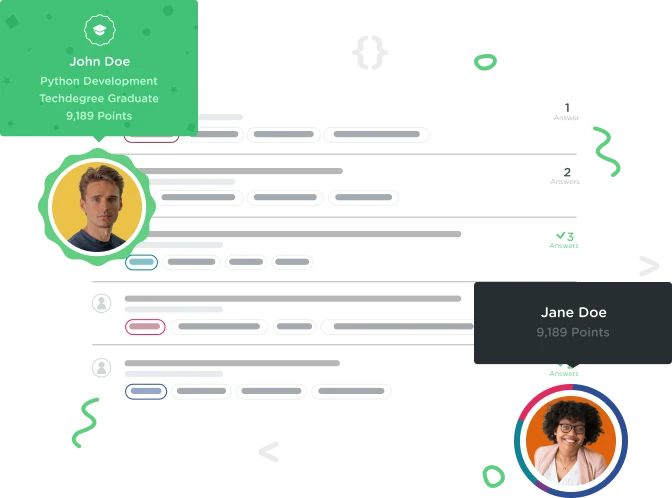
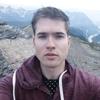
Gian Lazzarini
3,263 PointsConvert activities to fragments while using AppCompatActivity for Materiel Design support
I have some working android code that uses the AppCompatActivity to support floating TextEdit Labels, though, now I would like to convert these activities into fragments so I can add support for the Navigation Drawer.
I've been trying to figure this out for days, and it seems like it should be simple but has become complicated by using the android.support.v7.app.AppCompatActivity.
How do I turn my code into fragments so I can implement a Navigation drawer and other more advanced UI controls?
I have two "activity".java files and one layout.xml for each activity.
The Activity.java files are:
MainActivity.java
NewLogActivity.java
The layout.xml are:
main.xml
newlog.xml
The NewLogActivity.java and newlog.xml contain the working floating TextEdit Labels.
MainActivity.java
package com.lazztech.thoughtlog;
import android.app.*;
import android.content.*;
import android.os.*;
import android.support.v7.app.AppCompatActivity;
import android.view.*;
import android.widget.*;
public class MainActivity extends AppCompatActivity
{
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
public void onNewLogButtonClick(View view)
{
Intent intent = new Intent(this, NewLogActivity.class);
startActivity(intent);
}
@Override
public boolean onCreateOptionsMenu(Menu menu)
{
// Inflate main_menu.xml
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.main_menu, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item)
{
switch (item.getItemId())
{
case R.id.mainMenuAbout:
Toast.makeText(this, "This is my app!!!", Toast.LENGTH_SHORT).show();
return true;
case R.id.mainMenuExit:
finish();
return true;
}
return super.onOptionsItemSelected(item);
}
}
NewLogActivity.java
package com.lazztech.thoughtlog;
import android.app.*;
import android.os.*;
import android.view.*;
import android.widget.*;
import java.io.*;
import java.util.ArrayList;
import java.util.List;
import android.support.design.widget.TextInputLayout;
import android.support.v7.app.AppCompatActivity;
import android.content.*;
import android.app.Activity;
import android.os.Bundle;
import android.view.View.OnClickListener;
import android.widget.SearchView.*;
public class NewLogActivity extends AppCompatActivity
{
// GUI controls
EditText txtData;
EditText situation;
EditText thoughts;
EditText emotions;
EditText behavior;
CheckBox fortunetelling;
CheckBox mindreading;
CheckBox labeling;
CheckBox filtering;
CheckBox overestimating;
CheckBox catastrophizing;
CheckBox overgeneralizing;
List<String> distortions = new ArrayList<String>();
String fortuneTellingString = "Fortune-Telling";
String mindReadingString = "Mind-Reading";
String labelingString = "Labeling";
String filteringString = "Filtering";
String overestimatingString = "Overestimating";
String catastrophizingString = "Catastrophizing";
String overgeneralizingString = "Overgeneralizing";
EditText altbehavior;
EditText altthoughts;
Button btnWriteSDFile;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
//Set NewLogActivity.xml as user interface layout
setContentView(R.layout.newlog);
final TextInputLayout nameWrapper = (TextInputLayout) findViewById(R.id.nameWrapper);
nameWrapper.setHint("Name:");
// bind GUI elements with local controls
txtData = (EditText) findViewById(R.id.txtData);
situation = (EditText) findViewById(R.id.situation);
thoughts = (EditText) findViewById(R.id.thoughts);
emotions = (EditText) findViewById(R.id.emotions);
behavior = (EditText) findViewById(R.id.behavior);
fortunetelling = (CheckBox) findViewById(R.id.fortunetellingCheckBox1);
mindreading = (CheckBox) findViewById(R.id.mindreadingCheckBox2);
labeling = (CheckBox) findViewById(R.id.labelingCheckBox3);
filtering = (CheckBox) findViewById(R.id.filteringCheckBox4);
overestimating = (CheckBox) findViewById(R.id.overestimatingCheckBox5);
catastrophizing = (CheckBox) findViewById(R.id.catastrophizingCheckBox6);
overgeneralizing = (CheckBox) findViewById(R.id.overgeneralizingCheckBox7);
altbehavior = (EditText) findViewById(R.id.altbehaviors);
altthoughts = (EditText) findViewById(R.id.altthoughts);
btnWriteSDFile = (Button) findViewById(R.id.btnWriteSDFile);
}
//Checkbox onclick actions
public void onCheckboxClicked(View view)
{
// Is the view now checked?
boolean checked = ((CheckBox) view).isChecked();
// Check which checkbox was clicked
switch(view.getId()) {
case R.id.fortunetellingCheckBox1:
if (checked)
distortions.add(fortuneTellingString);
else
distortions.remove(fortuneTellingString);
break;
case R.id.mindreadingCheckBox2:
if (checked)
distortions.add(mindReadingString);
else
distortions.remove(mindReadingString);
break;
case R.id.labelingCheckBox3:
if (checked)
distortions.add(labelingString);
else
distortions.remove(labelingString);
break;
case R.id.filteringCheckBox4:
if (checked)
distortions.add(filteringString);
else
distortions.remove(filteringString);
break;
case R.id.overestimatingCheckBox5:
if (checked)
distortions.add(overestimatingString);
else
distortions.remove(overestimatingString);
break;
case R.id.catastrophizingCheckBox6:
if (checked)
distortions.add(catastrophizingString);
else
distortions.remove(catastrophizingString);
break;
case R.id.overgeneralizingCheckBox7:
if (checked)
distortions.add(overgeneralizingString);
else
distortions.remove(overestimatingString);
break;
}
btnWriteSDFile.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
// write on SD card file data in the text box
try {
File directory = Environment.getExternalStorageDirectory();
File myFile = new File(directory, "mythoughtlog.txt");
// Check if the file already exists so you don't keep creating
if(!myFile.exists()) {
//Log.i(TAG, "Creating the file as it doesn't exist already");
myFile.createNewFile();
}
// Open the FileoutputStream
FileOutputStream fOut = new FileOutputStream(myFile, true);
// Open the printStream to allow for Strings to be written
PrintStream printStream = new PrintStream(fOut);
// Using a stringBuffer to append all the values to
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append("Name: " + txtData.getText());
stringBuffer.append('\n');
stringBuffer.append("Situation: " + situation.getText());
stringBuffer.append('\n');
stringBuffer.append("Thoughts: " + thoughts.getText());
stringBuffer.append('\n');
stringBuffer.append("Emotions: " + emotions.getText());
stringBuffer.append('\n');
stringBuffer.append("Behavior: " + behavior.getText());
stringBuffer.append('\n');
stringBuffer.append("Distortions: ");
stringBuffer.append(distortions);
stringBuffer.append('\n');
stringBuffer.append("Alt Behavior: " + altbehavior.getText());
stringBuffer.append('\n');
stringBuffer.append("Alt Thoughts: " + altthoughts.getText());
stringBuffer.append('\n');
stringBuffer.append('\n');
// Print the stringBuffer to the file
printStream.print(stringBuffer.toString());
// Close everything out
printStream.close();
fOut.close();
txtData.setText("Name: "); //ClearScreen
situation.setText("Situation: ");
thoughts.setText("Thoughts: ");
emotions.setText("Emotions: ");
behavior.setText("Behavior: ");
altbehavior.setText("Alternative Behavior: ");
altthoughts.setText("Alternative Thoughts: ");
Toast.makeText(getBaseContext(),
"Saved to 'mythoughtlog.txt'",
Toast.LENGTH_SHORT).show();
} catch (Exception e) {
e.printStackTrace();
Toast.makeText(getBaseContext(), e.getMessage(),
Toast.LENGTH_SHORT).show();
}
}//Save onClick
});// btnWriteSDFile
}
}// AndSDcard
And my main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:gravity="top|center"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/title"
android:textStyle="normal"
android:gravity="center"
android:layout_marginTop="10dp"
android:textSize="20sp"
android:textColor="#000000" />
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:gravity="bottom|center">
<Button
android:layout_height="80dp"
android:text="New Log"
android:layout_width="match_parent"
android:layout_weight="1.0"
android:textSize="25sp"
android:onClick="onNewLogButtonClick"/>
</LinearLayout>
</LinearLayout>
newlog.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical">
<ScrollView
android:layout_width="fill_parent"
android:layout_height="500dp">
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<android.support.design.widget.TextInputLayout
android:id="@+id/nameWrapper"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/Theme.AppCompat.Light">
<EditText
android:layout_height="wrap_content"
android:inputType="textPersonName"
android:ems="10"
android:layout_width="match_parent"
android:hint="Name:"
android:id="@+id/txtData" />
</android.support.design.widget.TextInputLayout>
<android.support.design.widget.TextInputLayout
android:id="@+id/situationWrapper"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/Theme.AppCompat.Light">
<EditText
android:layout_height="wrap_content"
android:inputType="textMultiLine"
android:ems="10"
android:layout_width="match_parent"
android:hint="Situation:"
android:id="@+id/situation" />
</android.support.design.widget.TextInputLayout>
<android.support.design.widget.TextInputLayout
android:id="@+id/thoughtsWrapper"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/Theme.AppCompat.Light">
<EditText
android:layout_height="wrap_content"
android:inputType="textMultiLine"
android:ems="10"
android:layout_width="match_parent"
android:hint="Thoughts:"
android:id="@+id/thoughts" />
</android.support.design.widget.TextInputLayout>
<android.support.design.widget.TextInputLayout
android:id="@+id/emotionsWrapper"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/Theme.AppCompat.Light">
<EditText
android:layout_height="wrap_content"
android:ems="10"
android:layout_width="match_parent"
android:hint="Emotions:"
android:id="@+id/emotions" />
</android.support.design.widget.TextInputLayout>
<android.support.design.widget.TextInputLayout
android:id="@+id/behaviorWrapper"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/Theme.AppCompat.Light">
<EditText
android:layout_height="wrap_content"
android:ems="10"
android:layout_width="match_parent"
android:hint="Behavior:"
android:id="@+id/behavior" />
</android.support.design.widget.TextInputLayout>
<CheckBox
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:text="Fortune-telling: This occurs when we predict that things will turn out badly. However, we cannot predict the future because we don't have a magic ball!"
android:id="@+id/fortunetellingCheckBox1"
android:onClick="onCheckboxClicked"/>
<CheckBox
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:text="Mind-reading: This happens when we believe that we know what others are thinking and we assume that they are thinking the worst of us. However, we can't mind-read so we don't know what others are thinking!"
android:id="@+id/mindreadingCheckBox2"
android:onClick="onCheckboxClicked"/>
<CheckBox
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:text="Labeling: Sometimes we talk to ourselve in a mean way and we use a single negative word to describe ourselves. However, this kind of thinking is unfair and we are too complex to be summed up in a single word!"
android:id="@+id/labelingCheckBox3"
android:onClick="onCheckboxClicked"/>
<CheckBox
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:text="Filtering: This happens when we take note of all the bad things that happen, but ignore any good things."
android:id="@+id/filteringCheckBox4"
android:onClick="onCheckboxClicked"/>
<CheckBox
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:text="Overestimating: This happens when we believe that something that is unlikely to occur is actually about to happen."
android:id="@+id/overestimatingCheckBox5"
android:onClick="onCheckboxClicked"/>
<CheckBox
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:text="Catastrophizing: This is when we imagine the worst pssible thing is about to happen and we will be unable to cope with it."
android:id="@+id/catastrophizingCheckBox6"
android:onClick="onCheckboxClicked"/>
<CheckBox
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:text="Overgeneralizing: This is when we use words like always or never to describe situations or events. This is a problematic way of thinking because it does not take all situations or events into account."
android:id="@+id/overgeneralizingCheckBox7"
android:onClick="onCheckboxClicked"/>
<android.support.design.widget.TextInputLayout
android:id="@+id/altbehaviorsWrapper"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/Theme.AppCompat.Light">
<EditText
android:layout_height="wrap_content"
android:ems="10"
android:layout_width="match_parent"
android:hint="Alternative Behaviors:"
android:id="@+id/altbehaviors" />
</android.support.design.widget.TextInputLayout>
<android.support.design.widget.TextInputLayout
android:id="@+id/altthoughtsWrapper"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/Theme.AppCompat.Light">
<EditText
android:layout_height="wrap_content"
android:ems="10"
android:layout_width="match_parent"
android:hint="Alternative Thoughts:"
android:id="@+id/altthoughts" />
</android.support.design.widget.TextInputLayout>
</LinearLayout>
</ScrollView>
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:gravity="bottom|center">
<Button
android:layout_height="80dp"
android:text="Save"
android:layout_width="match_parent"
android:layout_weight="1.0"
android:textSize="25sp"
android:id="@+id/btnWriteSDFile"/>
</LinearLayout>
</LinearLayout>
1 Answer

Ozhan Saat
Courses Plus Student 4,002 PointsYou don't need fragments to be able to implement Nav drawer.
To convert to fragment you first want to extend Fragment. then you'll have to make some changes in the code.
You need onCreateView(LayoutInflater, ViewGroup, Bundle) instead of onCreate.
findViewById() becomes getView().findViewById()
There are a few other things but you'll work those out as you go.