Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial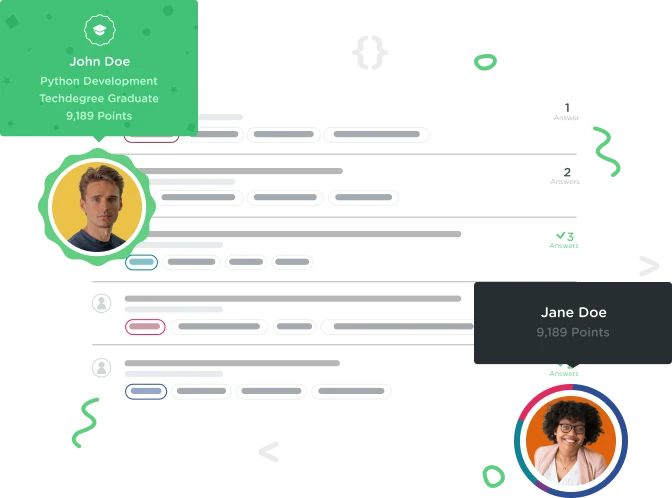
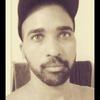
kabirdas
1,976 PointsConverting argument to integer, squared - help
I'm really not sure what direction to go in with this one. Please provide some assistance.
Here is the challenge:
This challenge is similar to an earlier one. Remember, though, I want you to practice! You'll probably want to use try and except on this one. You might have to not use the else block, though. Write a function named squared that takes a single argument. If the argument can be converted into an integer, convert it and return the square of the number (num ** 2 or num * num). If the argument cannot be turned into an integer (maybe it's a string of non-numbers?), return the argument multiplied by its length. Look in the file for examples.
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(apple):
try:
a = int(apple)
except ValueError:
return None
else:
return(a ** 2)
6 Answers
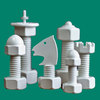
Steven Parker
230,995 PointsYou're halfway there.
It looks like you've got the case of returning a squared number covered. But if the input is not a number, the challenge says to "return the argument multiplied by its length" — yet your code returns None.
Fix that up and you should pass the challenge.
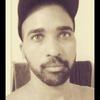
kabirdas
1,976 PointsAh, it's the parentheses! Thank you, Alex!
Ok, Joranin let's see if I'm explaining this right. The '=' is an assignment operator and '==' is an equality operator. I hope that helps! And if not I'm sure someone can elaborate. I'm quite new, so I don't feel too comfortable explaining things just yet!

someguy1234
3,450 PointsThank you, Kabir Das! It's fine with the answer. English is not my native, so it takes longer than usual to learn to code. I'm new too! Don't be discourage to help people. Thank you! Let's be a Python Master together! :D
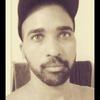
kabirdas
1,976 PointsYes! Let's!
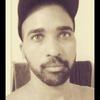
kabirdas
1,976 PointsThank you. I've changed it to:
return(apple * 5)
and now I get the error message, "'squared' didn't return the right answer."
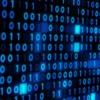
Alexander Davison
65,469 PointsYou need to multiply apple
by the length of the string apple
is containing.
Hint: To get the length of a string, use the len
function
>>> len("apple")
5
>>> len("BANANAZ!!") # Python returns 9 because it is also including the exclamation points
9
>>> len("hello world") # Remember the space counts as a character
11
~Alex
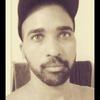
kabirdas
1,976 PointsI tried that before! Let me try it again. maybe I typed it wrong.
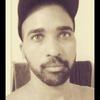
kabirdas
1,976 Pointsit still isn't coming out as correct....
def squared(apple):
try:
a = int(apple)
except ValueError:
return(apple * len("apple"))
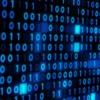
Alexander Davison
65,469 PointsDon't use quotes around "apples"! It will make Python think you want to get the length of the string "apple" itself.
Try this:
return apple * len(apple)

someguy1234
3,450 PointsI got return answer error too, then I figure it out I did wrong because I use if num == int(num) but when I use only one =. the quiz is passed.
int num == int(num) #not working
Can someone please explain why = is work when == don't.
def squared(num):
try:
if num = int(num)
return num**2
except ValueError:
return num * len(num)
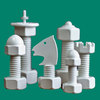
Steven Parker
230,995 PointsA single equal sign ("=
") is an assignment operator.
That causes the variable on the left to take on the value of the item or expression on the right.
Two of them ("==
") make a comparison operator. That's used for checking if two things have the same value. You'll often see these in if statements or in loops.
You probably don't want an assignment with an if, but you don't need the if anyway. If you just perform the assignment and it does not cause an exception, you know you have an integer and can then square and return it:
def squared(num):
try:
num = int(num)
return num ** 2
except ValueError:
return num * len(num)