Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial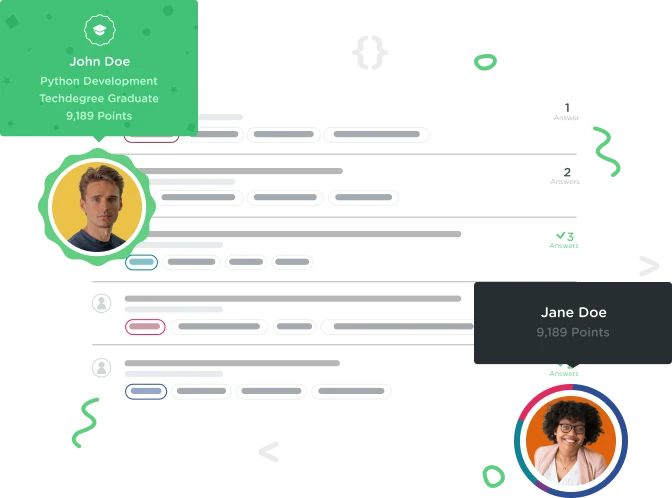

Aditya Shah
Courses Plus Student 776 PointsCourse's code seems lengthy. Thoughts on my solution?
Here's my code: https://w.trhou.se/vpriwow7cd
It's in script.js.
It accomplishes the goal of the previous challenge in a slightly different way. Namely, I don't understand why it's helpful to create a function for rgbcolor instead of leaving it as it is (just 1 line of code). However, since I'm a beginner, I might be missing an important principle. Is it simply that creating functions is "cleaner" (or DRYer) than having repetition in even a single line of code?
I realize that I could use a random int generating function and simplify my code even further here.
4 Answers
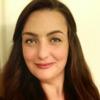
Jennifer Nordell
Treehouse TeacherHi there! The reason the rgbColor was moved out into its own function is so that it might be used in other places later on besides just that particular for
loop. You could potentially want to use it to set the color of a font or some other element that isn't being manipulated inside that particular loop using the rgbColor. It's just allowing for your code to grow.
That being said, you're doing something here that is overly complicating the code and has no effect. Math.random()
does not take any arguments.
function color(i) {
return Math.floor(Math.random(i) * 256 );
}
You pass in a 1, then a 2, and then a 3, but this is entirely unnecessary. This could be simplified to
function color() {
return Math.floor(Math.random() * 256 );
}
This would make the associated line to assign the rgbColor look like this:
rgbColor = 'rgb(' + color() + ',' + color() + ',' + color() + ')';
Hope this helps!

Dan Sally
Courses Plus Student 4,344 PointsI had a similar question, but my solution was a little different than Aditya's. Any comments on the code below?
function color(){
rgbcode = Math.floor(Math.random() * 256 );
return rgbcode
}
var html = '';
var rgbColor;
for(i=0; i<10; i += 1){
rgbColor = 'rgb(' + color() + ',' + color() + ',' + color() + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);

Christofer Chan
4,642 PointsHey Dan! Your code works fine. Instead of creating the variable "rgbCode" you can return the result directly to color() and get the same result.
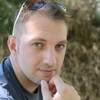
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 Pointsas christofer said you can return without a variable, so the function itself would actually return the color. your code:
function color(){
rgbcode = Math.floor(Math.random() * 256 );
return rgbcode;
}
function color(){
return Math.floor(Math.random() * 256 );
}
also careful on the camelCase just for best practice.
rgbcode
should be
rgbCode
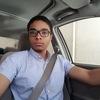
Luqman Shah
3,016 PointsThis part really confused me, I know we've already learned about functions, but we are already trying to understand the concept of loops, this was a bit too much information to swallow.
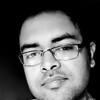
Arnab Banerjee
13,373 PointsHi all !! I was having a lot of fun with this challenge and came up with a way to solve it without using any functions
Have a look at the code!!