Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial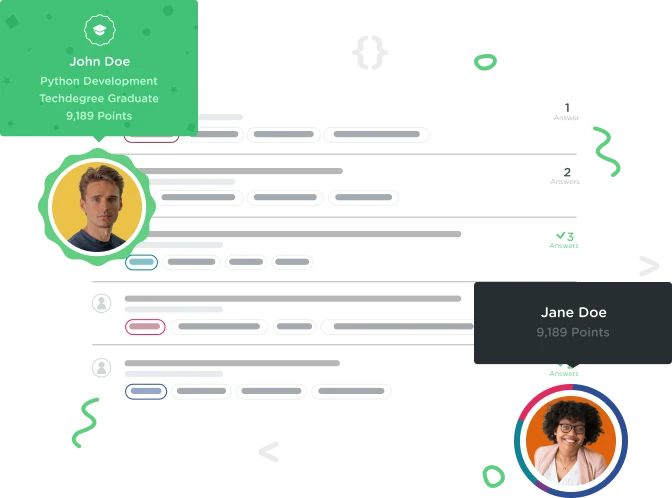
michaelabendroth
4,561 Pointscourses function in code challenge
The question asks us to write a function called courses that takes a dictionary of teachers and lists of the courses they teach and returns a list of all courses taught by all teachers. I figured I would need to loop through the keys in the dictionary, then loop through the items in each list. However, my answer is returning too many classes (when I tried it without the if statement to check for duplicates, it returned even more classes). Any help would be appreciated.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(a_dict):
teacher = ''
max_count = 0
for key in a_dict:
if len(a_dict[key]) > max_count:
max_count = len(a_dict[key])
teacher = key
else:
continue
return teacher
def num_teachers(a_dict):
count = 0
for key in a_dict:
count += 1
return count
def stats(a_dict):
a_list = []
for key in a_dict:
a_list.append([key, (len(a_dict[key]))])
return a_list
def courses(a_dict):
a_list = []
for key in a_dict:
for item in key:
if item not in a_list:
a_list.append(item)
else:
continue
return a_list
4 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi michaelabendroth,
In your outer for loop you're retrieving each key from the dict which is a teacher's name. You're then iterating over each letter of the teacher's name with your inner for loop which is leading you to incorrect results.
Instead, you should try to get the course list for that teacher with a_dict[key]
and add it onto your a_list
You can solve this without a nested for loop.

Gavin Ralston
28,770 PointsRemember that your goal here is to get the key and value pairs for each item in the dictionary.
Once you get the items from the dictionary, you can then unpack them into the variables as you'd expect, like so:
def most_classes(teachers):
top_teacher = ''
max_count = 0
for teacher, classes in teachers.items():
if len(classes) > max_count:
top_teacher = teacher
max_count = len(classes)
return top_teacher
If you just used for teacher, classes in teachers, you'd get a dict back, but not an iterable list of dict_items like you want to work with.

Jason Anello
Courses Plus Student 94,610 PointsHi Gavin,
I think the original code posted for most_classes
was correct and this question was about the last function courses
Also, in your version here, you're not updating the max_count variable so your top_teacher returned will always be the last teacher iterated over.

Gavin Ralston
28,770 PointsGood catch. I'll add the line back in. :) I was mainly focused on remembering that a dict object isn't a dict_items iterable, and wound up removing too much code.
Additionally, I wasn't sure if just posting the solution was an appropriate way to answer. I was hoping the inclusion of #items would trigger an a-ha moment. :)
michaelabendroth
4,561 PointsI appreciate you both having my back. I was stumped on that one.
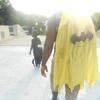
Prince Vonleh
Courses Plus Student 4,552 Pointsdef num_teachers(teach_dict): count_teachers = [] for teachers in teach_dict: count_teachers.append(teachers) return (len(count_teachers))
This worked for me!
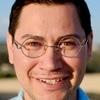
William Ruiz
10,027 PointsHere's what worked for me. If statement not necessary:
def courses(teach_dict):
my_list = []
for teachers in teach_dict:
for courses in teach_dict[teachers]:
my_list.append(courses)
return my_list
michaelabendroth
4,561 Pointsmichaelabendroth
4,561 PointsThank you! Appreciate the help