Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial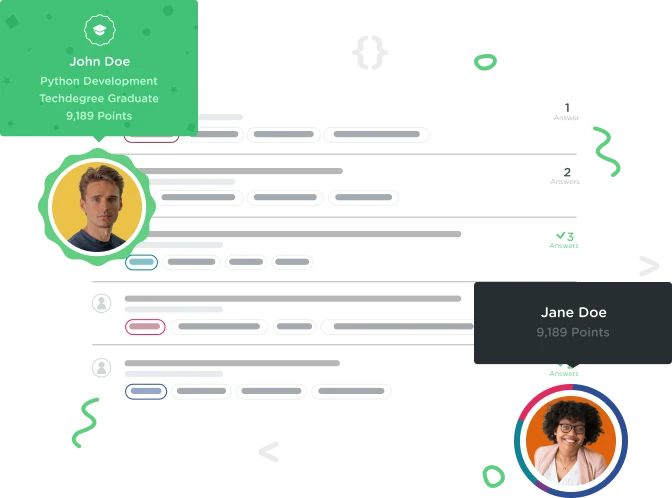
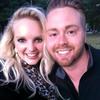
Jesse Fister
11,968 Pointscreate a do while loop
it is asking me to create a do while loop... this appears to be correct to me but it is saying my code is taking too long to run?
var secret = prompt("What is the secret password?"); var guess = prompt(secret); var correctGuess = false; var guessCount = 0;
do { guess = prompt("What is the secret password?"); guessCount += 1; if (parseInt(guess) === "sesame") { correctGuess = true; } } while ( ! correctGuess )
document.write("You know the secret password. Welcome.");
var secret = prompt("What is the secret password?");
var guess = prompt(secret);
var correctGuess = false;
var guessCount = 0;
do {
guess = prompt("What is the secret password?");
guessCount += 1;
if (parseInt(guess) === "sesame") {
correctGuess = true;
}
} while ( ! correctGuess )
document.write("You know the secret password. Welcome.");
//var guess = prompt(secret);
/*while (guess !== "sesame") {
prompt(secret);
secret = prompt();
}*/
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
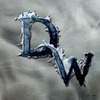
Hugo Paz
15,622 PointsHi Jesse,
You dont need auxiliary variables nor do you need an if. You can use the while to test the condition
var secret;
do {
secret = prompt("What is the secret password?");
} while ( secret !== 'sesame' );
document.write("You know the secret password. Welcome.");
Robert Mews
11,540 PointsRobert Mews
11,540 PointsHey Hugo,
I was trouble shooting the Javascript do while loop challenge when I saw this. Don't we need a conditional in the do part? What happens if the prompt is answered correctly on first attempt?
Hugo Paz
15,622 PointsHugo Paz
15,622 PointsHi Robert,
The advantage of the do...while loop is that it always runs at least one time.
So if we change the code above to this:
Even though the variable secret already has the correct value, the do..while loop will still run. And the prompt value will override the current one.
Regarding the prompt being answered right the first time. The prompt is inside the loop, so for the prompt to be called, the loop needs to run at least once (assuming you input the correct value the first time). The while part checks the condition. If its true the loop terminates.
Zherui Li
993 PointsZherui Li
993 Pointsthank you Hugo, your explanation very detail and helpful!
Stephen Acheson
7,412 PointsStephen Acheson
7,412 PointsThanks for this. Was a little stuck on this and knew it was something simple I was missing.