Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial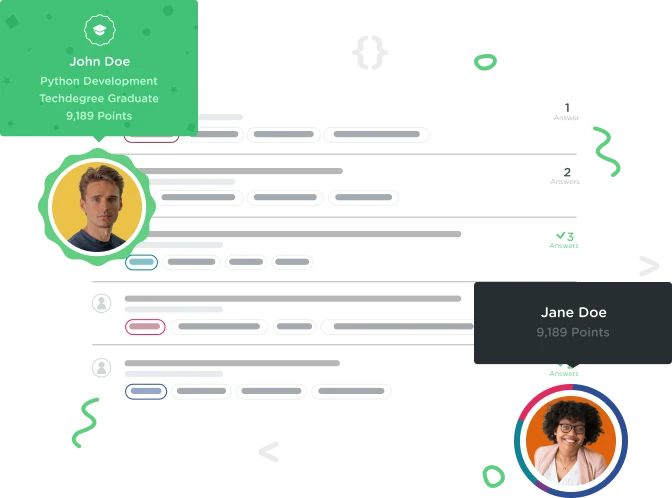
Nyasha Chawanda
7,946 Pointscreate a function named most_classes that takes a dictionary of teachers and returns the teacher with most classes
my code is picking any of the teachers instead of picking the teacher with most classes. Can anyone help me in identifying the error on my code.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes (d):
max_count=0
busy_teacher=''
for x,y in d.items():
if len(y)>max_count:
max_count=len(y)
busy_teacher=x
return busy_teacher
Nyasha Chawanda
7,946 Pointsthanks for identifying the error. it worked out!!
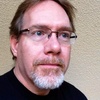
Chris Freeman
Treehouse Moderator 68,441 PointsSean McKeown: Please make your comment an answer so Nyasha Chawanda can marked it Best Answer (and to remove it from the unanswered list!). Thanks
4 Answers

Sean McKeown
23,267 PointsI think your busy_teacher=x line needs to be inside the if statement. Right now busy_teacher will always be the last one that the for loop processes.

David Diehr
16,457 PointsThe code provided by Abenezer does work, but it includes some concepts not yet taught at this point in the track so I thought that I would provide my answer (which was aided by looking at Abenezer's code).
def most_classes(teacher_dict):
big_num = 0
big_teacher = ''
for teacher in teacher_dict:
class_num = len(teacher_dict[teacher])
if (class_num > big_num):
big_num = class_num
big_teacher = teacher
return big_teacher
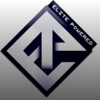
Abenezer Mamo
5,821 Pointsdef most_classes(my_dict):
teachers_list = {}
for teacher in my_dict:
teachers_list[teacher] = len(my_dict[teacher])
return max(teachers_list, key=teachers_list.get)

aleksandrsigaev
629 PointsFor what you use key=teachers_list.get ?
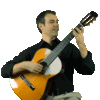
Stephen Hanlon
11,845 PointsHere's a solution starting off with list comprehension. Not sure if doing two loops to get the answer is ideal though.
def most_courses(treehouse):
most = max([len(item[1]) for item in treehouse.items()])
for teacher in treehouse.items():
if len(teacher[1]) == most:
return(teacher[0])
Just change built-in max() to min() to find out who taught the least amount of classes.
Sean McKeown
23,267 PointsSean McKeown
23,267 PointsI think your busy_teacher=x line needs to be inside the if statement. Right now busy_teacher will always be the last one that the for loop processes.