Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial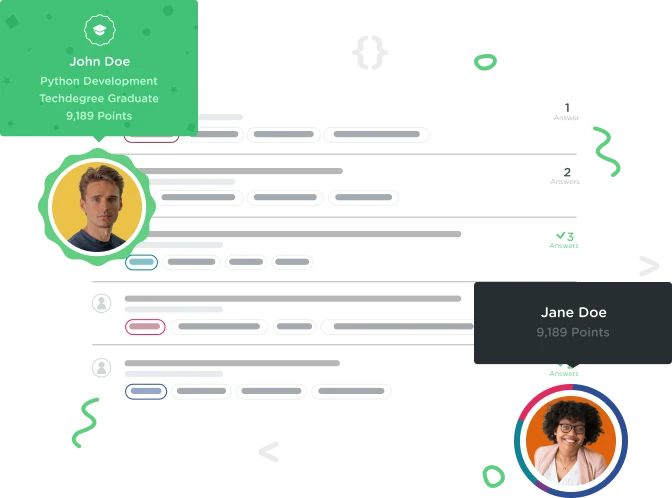

Kevin Her
7,220 PointsCreate a function named most_classes that takes a dictionary of teachers. Each key is a teacher's name and their value i
my_dict = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(my_dict):
most_class = "" #holds the name of teacher with the most class
max_count = 0 #max counter for classes
# For loop for most classes
for key in my_dict:
if len(my_dict[key]) > max_count:
max_count = len(my_dict[key])
most_class = key
return most_class
Create a function named most_classes that takes a dictionary of teachers. Each key is a teacher's name and their value is a list of classes they've taught. most_classes should return the teacher with the most classes.
I have my code attached and it works. I don't understand where in the code does it actually decide which key has the most value and returns the amount with the most value.
Secondly how come I have to create the variable most_classes = key and just can't return key
1 Answer

Zachary Kent
7,133 PointsHi Kevin,
for key in my_dict: # loops through each key in my_dict
if len(my_dict[key]) > max_count: # uses key to find its value, measures length & compares to max_count
max_count = len(my_dict[key]) # if the length was greater than max_count, update the max_count value
most_class = key # set the most_class variable to the teacher name(key) with the most classes
return most_class # returns most_class --> teacher's name or key in my_dict
The code decides which key has the highest value when it compares len(my_dict[key])
to max_count
. By writing
my_dict[key]
you get the list of classes each teacher has as a value and then you call len()
to get the number of items in the list. This number is then compared to the max_count
. If it is greater than max_count
you change to max_count
to that number and then keep track of the teacher with the most classes by assigning a thier name to the most_class
variable.
You need to keep track of the current teacher with the most classes and that is why you return the most_class variable at the end of the loop. You're basically are returning a key but just the one that you identified as having the most classes in the for loop.
You're using the two variables: most_class and max_count to keep track of the information you're looking for while running your for loop. By updating these variables when you find a teacher(key) with more classes(len(value)) you can then return the teacher with the most classes when the for loop is finished.
Hope that helps
Zachary Kent
7,133 PointsZachary Kent
7,133 PointsSorry about the first paragraph. I had it formated way differently so you could read it but when I hit submit it changed all the spacing and squashed the comments and code into one paragraph.
Haider Ali
Python Development Techdegree Graduate 24,728 PointsHaider Ali
Python Development Techdegree Graduate 24,728 PointsHi Zachary, I have added the correct formatting to your answer, hope that helps.