Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial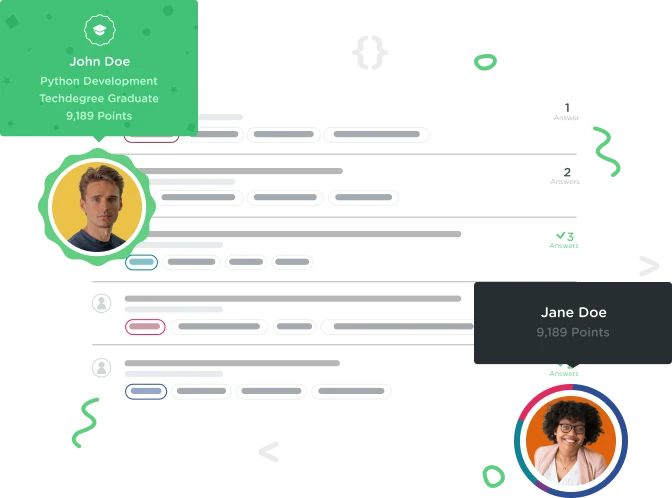

Ryan Lail
4,386 PointsCreate a function names stats that takes the dictionary and return a list...
For example, one item in the list would be ['Dave Mcfarland', 1]
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(dict_of_teachers):
max_count = 0
max_count_teacher = ''
for key, value in dict_of_teachers.items():
if len(value) > max_count:
max_count = len(value)
max_count_teacher = key
return max_count_teacher
def num_teachers(dict_of_teachers):
count = 0
for teacher in dict_of_teachers:
count += 1
return count
def stats(dict_of_teachers):
for key, value in dict_of_teachers.item():
output = "['{} , {}']".format(dict_of_teachers, len(dict_of_teachers[key]))
return output
1 Answer
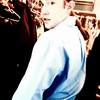
Brandon Wall
5,512 PointsOk let me take a shot at walking you through this, this one was always really hard for me too, even after finishing the entire python track i have a bit of trouble with it. Ironically i feel the challenges get easier and easier after python collections.
def most_classes(adict):
max_count = 0
max_teacher = ''
for key in adict.keys():
if len(adict[key]) > max_count:
max_count = len(adict[key])
max_teacher = key
return max_teacher
# Here we iterate through each key using the .keys() method of the dictionary class, then we call upon the length of the
#list held by each key with len(adict[key]). If it is greater than what is currently assigned to max count, that length gets
#assigned, they key holding that data gets assigned to max_teacher and our loop moves on to the next item, finally
#returning our max_teacher that should correspond to the list with the greatest length.
def num_teachers(adict):
total = 0
for key in adict.keys():
total += 1
return total
#Here we create another count variable,
#and iterate through each key again using the .keys() method. We know each key
#is a teacher, so for every key our for loop iterates through our
#total variable increases by one, finally we return the total
def stats(adict):
my_list = []
for key, value in adict.items():
another_list =[key, len(value)]
my_list.append(another_list)
return my_list
#Here we need to return a list of lists, i remember doing this differently when i first started out, but this is how i solved it
#this time. First we create a variable to hold a list, then we begin our for loop and use two variables with the .items()
#method. This method needs two variables to hold onto the data it will iterate through because it iterates through the
#key and its corresponding value at the same time. In our for loop we define another_list that holds a list that will have
#the current key our loop is on, as well as the value that is the length of the list. Remember that here the value variable is
#a list, because each key in the dictionary holds a list. Before our for loop continues on to fill that list again with different
#stuff in the next iteration of the loop we tell it my_list.append(another_list). Our for loop goes for as many iterations as
#there are items in our list. So through each iteration it builds the another_list variable, appends it to my_list and the
#continues on finally returning my_list which is now a list of lists.
def courses(adict):
all_classes = []
for value in adict.values():
for a_class in value:
all_classes.append(a_class)
return all_classes
#finally we need to return a list of just all the classes, so now we use the .values() method since we are not concerned
#with the teachers anymore which are the keys, we are only concerned with the classes which are held in the values
#which are lists. So here we need to use a nested for loop because we have an iterable, our dictionary, holding onto
#another type of iterable in its values which are lists of classes. So we are telling it to iterate over value, then telling
#another for loop to iterate over a_class in value. This effectively unpacks all of the lists held by each of the values, and
#on each iteration of our inner for loop we tell it to append each class to our variable all_classes which is an empty list.
#Finally returning all_classes which will give us one nice list instead of a list of lists. The End :D
Sorry about the wonky formatting of the comments, just highlight the text and move your mouse over as you drag to make it scroll over. Please read my comments, hopefully i don't just confuse you more, but I don't want to have just handed out lines of code without an explanation as well otherwise i'm not really doing you a service. Happy coding!