Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial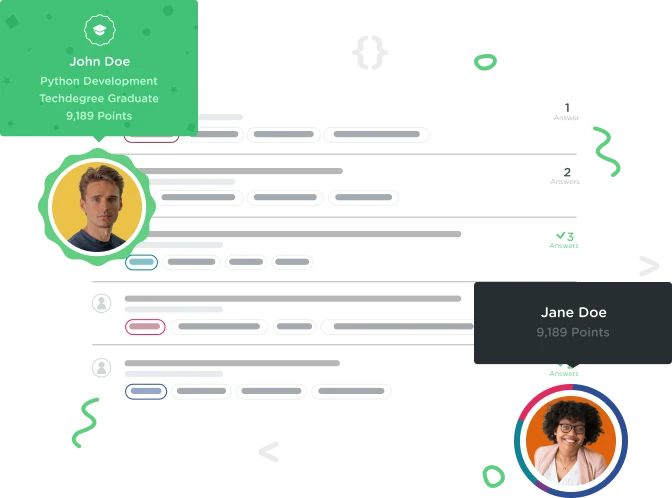
Hangyu Kang
7,027 PointsCreating a new array with search results, I'm able to display two Jodys
while(true){
var input = prompt("Search:");
var search = [];
if ( input === "" || input === "quit"){
break;
}
for ( var i = 0; i < students.length; i += 1){
if(input === students[i].name){
search.push(students[i]);
}
}
for ( var i = 0; i < students.length; i += 1){
printStudent(search[i]);
}
}

Craig Williams
2,883 PointsI used a similar method but realised you need to empty the search array after each time you call the function that prints it, otherwise multiple searches will continue to add more records to your search array, and each search will print out all of the array again as well as any additional records.
You can use an if statement to check the search array length instead of the second for loop, then call a function if there's items in the array and clear the array afterwards like so...
for ( var i = 0; i < students.length; i += 1){
if (input === students[i].name) {
search.push(students[i]);
}
}
if (search.length > 0) {
getStudentRecords();
search = []; /* Clears the array after the function runs, useful for multiple searches */
}
else {
print("<h2>No results found.</h2>");
}
Then the function you run in the if statement can loop through the search array and print the results, in this instance I called the function getStudentRecords.
function getStudentRecords() {
/* Create the variable you will print to the page and also display the number of matching records */
var record = "<h2>The search returned " + search.length + " record/s</h2>";
/* Create an ordered list to see each matching result more clearly */
record += "<ol>"
/* loop through the search array adding the results to the record variable */
for (var j = 0; j < search.length; j += 1) {
record += '<li><ul><li>Student: ' + search[j].name + '</li>';
record += '<li>Track: ' + search[j].track + '</li>';
record += '<li>Points: ' + search[j].points + '</li>';
record += '<li>Achievements: ' + search[j].achievements + '</li></ul></li>';
} /* loop ends */
record += "</ol>"; /* closes the opening <ol> tag */
print(record);
}
1 Answer
Hangyu Kang
7,027 PointsThank you! Great advice.
Andrei Fecioru
15,059 PointsAndrei Fecioru
15,059 PointsYou have the
i
var defined twice in the same lexical scope once for eachfor
loop.It's not an error but it is not required. It's not optimal either because you allocate the
i
variable twice (the second definition hides the first one). In fact, some IDEs (such as the IntelliJ WebStorm) would flag this as an warning. I would re-write this as follows:Also, in the section where you check the user input you also need to explicitly check for
null
because you use the strict equality operator and if the user clicks the Cancel button the return value from theprompt
function isnull
. Thenull
value will pass undetected through your input data validation phase.