Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial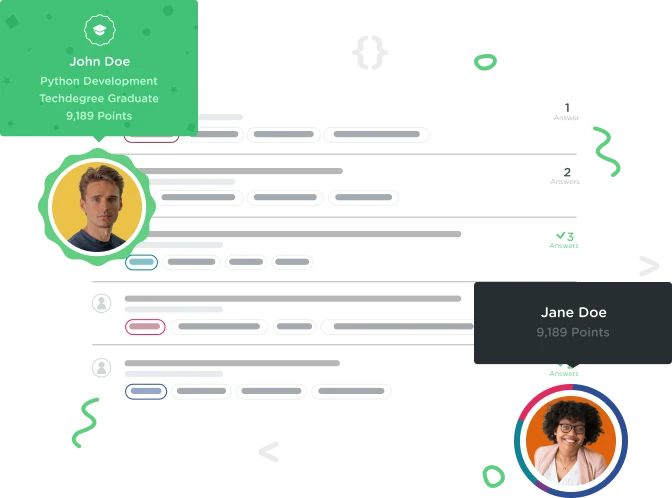
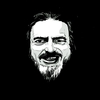
Kamal Nassouh
3,547 PointsCritique my code haha!
Please let me know what I did wrong and what you would have done differently.
//setting the correct variables that are needed to ask the questions " these will count down as per questions asked and the answers.
//console.log commands are in here for testing of scores and questions that are being asked..
var questions = 4;
var questionsLeft = ("questions remaining ");
var correct = 0;
console.log(questions,correct);
//first question *** if the question is correct. the *correct variable is updated to += 1
var question1 = prompt("what was the last year the bulldogs won the grand final? " + questionsLeft + questions);
if (question1 === "2004") {
correct += 1;
}
console.log(questions,correct);
questions = 3;
//second question *** if the question is correct. the *correct variable is updated to += 1
var question2 = prompt("what is the last name of the bulldog player Terry? " + questionsLeft + questions);
if (question2.toUpperCase() === "LAMB") {
correct += 1;
}
console.log(questions,correct);
var questions = 2;
//third question *** if the question is correct. the *correct variable is updated to += 1
var question3 = prompt("what is the name of the best half back? " + questionsLeft + questions );
if ( question3.toUpperCase() === "THURSTON") {
correct += 1;
}
console.log(questions,correct);
var questions = 1;
//fourth question *** if the question is correct. the *correct variable is updated to += 1
var question4 = prompt("complete the following sentence what is the name of the progamming code Ruby on **** ??? " + questionsLeft + questions );
if (question4.toUpperCase() === "RAILS") {
correct += 1;
}
console.log(questions,correct);
var questions = " last question ";
//last question
var question0 = prompt( " finish the name of the game Counter ****** ???" +questions );
if (question0.toUpperCase === "STRIKE") {
correct += 1;
}
//alert questionaire finished
alert("thanks for completeing my questions, click ok lets see how you went");
// if statements if user has < 1 then he is displayed one message, if nto displayed the else if statements.
if (correct < 1) {
alert("You really need to start doing somthing awesome you failed");
} else if ( correct >= 3) {
alert(" hey not bad at all you got 3 or more right!!!!! ")
}
4 Answers
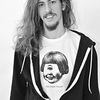
eck
43,038 PointsThanks!
So the questions
variable you are asking about does not actually ask the questions exactly, but stores the questions and their answers in a way that is easy to loop over.
forEach()
is a method of Array
("questions" is an array containing objects) that lets us loop over each object. Now we don't need to repeat the code for prompt()
and validating the user's answer, keeping track of correct answers, etc...
Ah yes, if you are declaring multiple variables in a group, you can use commas to avoid writing "var" more than once. This is a stylistic choice make because I write less and technically my file size will be slightly less (it will not really make any performance difference, I just like the idea of it :P)
var questions, questionsAsked, questionsCorrect;
// or
var questions,
questionsAsked,
questionsCorrect;
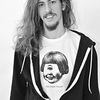
eck
43,038 PointsI went through your code and found some code style issues, spelling, and minor syntax mistakes which you can see a breakdown of below:
- The "questions" variable starts at 4 but there is actually 5 questions.
- When declaring the value for the "questionsLeft" variable you placed the string in unnecessary parentheses.
- Unnecessary spaces and sporadic spaces throughout the code. (You want to be consistent in how and where you use spaces)
- Inconsistent use of tabs and spaces to indent code.
- Closing curly braces for have not been correctly indented in most cases.
- Many comments are redundant.
- There are some spelling mistakes: "progamming", "questionaire", "completeing", "nto", "somthing", "bulldog" (Bulldogs)
- Grammar and punctuation could also be improved (I fixed some of this in the last example I show)
- I think it makes a bit more sense to reassign the value of the "questions" variable before the console.log is called to report the changes.
- You are re-declaring the "questions" variable with the var keyword multiple times. You do not need to include var if the variable already exists.
- The name of the "questionsLeft" variable is confusing. One would expect its value to be an integer of the remaining questions, not a string.
- You missed a semi-colon for the last alert at the end of the code.
- If the person answering gets 1 or 2 questions right nothing will be alerted at the end.
- It is considered a best practice by many to only declare variables at the top of a scope/block (I didn't make any changes related to this in the first code example)
var questions = 4;
var questionsLeft = ("questions remaining ");
var correct = 0;
console.log(questions,correct);
var question1 = prompt("what was the last year the bulldogs won the grand final? " + questionsLeft + questions);
if (question1 === "2004") {
correct += 1;
}
questions = 3;
console.log(questions,correct);
var question2 = prompt("what is the last name of the bulldog player Terry? " + questionsLeft + questions);
if (question2.toUpperCase() === "LAMB") {
correct += 1;
}
questions = 2;
console.log(questions,correct);
var question3 = prompt("what is the name of the best half back? " + questionsLeft + questions);
if (question3.toUpperCase() === "THURSTON") {
correct += 1;
}
questions = 1;
console.log(questions,correct);
var question4 = prompt("complete the following sentence what is the name of the programming code Ruby on **** ??? " + questionsLeft + questions);
if (question4.toUpperCase() === "RAILS") {
correct += 1;
}
questions = " last question";
console.log(questions,correct);
var question0 = prompt("finish the name of the game Counter ****** ???" + questions);
if (question0.toUpperCase === "STRIKE") {
correct += 1;
}
alert("thanks for completing my questions, click OK lets see how you went");
if (correct < 1) {
alert("You really need to start doing something awesome you failed");
} else if (correct >= 3) {
alert(" hey not bad at all you got 3 or more right!!!!!");
} else {
alert("Try again!");
}
There are also some more advanced tips I have for you.
To take this code to the next level you will want to make it DRY. Currently the way each question is asked is very similar, so there is the potential to really cut down the amount of code you have written.
Below I will show you a solution that is a bit more DRY, don't worry if you don't understand everything, there is probably some unfamiliar stuff going on. Please feel free to ask me any questions you have about either example!
var questionsAsked = 0,
questionsCorrect = 0,
questions = [
{
q: "What was the last year the Bulldogs won the grand final?",
a: "2004"
},
{
q: "What is the last name of the Bulldogs player Terry?",
a: "LAMB"
},
{
q: "What is the name of the best half back?",
a: "THURSTON"
},
{
q: "Complete the following sentence: what is the name of the programming code Ruby on **** ???",
a: "RAILS"
},
{
q: "Finish the name of the game Counter ****** ???",
a: "STRIKE"
}
];
questions.forEach(function(question){
var questionsLeft = questions.length - questionsAsked,
answer = prompt("Questions left: " + questionsLeft + "\n" + question.q).toUpperCase();
if (answer === question.a) {
questionsCorrect++;
}
questionsAsked++;
console.log(questionsLeft - 1, questionsCorrect);
});
alert("Thanks for completing my questions, click OK to see how you did.");
if (questionsCorrect < 1) {
alert("You really need to start doing something awesome you failed.");
} else if (questionsCorrect >= 3) {
alert("Hey not bad at all, you got 3 or more right!!!!!");
} else {
alert("Try again!");
}
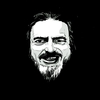
Kamal Nassouh
3,547 PointsHi Erik,
Thanks for the response
first off! BANGING Website you have dude whoah..!!!!!
I do understand some of the code however, I just wanted to ask you about the questions section .
is this part of the code asking the questions ?
questions = [
{
q: "What was the last year the Bulldogs won the grand final?",
a: "2004"
},
{
q: "What is the last name of the Bulldogs player Terry?",
a: "LAMB"
},
{
q: "What is the name of the best half back?",
a: "THURSTON"
},
{
q: "Complete the following sentence: what is the name of the programming code Ruby on **** ???",
a: "RAILS"
},
{
q: "Finish the name of the game Counter ****** ???",
a: "STRIKE"
}
and also, when declaring the VAR you only typed it for one of the variables, I didn't know that could be done, so your basically creating a variable ? :
var questionsAsked = 0, questionsCorrect = 0, <-------------------------- questions = <---------------------
Thanks for your help !
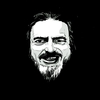
Kamal Nassouh
3,547 Pointsthanks bud appreciate the in depth responses!
eck
43,038 Pointseck
43,038 PointsI edited your post to format your code as JavaScript. Make sure when you use backticks to indicate a block of code that you also include js, html, css, or php etc depending on what language it is written in. You would write this directly behind the first 3 backticks (no spaces, I think)