Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial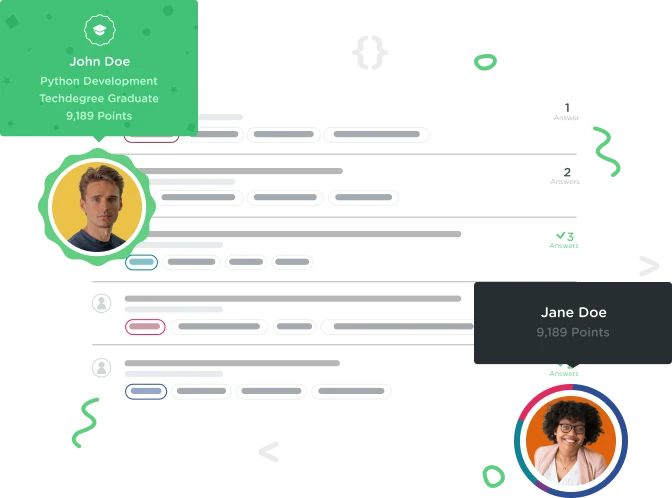

kevin cleary
4,690 Pointsdecrement start by 1
Make a while loop that runs until start is falsey. Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99. If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number. Finally, decrement start by 1.
I think I'm getting hung up on the decrement start by 1 part. I think the rest is close. Any suggestions would be greatly appreciated. Thank you.
import random
start = 5
def even_odd(num):
rand = random.randint(1, 99)
while rand = rand % 2:
print("{} is even".format(rand))
else:
print("{} is odd".format(rand))
start = start -= 1
return not num % 2
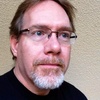
Chris Freeman
Treehouse Moderator 68,461 PointsAdded ```python markdown formatting.
4 Answers
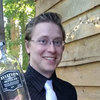
Evan Demaris
64,262 PointsHi kevin,
Your code isn't using the "start" variable at all. So start will be decremented each loop through, but your while loop doesn't look at that. Your code is also trying while rand = rand % 2
, which means that it's assigning rand % 2 to rand then checking rand, where it could just check rand % 2 without assignment. However, I wouldn't recommend using that while loop at all, as a rand % 2 while loop will stop as soon as it runs into an even number (0 is falsey). You can also just use start -= 1 towards the end of your code, instead of assigning start to start -= 1
.
The while loop is supposed to be outside the function, and you can use while start
for it because it will become falsey once start is decremented to 0.
I've included a passing version of the code below, let me know if you have any questions about how or why it works!
import random
start = 5
def even_odd(num):
return not num % 2
while start:
randint = random.randint(1, 99)
if even_odd(randint):
print("{} is even".format(randint))
else:
print("{} is odd".format(randint))
start -= 1
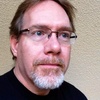
Chris Freeman
Treehouse Moderator 68,461 PointsHere is a step by step code for Task 3:
# Task 3 of 3
# Alright, last step but it's a big one.
# Make a while loop that runs until start is falsey. (start is truthy)
while start:
# Inside the loop, use random.randint(1, 99) to get a
# random number between 1 and 99.
number = random.randint(1, 99)
# If that random number is even (use even_odd to find out)...
if even_odd(number):
# print "{} is even", putting the random number in the hole.
print("{} is even".format(number))
# Otherwise,...
else:
# print "{} is odd", again using the random number.
print("{} is odd".format(number))
# Finally, decrement start by 1.
start -= 1
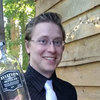
Evan Demaris
64,262 PointsI like how you used while start
instead of the while start > 0 that I used, it's more efficient - I updated my answer to reflect that!
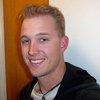
Richard Boag
5,547 PointsLove the step by step explanation! Some of the procedural things get lost on me, so seeing an explanation of what each line accomplishes was a great help!

Kevin Veerasammy
7,786 PointsLOVE THIS! id love to see more step by step explanations! it helps me learn alot better!
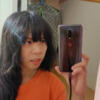
Name:GoogleSearch orJonathan Sum
5,039 Pointswhat does the Start -=1 mean ? how does the function even_odd() work?
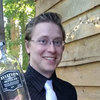
Evan Demaris
64,262 PointsHi Jonathan,
start -=1
decrements the value of start
each time it is run, which is important for start
to reach 0 and end the while
loop.
even_odd()
returns the opposite of modulo 2 of the number it's given. As an example, 3 modulo 2 would return 1, which is truthy, but 4 modulo 2 would return 0, which is falsey. Because even numbers would return false, and the intent of the even_odd()
function is to return true for even and false for odd, not
is used to reverse the true/false output. So even_odd()
will return true for even, and false for odd.
Hope that helps!

ryan smith
687 PointsChris Freeman Why can't it be
while start True:
instead of
while start:
Also why is number not num in the following code
if even_odd(number):
because the function was
def even_odd(num):
Does it not have to be the argument exactly?
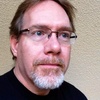
Chris Freeman
Treehouse Moderator 68,461 PointsGood question Ryan!
First, consider that a while
command needs a conditional statement that evaluates to True or False. In addition to explicitly True and false values, objects that have a "truthy" or "false-like" value are also acceptable.
The variable start
is an int so when it's used in a conditional statement it is "true" unless it has a zero value, then its "false".
# this is a syntax error without an operator
while start True:
# this fails because an integer may be truthy, but never actually True
while 5 == True
>>> 5 == True
False
>>> 0 == True
False
# this works when used in a truthy context
while start:
Also why is number not num in the following code
In the first code, number
is in the context of the while
, when the code is executed number
is used as the argument when calling the function even_odd
.
if even_odd(number):
The function even_odd
uses num
as a parameter with which to accept an argument from the calling code.
def even_odd(num):
The argument/parameter connection is done through passing object references, not by actually passing values around.
>>> def func(arg):
... print(arg, id(arg))
...
>>> value = 5
>>> print(value, id(value))
5 4307671712
>>> func(value)
5 4307671712

Amanda-Greg Robinson
2,932 Points@Chris Freeman, I wrote exactly what you have above, and even tested it in workspaces. It works just as it's supposed to, but the quiz is not accepting it. Is there a bug or any way to find out what the error is? I've checked and re-checked. Thanks
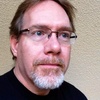
Chris Freeman
Treehouse Moderator 68,461 PointsAmanda-Greg Robinson, I reran my code above and it passes. The solution posted is only for Task 3. For Tasks 1 & 2, you need to add the two lines:
# Task 1 of 3: import random
import random
# Task 2 of 3: add 'start' variable
start = 5
If you're still having an error, I suggest opening a new Post from the challenge Help button. Tag me in it if you wish!

John Vieyra
2,826 Points#why doesn't this work??
import random
start = 5
def even_odd(num):
return not num % 2
while start:
number = random.randit(1, 99)
if even_odd(number):
print("{} is even".format(number))
else:
print("{} is odd".format(number))
start -= 1
[MOD: added ```python formatting -cf]
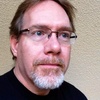
Chris Freeman
Treehouse Moderator 68,461 PointsThere are two errors:
- the
while
loop is indended too far which makes to part of the function definition. Instead it should be outside the function
- the method
randit
should berandint
Cena Mayo
55,236 PointsCena Mayo
55,236 PointsEdited code for clarity.