Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial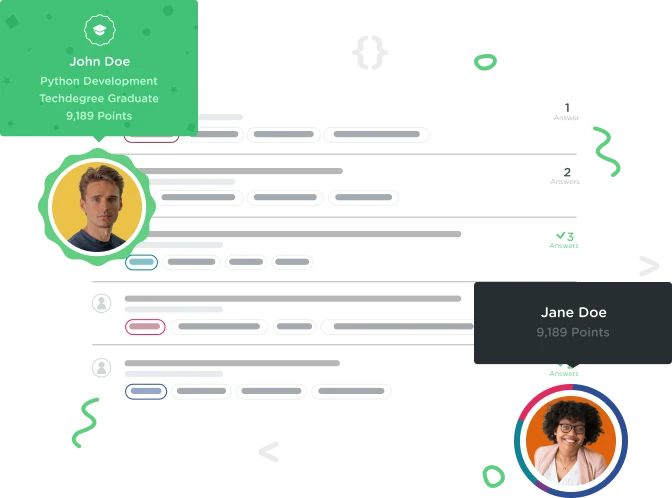

boo park
1,169 Pointsdict final challenge 1 of 4
I am stuck for the 50th time and I am sick and tired of copying other people's solutions. I am wondering if I am even close to answering this question correctly, and where and how I need to fix it. Please help!
Here is my code:
def most_classes(teachers):
max_count = {}
for class_names in teachers:
if len(class_names) > len(max_count):
max_count = class_names
else:
continue
for teacher in max_count:
return teacher
4 Answers
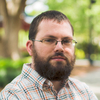
Kenneth Love
Treehouse Guest TeacherLet's look at your code:
def most_classes(teachers):
max_count = {} # Create a dictionary named max_count
for class_names in teachers: # for each thing in teachers, call the thing class_names
if len(class_names) > len(max_count): # if class_names, which will be the key aka teacher's name is longer than the number of keys in the max_count dictionary
max_count = class_names # set the max_count dictionary to the teacher's name
else:
continue
for teacher in max_count: # for each letter in the teacher's name, which we'll call teacher
return teacher # return the letter
It's a good try but I bet you got lost somewhere along the way with all of those tries. Let's think it through in English instead of code:
- Our function,
most_classes
is going to get a dictionary of stuff. The keys will be teachers' names and the values will be a list of their courses. - I should hold onto a highest count of classes so I can remember what the biggest set is so far.
- Well, if I have that, I should probably hold a teacher name, too, to remember who had the biggest set.
- Now I should go through all of the teachers in the dictionary, one name at a time.
- I'll get the list of courses that belongs to that teacher by using the teacher name as the key in the dictionary.
- If the length of that list is bigger than my high count, change the high count to the new length.
- Also, if it's bigger, change the teacher name that I have recorded to the key that I'm on.
- When that loop is all done, send back just the name of the teacher with the highest count.
Think you can take those 8 steps and turn them into code?

boo park
1,169 PointsFinally figured it out Kenneth! Thanks a bunch for your help. Couldn't have done it without you.
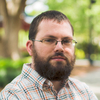
Kenneth Love
Treehouse Guest TeacherCongratulations! I know some of the challenges are extra...uhm...challenging. But the boost you get in both self esteem and knowledge makes them worth working through.

boo park
1,169 PointsI'll try again Kenneth. Thank you for your remark. I'll post the progress.
Alexander Torres
4,486 PointsThe bottom is what I came up with but idk how I got it right, and treehouse is letting me move on. It's returning the wrong answer I think.....am I correct? Sorry I am a noob at this stuff.
teachers = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(teachers): max_count = {}
if (teachers['Jason Seifer']) > (teachers['Kenneth Love']): max_count = {'Jason Seifer'} else: max_count = {'Kenneth Love'}
for teacher in max_count: return teacher