Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial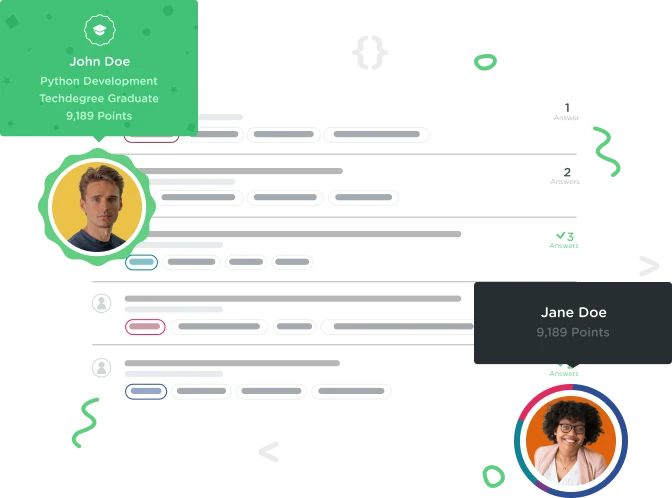

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsDictionary ... list of list
Question : create a function named stats that takes a dictionary of teachers and returns a list of lists in the format [<name>, <number of classes> ].
My code :
def stats(my_dict): my_list = list() my_list_of_list = list(); for key,value in my_dict.items(): temp_count = len(value) my_list.append((key,temp_count)) my_list_of_list.append(my_list) my_list = [] return my_list_of_list
Like to know where am i going wrong .....
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
my_dict = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(my_dict):
max_count = 0
max_class_teacher = ""
for key,value in my_dict.items():
temp_count = len(list(filter(bool, value)))
if max_count < temp_count:
max_count = temp_count
max_class_teacher = key
return max_class_teacher
def num_teachers(my_dict):
return len(my_dict)
def stats(my_dict):
my_list = list()
my_list_of_list = list();
for key,value in my_dict.items():
temp_count = len(value)
my_list.append((key,temp_count))
my_list_of_list.append(my_list)
my_list = []
return my_list_of_list
4 Answers

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsGot it. As it said list of list. I was unable to get it. So Thank You very much for clarification.

Trevor Currie
9,289 PointsIn your function, stats
, the line that is producing an error is:
my_list.append((key,temp_count))
Your variable my_list
has been established as a list, and now you're correctly appending an item to it, but you're giving it a tuple by putting "()" around the key, temp_count
. You need to use "[]" to establish it as a list - this function actually creates a list of a list of tuples.
Rethink how many lists you need as you iterate over your dictionary! There's a few too many in there...

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsI tried changing it to "[]" in append. But still there is some problem. Answer not accepted.
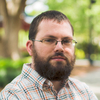
Kenneth Love
Treehouse Guest TeacherYour final list is going to look something like:
[[['Jason Seifer', 5]], [['Kenneth Love', 4]]]
You have too many levels of lists.

SUDHARSAN CHAKRAVARTHI
Courses Plus Student 2,434 PointsAs per the comments , i modified the append(), : as below:
# your code goes here
my_dict = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],'Kenneth Love': ['Python Basics', 'Python Collections']}
def stats(my_dict):
my_list = list()
my_list_of_list = list();
for key,value in my_dict.items():
temp_count = len(value)
my_list.append([key,temp_count])
my_list_of_list.append(my_list)
my_list = []
print(my_list_of_list)
return my_list_of_list
Output:
[[['Kenneth Love', 2]], [['Jason Seifer', 3]]]
I don't understand what's wrong . still not accepted in tree house.
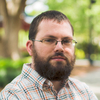
Kenneth Love
Treehouse Guest TeacherYou still have too many levels of lists. Let's break it down.
def stats(my_dict):
my_list = list() # creates a list []
my_list_of_list = list(); # creates another list
for key,value in my_dict.items():
temp_count = len(value)
my_list.append([key,temp_count]) # appends a new list to my_list
my_list_of_list.append(my_list) # appends my_list and its internal list to my_list_of_list
my_list = []
print(my_list_of_list)
return my_list_of_list
You don't need my_list
or the list you're appending to it. Just append a new list to my_list_of_list
.