Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial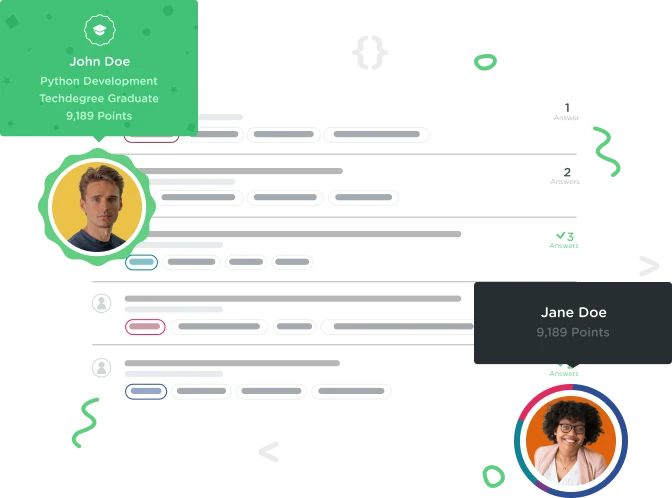

Joshua Clement
2,428 PointsDidn't know how to do this challenge
I tried doing this challenge and even going back to previous videos to figure out a way to do it but I just couldn't figure it out. Finally I came to watch this video to see how it's done and it seemed so easy. I feel like I should've been able to figure that out. Anyone else have the same problem as me?
3 Answers
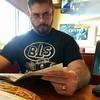
Alex Arzamendi
10,042 PointsStuff like that happens. You learn from practice and if you are a beginner it is totally fine to not be able to get things on the first try. Exploratory programming is a great way of getting used to the logic of how things work and sometimes having a little imagination comes a long way. Try this, open up a browser console and just start typing Javascript statements, create a name variable which holds your name and an age variable with your age, start adding names for your friends or favorite things and do some conditional statements for checking stuff up. I have been programming for a long time, so a code challenge like this is beyond basic at my stage. But, when I was starting out I would pull my hair like crazy because I couldn't figure things out. Practice and more practice, you can learn this, this can be learned.

Josian Acevedo
3,662 PointsHey! It took me quite some time to figure this out, mostly because I had no previous experience in JavaScript. I think the hardest part was figuring out the logic of this problem rather than knowing how to code it. I think I've finally got it right because I used to solve this kind of situations in other programs, but I think I wouldn't have be able to solve it if hadn't code in other languages. Don't let it get you down, the only way to keep going is practicing! You will see that the next time you encounter a similar problem, it will be easier to solve it.

Matthew Fung
8,643 PointsIf you find it difficult to understand a certain problem, it always helps to write down the step by step logic first, otherwise known as 'pseudo code'.
For example:
OUTPUT "What is 4 -2 ? "
INPUT user inputs their answer
STORE user input in a variable
IF answer = 2 THEN
STORE correctAnswers + = 1
ENDIF
Having a blank file and lots to do can be a bit overwhelming, so build bits in steps. I tend to think of all the things I would need to keep track of, and make variables for them.
In this instance I made 5 question variables, since we'll need to actually ask the questions and then a variable to hold how many questions the user got right.
var questionOne = prompt('What is 3-1?'),
questionTwo = prompt('What is 4/2 ?'),
questionThree = prompt('What is 2+4-1 ?'),
questionFour = prompt('What is 15*3 ?'),
questionFive = prompt('What is 2+2? '),
answersCorrect = 0,
answersWrong = 0;
Note: It's probably better to have the question prompt next to the answer checking bits of code, but I tend to keep all global scope variables near each other, for reference.
To check if the answer is correct, use the equality / strict equality operator. Remember to convert a string to an integer if needed.
if ( parseInt(questionOne) === 2) {
// my code here
}
I then just had a load of conditional statements to check if the value submitted was the correct answer and incremented the value of correct answers by 1.
// Check correct answers, answersWrong is just for testing purposes and should be deleted later.
if ( parseInt(questionOne) === 2) {
answersCorrect += 1;
} else {
answersWrong += 1;
}
if ( parseInt(questionTwo) === 2 ) {
answersCorrect += 1;
} else {
answersWrong +=1;
}
// and so on
I then used a comparison operator for checking the score, and giving the correct message.
if ( answersCorrect > 4 ) {
document.write('<h2>Congratulations!</h2>' + '<p>You scored ' + answersCorrect + " out of 5. You've been awarded the gold medal!</p>")
} else if ( answersCorrect >= 2 ) {
document.write('<h2>Congratulations!</h2>' + '<p>You scored ' + answersCorrect + " out of 5. You've been awarded the silver medal!</p>")
} else {
document.write('<h2>Congratulatiosn!</h2>' + '<p>You scored ' + answersCorrect + " out of 5. You've been awarded the bronze medal!</p>")
}