Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial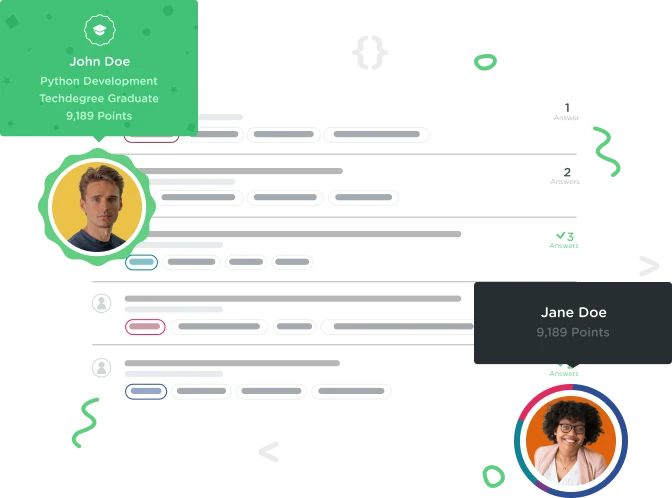

nadhvadlamu
99 Pointsdifference between instance variable , local variable , class variable ?
can i please know the difference between instance variable , local variable , class variable with examples ?
1 Answer
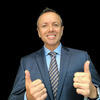
Marco van Vemden
12,553 PointsHi Nadh,
I know, some of this stuff can be (somewhat) complicated. Let's see if the following example helps:
The ThreeVariableTypes class below sets a class variable (@@class_variable), instance variable (@instance_variable), and local variable (local_variable), each to a random number below 10000.
class ThreeVariableTypes
# The class variable is set in the class, so not in a method
@@class_variable = rand(10000)
# The instance and local variables are set in a method.
def initialize
@instance_variable = rand(10000)
local_variable = rand(10000)
end
# All three variable types have been set above
# upon initialization of the class instance (or object)
# We use the getter methods below, to output the
# values of the three variable types.
def output_values
puts "Class variable :#{get_class_variable}"
puts "Instance variable :#{get_instance_variable}"
puts "Local variable :#{get_local_variable}"
# Add a divider to the output for readability
puts "*" * 25
end
# Important: If a certain variable is not available, the
# getter methods will return "----"
def get_class_variable
@@class_variable ||= "----"
end
def get_instance_variable
@instance_variable ||= "----"
end
def get_local_variable
local_variable ||= "----"
end
end
# Create 3 class instances (or 3 objects)
object_a = ThreeVariableTypes.new
object_b = ThreeVariableTypes.new
object_c = ThreeVariableTypes.new
# Output the class instance variables
object_a.output_values
object_b.output_values
object_c.output_values
Now, if you save the above code in a file (I named mine "variables.rb") and run the file in your Ruby workspace (just type: ruby variables.rb), your output will have different random numbers but will look similar to this:
treehouse:~/workspace$ ruby variables.rb
Class variable :2502
Instance variable :8198
Local variable :----
*************************
Class variable :2502
Instance variable :2584
Local variable :----
*************************
Class variable :2502
Instance variable :5026
Local variable :----
*************************
Above you see the values of the class variable, instance variable and local variable of 3 different class instances (or objects) separated by a divider. You will notice that:
- For all 3 class instances, the values of the class variables are the same
- For all 3 class instances, the values of the instance variables are different
- For all 3 class instances, the values of the local variable are not available (or "visible").
How come? This has to do with the scope or visibility of the the variable types:
- The class variable is set at the class definition (when the code is loaded), and that same variable is "visible" from within the class and all its class instances (or objects).
- The instance variable is set when the class instance is initialized (e.g. object_a = ThreeVariableTypes.new), and each class instance gets its own instance variable which is only "visible" from within methods of that class instance. Thats is why the get_instance_variable method can return the instance variable set in the initialize method.
- The local variable is set when the class instance is initialized, but is only "visible" from within the method it was set in. That is why the get_local_variable method returns an empty value; the value was set in the initialize method and is only "visible" in that method.
How to reset the value of the class variable? Run the "ruby variables.rb" command in your workspace again to re-load the class definition, and you will see the value of the class variable has changed into another random number. Just like below:
treehouse:~/workspace$ ruby variables.rb
Class variable :1174
Instance variable :7665
Local variable :----
*************************
Class variable :1174
Instance variable :8930
Local variable :----
*************************
Class variable :1174
Instance variable :4237
Local variable :----
*************************