Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial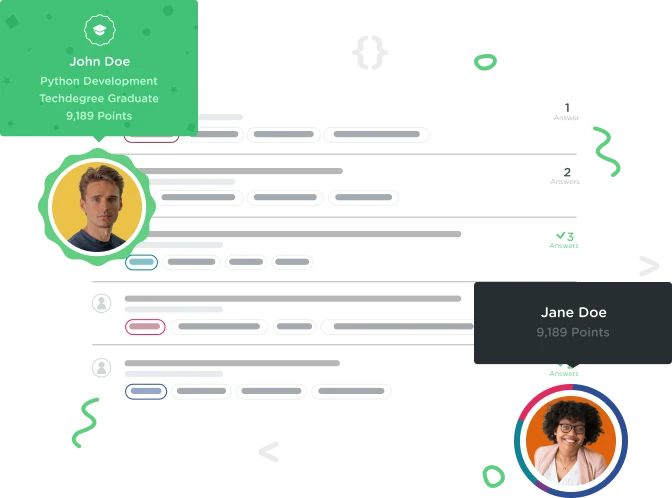
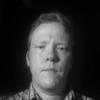
Robert Ellefson
5,672 PointsDifference between return and puts?
I just completed this challenge after struggling quite a bit. They ask you to return a string that says "The remainder of a divided by b is c" I have trouble with the fact that on these challenges you cant see whether there's any output or not, just a pass/fail response, so I tried to work it out in a workspace. I eventually came to the following code, which as far as I can tell should satisfy the challenge goals:
def mod(a, b) c = a%b puts "The remainder of #{a} divided by #{b} is #{c}."
end
mod(33, 5)
However when I put this code into the challenge form it stated something like "Make sure your string is in a nice format" I have no idea what they mean, since I can't see what the result of my code is.
I changed:
puts "The remainder...
to:
return "The remainder...
and it passed the challenge.
Here's where I'm confused. When I changed the puts command to return in my workspace, I got no output. Just now as I write this I changed:
mod(33, 5)
to:
puts mod(33, 5)
which again gave a string output like what they were asking for in the challenge.
So what does return vs. puts mean and why did it pass me if the code I input can't return the proper string?
I tried to look up return on Ruby-doc.org and they don't list it. I tried to find something on stackOverflow but couldn't make any sense of what I found there.
2 Answers
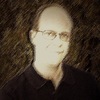
Jason Anders
Treehouse Moderator 145,861 PointsHopefully I can help to clarify.
Your method above (mod) takes in two arguments and performs the modulus on them. It then returns the values into the function/method (passed into your formatted sentence). This will not print or otherwise show itself to the user (returns don't).
Because you called this function (method) with puts, the value returned (your formatted sentence) will be printed to the screen for the user.
When you called this method puts mod(33, 5)
, you are passing 33 into a
and 5 into b
. You sentence is now "The remainder of 33 divided by 5 is 6" will be returned to the function, but only printed out with your puts
line.
Alternatively, you could do value = mod(33, 5)
. This will store the return value into the variable named value.
In summary, the return
in a function/method does just that -- it only returns a value to the function to be used later. It's how you call the function that will determine how (if) the values are displayed.
Hope this helps make sense... :)
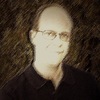
Jason Anders
Treehouse Moderator 145,861 PointsHey Robert. Welcome to Treehouse.
puts
will print something out so that it's visible to the user.
return
will only return the value to the variable and will not be visible to the user.
In the challenges, which are very picky and strict, you just need to pay attention as to whether the question asks you to 'return' or 'puts' the result. Either way, in the challenges, you won't see your output, but it's critical as to what the challenge asks for. If it says to 'return the...' and you use puts
, you will get a "Bummer..."
Hope that helps. :)
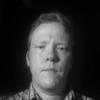
Robert Ellefson
5,672 PointsThanks for your reply, Jason, but I'm still confused about what's going on here. What values are going where in the following code?
My programming vocab is still weak so what's a variable and what's a value is a little confused for me. If you can tell me something along the lines of "33 is going to def mod(a) and then that's going to #{a} in the string" (I use this as an example because this is what I'm pretty sure I'm understanding properly about how the code is being read by the computer) to explain to me what the computer is doing with the return method then I think I might be able to wrap my head around it!
def mod(a, b)
c = a%b
return "The remainder of #{a} divided by #{b} is #{c}."
end
puts mod(33, 5)
Thanks!
Robert Ellefson
5,672 PointsRobert Ellefson
5,672 PointsI think I understand the flow, but what isn't making sense to me is what is happening with the return. It seems to me like a pointless exercise if all you're doing is making a program that creates a string that gets completed by the computer and then seems to go nowhere and do nothing. What is the return method for?
I'm trying to find some explanations online of return and coming up with very little. As far as I can tell, putting code like this into a larger program creates a kind of "mini program" (algorithm, i think is what it would be called?) that you can call up later somehow. Perhaps that's what you were alluding to with your remark about changing puts mod() to value = mod()? I tried changing my code to reflect this and found that I can then type puts value and it will print the string. I also found that I can create multiple values such as value1 = mod(a, b) and value2 = mod(a, b), assign different variables to each and then use puts value1 or puts value2 to print the resulting string. Maybe this is useful but right now I don't understand how.
I know it's probably too much to get into and it may be explained more thoroughly later so I don't want to compel you to write a lengthy response! Am I on the right track with this?
Jason Anders
Treehouse Moderator 145,861 PointsJason Anders
Treehouse Moderator 145,861 PointsYou are on the right track and will learn more advanced uses of functions other than just returning a hard-coded string. Functions can perform very complex 'functions' and just kind of wait for you to 'call' on them.
Your example of creating multiple variables and using the same function (but with different arguments) is one of the things that make functions so useful and important in programming. Instead of having to code that equation every time you want to figure the remainder of two numbers, you just call the function and pass in the arguments.
Granted this is a very simple function with only one line of code, but think about a highly advanced/complex function that could have 20, 30, or even more lines of code (conditionals, formulas, etc) that can take any number of arguments... That's not something you'd want to have to write out multiple times.
Now most functions will just
return
something, usually into a variable you declare. This way, if needed, you can manipulate the returned value (now the variable) and use it anyway that is needed, without altering or affecting the actual function. That's the main reason for thereturn
.You're getting there. There are much more complex and wonderful things to come! Keep Coding! :)
Robert Ellefson
5,672 PointsRobert Ellefson
5,672 PointsThank you for your help, Jason!