Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial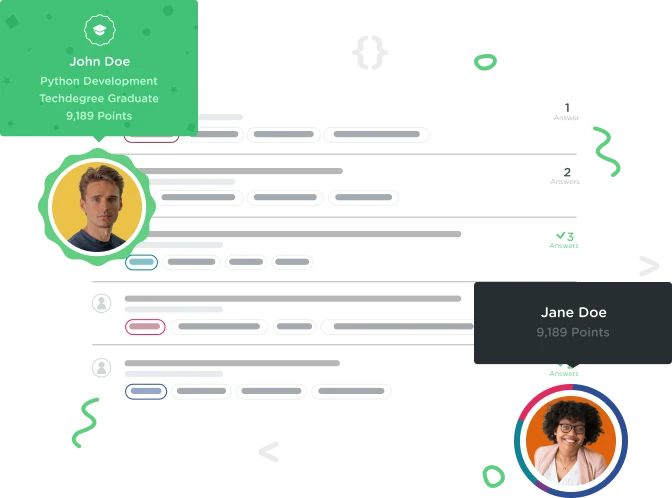
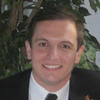
Hugo Amorim
Courses Plus Student 23,205 PointsDo numbers need to be encase in "quotes" when building a json object array?
For example: var students = [ { name : "Hugo", track : "Mac OSX", acheivements : 3, points : 23345 }, { name : "Sean", track : "Ios", acheivements : 1, points : 23 },
does it need to be :
var students = [ { name : "Hugo", track : "Mac OSX", acheivements : "3", points : "23345" }, { name : "Sean", track : "Ios", acheivements : "1", points : "23" },
4 Answers

zazza
21,750 PointsIt can be either. Both strings and numbers are valid JSON values. Choosing which one depends on how you are going to use it, although in the Part 2 video, it doesn't matter since JavaScript numbers are coerced into strings automatically. For example:
var stringVal = "23";
var numVal = 23;
console.log("Points: " + stringVal);
console.log("Points: " + numVal);
Both console.log
statement will function as expected since numVal
is automatically converted to a string. Personally, I would represent numbers are Number
s since it more accurately describes the data as a number rather than as a "numeric string" and allows for sensible operations. For example:
var stringVal = "23";
var numVal = 23;
console.log("Points: " + (stringVal + 1));
console.log("Points: " + (numVal + 1));
The first console.log
will return "Points: 231" while the second is most likely what you want (24). To remedy the first case, you'd have to do something like:
console.log("Points: " + (parseInt(stringVal, 10) + 1));
Which is arguably more convoluted.

Trevor Johnson
37 PointsGeorge is correct.
If the value will ONLY EVER contain numbers then leave out the quotes.
If it is possible that the value MAY contain letters, then always quote that value.
Incidentally, https://json-csv.com is a useful online tool for viewing JSON data.

Zeljko Porobija
11,491 PointsActually, Dave was not consistent in the video, some numbers are not in quotes. An oversight, quite excusable. In all programming languages it always depends on what you want to do with number. For my part, the numbers are there only for calculations. If you simply write a number as a data (e.g. age: 23) and you do not intend to calculate anything, keep it as a string. Working with databases can be trickier if your input is number than if it were a string.
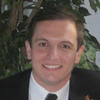
Hugo Amorim
Courses Plus Student 23,205 PointsThanks, makes complete sense.