Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial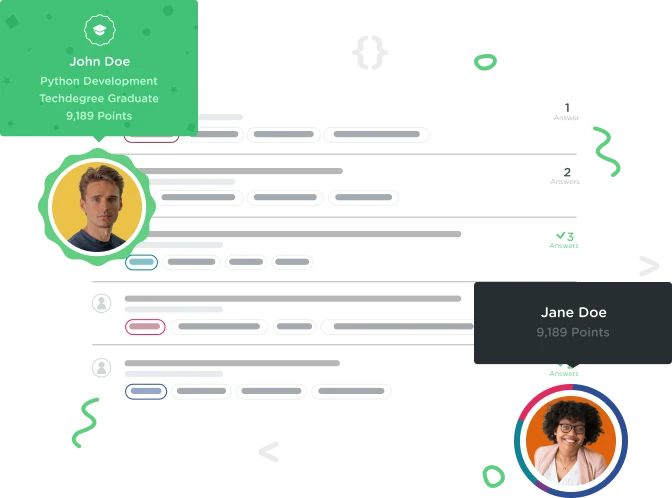

Kathryn Lawhorn
3,645 PointsDo... while challenge, confused by the suggestion about only running the prompt() method inside the loop. Aren't I?
var secret = prompt("what is the secret password?");
do { secret = prompt("what is the secret password?"); } while ( secret !== "sesame")
document.write(......)
The 'Bummer!' message is telling me that I should only run the prompt method once, within the loop, and I am so confused as to how I'm not doing that. And I have changed none of the code, only re-organized it into the do while. ??? Please don't say syntax error. Or please do? Dang.
var secret = prompt("What is the secret password?");
do {
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" );
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
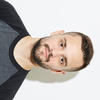
jacobproffer
24,604 PointsHey Kathryn,
Try writing it like this:
var secret;
do {
secret = prompt("What is the secret password?");
} while (secret !== "sesame");
document.write("You know the secret password. Welcome.");
As the error message indicates, you should only run the prompt method once. If you look at your code, you are prompting the user twice for input (When declaring the 'secret' variable and also within your do while loop). With that being said, it's important to remember that do while loops always run at least once.
Best,
Jacob
Kathryn Lawhorn
3,645 PointsKathryn Lawhorn
3,645 PointsThank you! That was what they wanted.
Follow up question though for my own understanding...
Wouldn't my code still produce the same result in terms of the function of the loop? I understand that I am prompting them once unnecessarily, and even if they entered 'sesame' the first time, I would still just prompt them again... I guess I just need to feel like I'm understanding the content after spending 30 minutes on this challenge. Charity backpat? I get this right?
jacobproffer
24,604 Pointsjacobproffer
24,604 PointsHey Kathryn,
There is one key difference between a while loop and a do-while loop.
Any code placed within a while loop will only run if the boolean condition of that loop is met.
Example:
This while loop will only run if the user enters anything other than a number or a number less than zero.
However, unlike while loops, do-while loops will always run at least once.
This do-while loop will basically do the same as the while loop. As mentioned, it will always run at least once but will only continue to run if the user enters anything other than a number or a number less than zero. You could most likely re-write the code to be more efficient but this is just to get the point across between the difference of while and do-while loops.
In short, while loops will only run if the boolean condition is true. Do-while loops will always run once but only continue to run if the boolean condition is true thereafter.
Best,
Jacob