Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial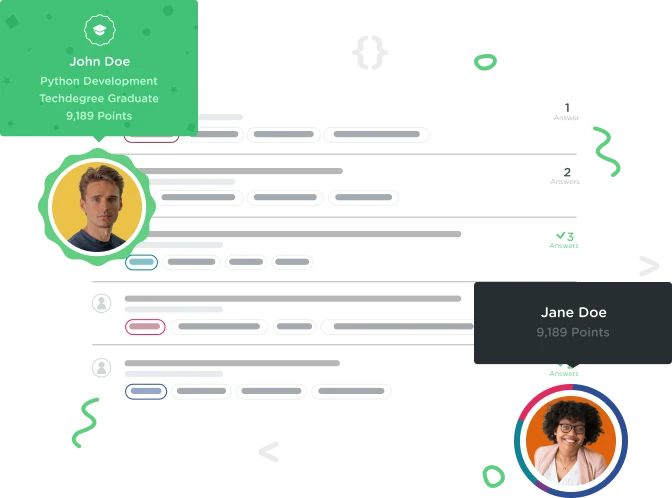

Harcharan Riyait
1,625 PointsDo While loop in endless loop
I have tried following along in the Do...While lesson, but when previewing the code, it keeps looping over and over. Can anyone see what I am doing wrong?
Thanks in advance
<script>
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
do {
guess = prompt("I am thinking of a number between 1 and 10. What is it?");
guessCount += 1;
if(parseInt(guess) === randomNumber){
correctGuess = true;
}
} while( ! correctGuess )
document.write("<h1>You guessed the number!</h1>");
document.write("It took you " + guessCount + " tries to guess the correct number " + randomNumber);
</script>
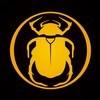
rydavim
18,814 PointsCan you elaborate on the problem you're encountering? Your code seems to be working correctly for me.
3 Answers

Stephen Layton
8,643 PointsIt doesn't look like your calling the getRandomNumber
function. Adding a randomNumber
variable above your do should do the trick.
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
var randomNumber = getRandomNumber(10);
do {
guess = prompt("I am thinking of a number between 1 and 10. What is it?");
guessCount += 1;

Harcharan Riyait
1,625 Points@Stephen Layton: Isn't the getRandomNumber called at the top of the script?
i am confused because this is the code from the lesson. It's stopped me dead on the loops section because I don't uunderstand why it gets stuck in the loop. I have looked for a line I may have missed when following along, but can't see it.
@rydavim: The loop carries on over and over asking for the random number. I try each number from 1 through 10 but none work.
Again, this is the code from the lesson as close as I could match it.

Harcharan Riyait
1,625 PointsOk, so I threw the code into JSBin and now it reaches the end with the 'correct number' message regardless of the number I input. I could input 1 over and over and it will still end saying that I guessed the correct number 8 or 5 or 7, etc.
So confused!

Iain Simmons
Treehouse Moderator 32,305 PointsCan you open the JavaScript console and report back any errors you're getting in either case?
Alternatively, you can 'watch' the value of the variables within the function by adding a breakpoint in the sources tab of the dev tools (if using Chrome), but that's a little more advanced.
Pier Yos
15,721 PointsPier Yos
15,721 PointsIt stops when you enter the correct number. It worked fine when i tested.