Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial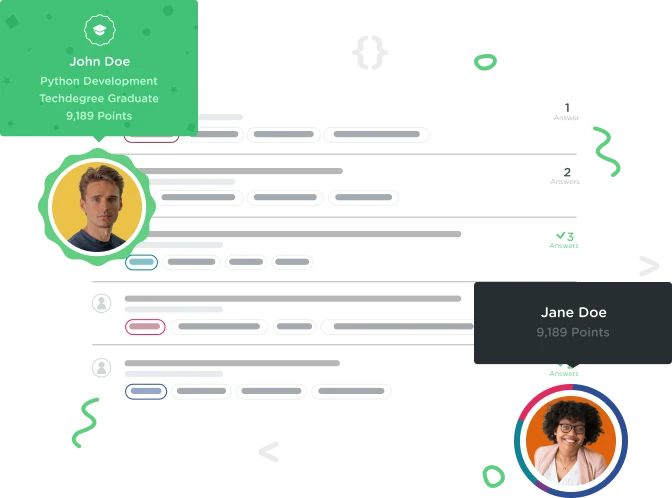
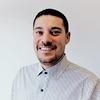
David Dehghani
6,992 PointsDo while loops
I'm prompting the user the question and the condition is checking if it matches, but I'm not sure how to declare the variable before starting the do while loop.
Thanks!
var secret = "sesame";
do {
secret = prompt("What is the secret password?");}
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
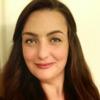
Jennifer Nordell
Treehouse TeacherHi there! I can see you've worked on this and maybe even overworked it a bit, so I'm going to give some hints and pseudocode here.
Hints
- Try declaring the secret to be set as an empty string.
- Your while loop is set up incorrectly
- Only one prompt is needed
pseudocode
declare variable secret
begin do {
prompt the user for their secret word guess
} while the secret is not sesame
print success message
Hope this helps, but let me know if you're still stuck!
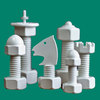
Steven Parker
231,236 Points
Your declaration is good, but your loop syntax needs a bit of work.
The syntax for a "do...while" loop looks like this:
do {
// body of loop code goes here
} while ( /* condition expression goes here */ );
An advantage of using this type of loop in this situation is that you will need only one prompt statement.
Also, while your variable declaration should pass as-is, you could reduce it by leaving off the initial assignment. The initialization is not needed here as it will get overwritten when it is assigned with the return value of the prompt.