Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial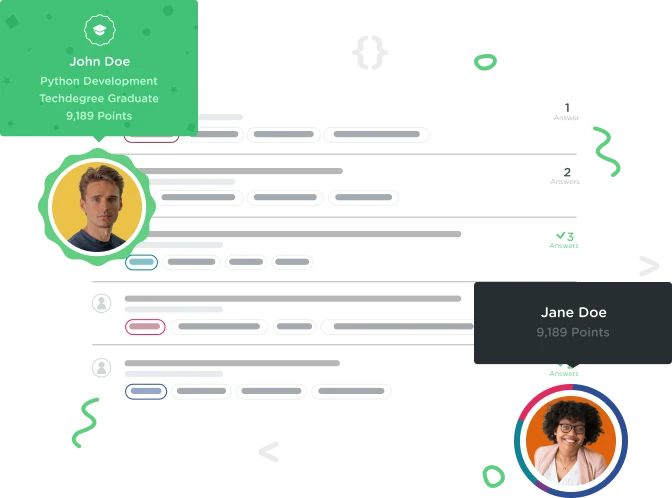

Alexander Melo
4,297 PointsDo While Loops -- Difference between 'not' operators?
Guil in this video uses the ! correctGuess. I was wondering if this is the same as writing correctGuess !== true ?? Please tell me the difference between these two operators.
4 Answers
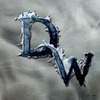
Hugo Paz
15,622 PointsHi Alexander,
They are similar but there is a crucial difference. The !correctGuess is not strict like the !== (or ===).
Let me give you an example:
var check = 0;
//case 1
if(!check){
console.log('In this case 0 is evaluated to false');
}
//case 2
if(check==false){
console.log('In this case 0 is evaluated to false as well');
}
//case 3
if(check===false){
//this will fail because 0 is not strictly false
}
So if correctGuess is a boolean value, both options are the same. But if you use a value, like 0, both will work differently as you saw above.
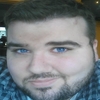
Marcus Parsons
15,719 PointsHey Alexander,
Using !variableName
is a way to check that a variable contains any falsey values. Falsey values are: false, 0 (or 0.0), "", null, NaN, and undefined. It's a good catch all to check to see if a variable is empty, not declared, not false, etc. and then do some code if it is one of those things.
if (!correctGuess) {
//do code
}
//The above is the same as writing:
if (correctGuess === false || correctGuess === "" || correctGuess === 0 || correctGuess === null || correctGuess === NaN || correctGuess === undefined) {
//do code
}
As you can see, writing !correctGuess
is a much faster and more efficient way of checking to make sure something is a falsey value than writing out all of those OR statements.
Here are some logic tests that you can copy and paste into your web console (or on a test page):
var a = 0;
if (!a) {
alert(a + ' is a falsey value!');
}
var b = false;
if (!b) {
alert(b + ' is a falsey value!');
}
var c = "";
if (!c) {
alert(c + ' is a falsey value!');
}
var d = null;
if (!d) {
alert(d + ' is a falsey value!');
}
var e = NaN;
if (!e) {
alert(e + ' is a falsey value!');
}
var f; //undefined
if (!f) {
alert(f + ' is a falsey value!');
}
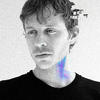
derekverrilli
8,841 PointsYes, they both mean the same thing.
An aside, Dave McFarland teaches this course, not Guil Hernandez ;)
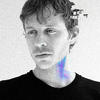
derekverrilli
8,841 PointsHugo explained it much better.
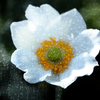
ellie adam
26,377 PointsHi Alexander,
These are basic operators.
== equal to x == 8 false
x == 5 true
=== equal value and equal type x === "5" false
x === 5 true
!= not equal x != 8 true
!== not equal value or not equal type x !== "5" true
x !== 5 false
greater than x > 8 false < less than x < 8 true
= greater than or equal to x >= 8 false
<= less than or equal to x <= 8 true
http://www.w3schools.com/js/js_comparisons.asp https://docs.oracle.com/javase/tutorial/java/nutsandbolts/while.html