Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial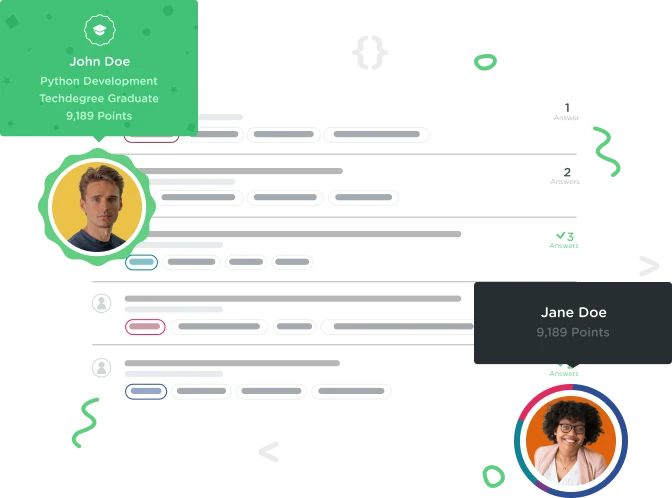
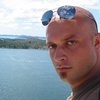
Giuseppe Ardito
14,130 Points"Do... While" to complete the challenge (and some other details added). Please, comment my code
Hi guys,
I just wanted to share with you my solution to this challenge. I confess I took a while to make everything work properly!
As you can see I used a "While... Do" loop and added one more thing: if you type nothing and enter, you will see a message saying "Type something", while if you type something that doesn't match any student name, then "No Student found" will appear. Please feel free to suggest any improvement or comment any part of the code. Thanks!
// Declaring the functions
var message = '';
var student;
var search;
var flag = false;
// Print function
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// This function gets the key values from the students objects and returns it.
// Also sets flag to true so that the 'No student found' message isn't displayed
function getStudent(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
flag = true;
return report;
}
// Do While loop begins
do { // get student name to search from the user
search = prompt('Search by name or type "Quit" to terminate');
// if user clicks on cancel, no null error message appears in
// the console as the loop breaks immediately before
// to get to the .toLowerCase() function, that makes the error appear
if (search === null) {break;} else if ( search === '') { print('<h2>Type something</h2>');}
// above, if the users just presses 'Enter' typing nothing, he will get a 'Type Something' message
// Starting to loop through the students array of objects
for (var i = 0; i < students.length; i += 1) {
student = students[i];
// if the search variable matches a name (all goes lower case)
if (search.toLowerCase() === student.name.toLowerCase() ) {
// then the function stores the correspondent html in 'message' and prints the message
message += getStudent(student);
print(message);
}
}
// if the flag is false (in other words the search variable doesn't
// match any name and the getStudent function doesn't run)
// then a 'No student found' message is shown
if (flag === false && search !== '') { print('<h2>No student found</h2>'); }
// flag goes back to false to be reused at the next search
flag = false;
// Same for message, only the result of the last search will be displayed
message = '';
// if the word quit is input, the program exits the loop
} while (search.toLowerCase() !== 'quit')
// and a final message appears! :)
print('<h2>Thanks for testing this script! :)</h2>');
5 Answers

Victoria Felice
14,760 PointsThank you for sharing your solution! If you add a "+" to your line (number 33, my guess) like this:
message += getStudent(student);
it will print a second student with the name Jody.
Also, when I typed Quit with the capital "Q", program did not terminate. I bet you know the solution to this problem! :)

Daniel Newman
Courses Plus Student 10,715 Pointsvar message = '';
var student;
var search;
var flag = false;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudent(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
flag = true; // what this flag for?
return report;
}
do {
search = prompt('Search by name or type "Quit" to terminate');
// here or on while(expression) is better to turn it lowercase. Isn't it?
if (search === null) {
break;
} else if (search === '') {
print('<h2>Type something</h2>');
}
for (var i = 0; i < students.length; i += 1) {
student = students[i]; // is it predefined "students" set?
if (search.toLowerCase() === student.name.toLowerCase()) {
message = getStudent(student);
print(message);
}
}
if (flag === false && search !== '') { // flag true only once? If search === '' don't we print "Type some"?
print('<h2>No student found</h2>');
}
flag = false; // Why?
message = ''; // It's useless also
} while (search !== 'quit')
Let's fix it:
var students = [
{ "name" : "Bill",
"track" : "Javascript",
"points" : "over9000",
"achievements" : "King of Dancehall"
},
{ "name" : "John",
"track" : "Perl",
"points" : "777",
"achievements" : "Newbie"
}
];
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
if( outputDiv === null ) {
print("No HTML block we need");
}
outputDiv.innerHTML = message;
}
function getStudent(student) {
var report = '<h2>Student: ' + student.name + '</h2>'
+ '<p>Track: ' + student.track + '</p>'
+ '<p>Points: ' + student.points + '</p>'
+ '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
do {
search = prompt('Search by name or type "Quit" to terminate').toLowerCase();
if (search === null || search !== '') {
print('<h2>Type something</h2>');
print('<h2>No student found</h2>');
break; // ? have no idea about it
}
for (var i = 0; i < students.length; i += 1) {
student = students[i]; // is it predefined "students" set?
if (search.toLowerCase() === student.name.toLowerCase()) {
message = getStudent(student);
print(message);
}
}
} while (search !== 'quit');
Try to figure out, why it doesn't work. Regards.
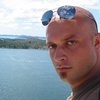
Giuseppe Ardito
14,130 PointsHi Daniel, Thank you for commenting my code!
Actually my code is perfectly working! The flag is a way I found to make sure the message "No student found" isn't displayed when a search matches a student name.
I provided to use the toLowerCase() function in the condition of this if if (search.toLowerCase() === student.name.toLowerCase())
Also, yes, the students object is defined in a separate file as from the example of the concerned video lesson.
I use the break if search === null to avoid the console error message mentioned in the video whenever you hit esc or "cancel".
To make my code more readable, I will comment it all the way though, so it will be way easier to get at a first look
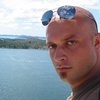
Giuseppe Ardito
14,130 PointsHi again,
I just commented the whole script so everyone can easily understand it.
I hope this can help.
Thanks
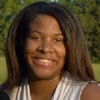
Marlinda Davis
7,423 PointsHello, this was helpful to me. I was getting the same problem where it would keep popping up after I typed in a student that was not in the list. I will try to add the var flag to my code. Thanks!
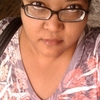
Amy Rutherford
9,659 PointsI came back to this code tonight and used the flag variable and got it working fairly quickly. Thank you!
Giuseppe Ardito
14,130 PointsGiuseppe Ardito
14,130 PointsHi Victoria, thanks for commenting
I added the + before I wrapped the getStudent code in a function and it was displaying also multiple students with the same name. I will find the way to fix this back and update the code above.
Also, the quit keyword actually stops the loop but you see the "No student found" message as the program goes through the loop at least once, I will try to fix this too and update my code!
Thanks!
Victoria Felice
14,760 PointsVictoria Felice
14,760 PointsI typed Quit with the capital letter as suggested in your prompt. :)
Giuseppe Ardito
14,130 PointsGiuseppe Ardito
14,130 PointsI have just applied your suggestions. Great!
Giuseppe Ardito
14,130 PointsGiuseppe Ardito
14,130 Pointsahh I see what you mean.
I have just taken out the .toLowerCase() method from the if condition and added it to the prompt
Now it should work correctly! This is fun!
Victoria Felice
14,760 PointsVictoria Felice
14,760 PointsGood job, Giuseppe! Another way is to put it in your 'do while' condition:
while (search.toLowerCase() !== 'quit');
I agree, programming/solving problems is so much fun!!