Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial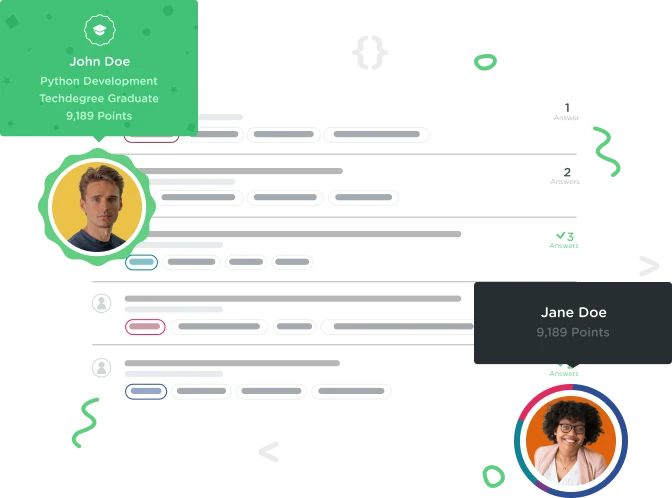

Anshul Laikar
4,428 PointsDoes the placement of this line of code matter?
This is Kenneth's code:
import os
import random
# draw grid
# pick random location for player
# pick random location for exit door
# pick random location for the monster
# draw player in the grid
# take input for movement
# move player, unless invalid move (past edges of grid)
# check for win/loss
# clear screen and redraw grid
CELLS = [(0, 0), (1, 0), (2, 0), (3, 0), (4, 0),
(0, 1), (1, 1), (2, 1), (3, 1), (4, 1),
(0, 2), (1, 2), (2, 2), (3, 2), (4, 2),
(0, 3), (1, 3), (2, 3), (3, 3), (4, 3),
(0, 4), (1, 4), (2, 4), (3, 4), (4, 4)]
def clear_screen():
os.system('cls' if os.name == 'nt' else 'clear')
def get_locations():
return random.sample(CELLS, 3)
def move_player(player, move):
x, y = player
if move == "LEFT":
x -= 1
if move == "RIGHT":
x += 1
if move == "UP":
y -= 1
if move == "DOWN":
y += 1
return x, y
def get_moves(player):
moves = ["LEFT", "RIGHT", "UP", "DOWN"]
x, y = player
if x == 0:
moves.remove("LEFT")
if x == 4:
moves.remove("RIGHT")
if y == 0:
moves.remove("UP")
if y == 4:
moves.remove("DOWN")
return moves
monster, door, player = get_locations()
while True:
valid_moves = get_moves(player)
clear_screen()
print("Welcome to the dungeon!")
print("You're currently in room {}".format(player))
print("You can move {}".format(", ".join(valid_moves)))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if move in valid_moves:
player = move_player(player, move)
else:
print("\n ** Walls are hard! Don't run into them! **\n")
continue
# Good move? Change the player position
# Bad move? Don't change anything!
# On the door? They win!
# On the monster? They lose!
# Otherwise, loop back around
I have an issue with the placement of
monster, door, player = get_locations()
Does it matter if that line is just before the while loop or can it be placed anywhere? Does it affect the order of initialisation of these variables or something??
1 Answer

Moosa Bonomali
6,297 PointsPython statements are executed sequentially and so order is important
If I do something like this
hello()
def hello():
print("hello")
This code will give a NameError, because the code that declares the hello() function has not yet been executed.
So in your case
monster, door, player = get_locations()
has to be called after the get_locations() function.
He could have placed it just after the
def get_locations():
return random.sample(CELLS, 3)
but I think it was good presentation to declare all your functions 1st
Secondly, the monster, door and player variables have to be declared before the while loop, because they are referenced within the while loop. If the code was placed after the while loop, an error will be generated
Take note that for the same reason;
move_player(player, move)
get_moves(player)
functions also needed to be declared before the while loop.

Anshul Laikar
4,428 PointsThanks a lot, your answer was very helpful :)
Moosa Bonomali
6,297 PointsMoosa Bonomali
6,297 PointsPython statements are executed sequentially and so order is important
If I do something like this
This code will give a NameError, because the code that declares the hello() function has not yet been executed.
So in your case
monster, door, player = get_locations()
has to be called after the get_locations() function.
Secondly, the monster, door and player variables have to be declared before the while loop, because they are referenced within the while loop. If the code was placed after the while loop, an error will be generated
Take note that the
functions also needed to be declared before the while loop.