Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial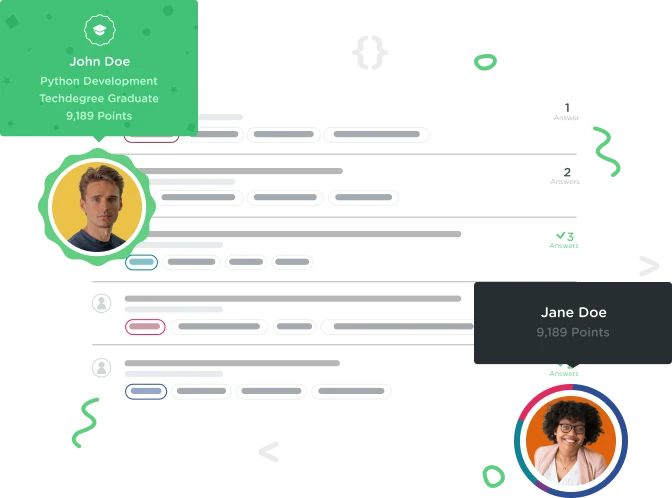
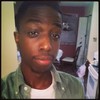
abou93
14,318 Pointsdon't even know where to start with this one. challenge task 1 of 1 in word_count.py
so heres the directions: Create a function named word_count() that takes a string. Return a dictionary with each word in the string as the key and the number of times it appears as the value.
I'm just so stuck I'm not even sure what is being asked.
heres my code:
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
string_dict = "{i} {am} {that} {i} {am}"
split_string = string_dict.split()
def word_count(string_dict):
for word in split_string:
if string_dict[word]:
string_dict[word] += 1
else:
string_dict[word] = 1
return split_string
13 Answers
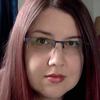
Tree Casiano
16,436 PointsI was stumped on this one for a long time. Here's what I figured out. I'll break it down into steps.
The argument that goes inside of the function is a string, not the dictionary. I simply called it a_string. You do not need to define the actual words in the string. Any string can be passed into this function.
Within the function, you should create a variable that is an empty dictionary. This is done by using empty curly brackets, i.e., string_dict = {}.
Create a for loop that iterates through each word in a list made of the string. You can use a_string.split() to create this list.
Create a conditional statement within this loop that increments the value of each key by 1 if it is already present or creates a new key with a value of 1 if it is not already in there.
The last step in the function is to return the dictionary.
def word_count(a_string):
string_dict = {}
for word in a_string.split():
if word in string_dict:
string_dict[word] += 1
else:
string_dict[word] = 1
return string_dict

Damian Podraza
3,199 Pointsdef word_count(str):
my_dict = {}
str.lower()
new_str = str.split()
for words in new_str:
my_dict[words] = new_str.count(words)
return my_dict
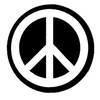
john larson
16,594 PointsHey Damian, I like yours
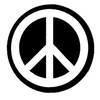
john larson
16,594 PointsI took your idea and tightened it up a bit.
def word_count(str):
count_dict = {}
for words in str.lower().split():
count_dict[words] = str.count(words)
return count_dict
``
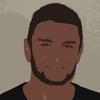
jsdevtom
16,963 PointsPython has a built in counter tool for this:
from collections import Counter
def word_count(string):
words = string.split()
return dict(Counter(words))

Lisa Burbon
1,030 Pointsdef word_count(string):
string = string.lower()
new_string = string.split(' ')
final = {}
for key in new_string:
if key in final:
final[key] += 1
else:
final[key] = 1
return final

Michel Näslund
18,583 PointsYou can use dictionary comprehension. Building the dictionary from a list will get you the keys and the count.
def word_count(string):
list_of_words = string.lower().split()
string_dictionary = {word: list_of_words.count(word) for word in list_of_words}
return string_dictionary

Eduardo Velasquez
15,697 PointsReturn a dictionary with each word in the string as the key and the number of times it appears as the value.
-> This means that your function should return something like this { 'i': 2, 'that': 1, 'am' : 2 }
in the first key-value pair that gets returned, 'i' gets passed into the for loop and returns 2 as the value because the for loop iterates over the string_dict and matches 'i' twice. It then does the same for the other two words and builds the dictionary with 'i', 'that', and 'am' as the keys.

MUZ140952 Brighton Phiri
3,207 PointsHie help i tried that and everything else but it keeps saying SyntaxError: else:
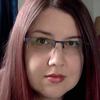
Tree Casiano
16,436 PointsPlease paste your code using the Markdown Cheatsheet and I'll take a look and try to help.
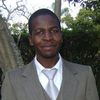
MUZ140920 Kudakwashe Murungu
6,316 PointsTree Casiano I tweaked your code a little bit. This is what i came up with
def word_count(a_string):
string_dict = {}
for word in a_string.split():
if word in string_dict:
string_dict[word] += 1
else:
string_dict[word] = 1
return string_dict
a_string = "I am what i am because of God"
a_string = a_string.lower()
print(word_count(a_string))
Thanks a lot, I was also stuck on this but your breakdown simplified the whole challenge. Thanks - happy coding

MUZ140952 Brighton Phiri
3,207 Points@ Tree Casio here is my code but it keeps saying syntax error: else
def word_count(a_string): string_dict = {} for word in a_string.split(): if word in string_dict: string_dict[word] += 1 else: string_dict = 1 return srting_dict

MUZ140952 Brighton Phiri
3,207 Pointshelp: how do you use Markdown Cheatsheet to copy and paste a code?
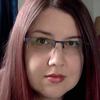
Tree Casiano
16,436 PointsThere's a link to the Markdown Cheatsheet just above the buttons when you post a comment or add an answer.
Markdown is a short-hand syntax for easily converting text to HTML.
Code Wrap your code with 3 backticks (```) on the line before and after. If you specify the language after the first set of backticks, that'll help with syntax highlighting.
```html
<p>This is code!</p>
```
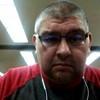
micram1001
33,833 PointsThis sounds stupid but the backtick key (`) is located on the upper left side of the keyboard. The apostrophe key (') is on the right side (before the num lock key pad and movement keys).

Cubes School
Courses Plus Student 17,884 PointsThanks a lot!

Douglas Ngwenya
3,132 PointsI modified Lisa's answer as follows:
def word_count(string): string = string.lower() new_string = string.split() final = {} for key in new_string: if key in final: final[key] += 1 else: final[key] = 1 return final

bowei zhou
1,762 Pointsdef word_count(sentence): words = sentence.split() words_lower = [] dic = {} for key in words: key = key.lower() words_lower.append(key)
for key1 in words_lower:
dic[key1]=words_lower.count(key1)
return dic
word_count(sentence)
abou93
14,318 Pointsabou93
14,318 PointsThank you i finally got it! all you're doing is increasing the count. I started off not fully understanding the question exactly so i was confused where to go about it
sakatagintoki
12,624 Pointssakatagintoki
12,624 PointsMy cheers to you sir.
Annie Scott
27,613 PointsAnnie Scott
27,613 Pointsthank you so much for explaining it and showing the answer, it taught me alot, was just so lost.
Simon Hemmings
11,922 PointsSimon Hemmings
11,922 PointsGreat answer helped a lot!
Neil Gordon
8,823 PointsNeil Gordon
8,823 Pointsthank you Tree Casiano
jamie threadgold
925 Pointsjamie threadgold
925 PointsSilly question perhaps but what part of this code appends the 'word' to 'string_dict' in the first place???
Thanks, Jamie