Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial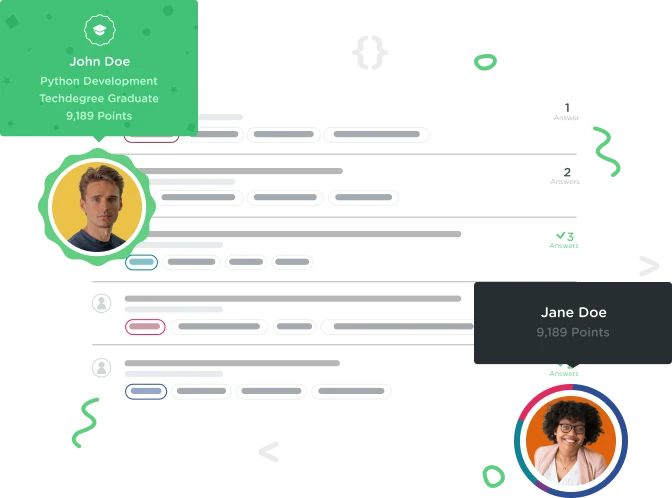
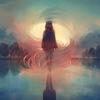
Aananya Vyas
20,157 PointsDon't know what to do?!
??????
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init (red : Int , green: Int , blue : Int , alpha: Int , description: String){
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red) ,green: \(green) ,blue: \(blue) , alpha:\(alpha)"
}
func getcolor (red : Double , green: Double , blue: Double , alpha: Double){
return "red: \(red) ,green: \(green) ,blue: \(blue) , alpha:\(alpha)"
}
}
let acolor = getcolor (red : 86.0 , green:191.0, blue:131.0, alpha: 1.0)
1 Answer

Alexander La Bianca
15,959 PointsHi, You do not need to include the description property in the initializer function. Here is how I would create the RGBColor struct. Notice that the init takes Double values and not Int
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
By passing in all the Double values you can create a string and assign it to the description property. That way you automatically create a value for the description property every time you initialize a new RGBColor object.
You can access the description property the following way now:
let someColor = RGB(red: 86.0, green:131.0, blue: 91.0, alpha:0.8)
let someDescription = someColor.description
//someDescription will be "red: 86.0, green: 131.0, blue: 91.0, alpha: 0.8"
Aananya Vyas
20,157 PointsAananya Vyas
20,157 PointsThanks a lot!!!!