Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial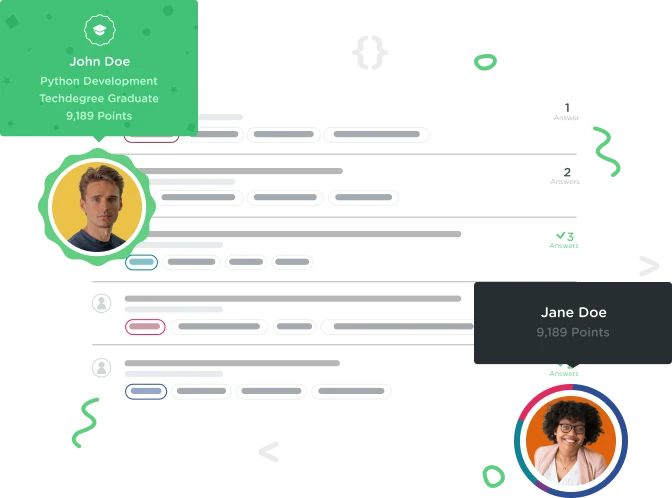

Heriberto Perez
1,298 Pointsdon't understand the question about the variables Monday and today chanllenge task 1 of 1
can anyone help me?
var money = 9;
var today = 'Friday';
if ( money >= 8 || today === 'Friday' ) {
alert("it's Friday, but i don't have enough money to go out");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
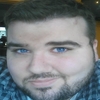
Marcus Parsons
15,719 PointsHey Heriberto,
In order to this challenge, you must think about the logic presented. As Theodore stated, the current logic is an || (OR) based logic. It's saying if either of the cases is true to execute the if statement. But each one of these alerts tells you that it costs less and less each time to do something as you're checking how much money you have and it needs to be a night that most people have off: "Friday". Notice the keyword I used in my sentence: and. You need to use && (AND) logic instead of the || (OR) logic.
After that, you can see that the last else-if is completely wrong. You know that using the ! with a pair of == signs means "is not equal to". So, the last else-if is saying: If today is not Friday, then say, "It's Friday, but I don't have enough money to go out". That doesn't make any sense. So, if we replace the ! with another = sign, we get === which means "is strictly equal to". Then, the logic works out because we're asking if today is strictly equal to Friday and we know that it is by looking at the today variable.
You shouldn't have changed the order of the alert statements because there were only a few things you need to change. Here is the solution to the challenge:
var money = 9;
var today = 'Friday'
//changed || to && logic
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
//changed the !== to ===
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
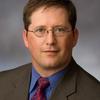
Ted Sumner
Courses Plus Student 17,967 PointsI think you don't understand the code because I think the code is wrong. || = or. Your current code says:
If you have more than or equal to 8 OR it is Friday say its Friday, but I don't have enough money to go out. It should be if money is less than 8 AND its Friday . . .
Else if money is greater than or equal to 50 OR its Friday say time for a movie and dinner.
It goes on. I think the script should read:
var money = 9;
var today = 'Friday';
if (money <= 8 && today === 'Friday') {
alert("It's Friday, but I don't have enough money to go out");
} else if (money>= 50 && today === 'Friday') {
alert("Time for a movie and dinner");
} else if (money > 10 && today === 'Friday') {
alert ("Time for a movie.");
} else if (today === 'Friday') {
alert ("It's Friday, but I don't have enough money to go out");
} else {
alert ("this isn't Friday. I need to stay home.");
}
It appears to me that you have and/or reversed and some of your conditions are not correct. Once you get your code correct, it will make it more sense.
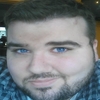
Marcus Parsons
15,719 PointsAlthough your code passes the challenge, the logic presented is redundant: specifically the first if-statement. The last else-if takes care of all money scenarios that where money
is 10 or less and is why the code passes the challenge. In fact, you changed a fundamental part of the challenge which was an alert statement that says "Time to go to the theater" if you have money greater than or equal to 100. I don't know why you deleted it, but the first if-statement should remain intact as is.
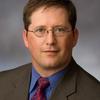
Ted Sumner
Courses Plus Student 17,967 PointsI did not refer to the challenge itself. Rather, I rewrote the code he had with the proper logic without analyzing it for redundancy or for passing the challenge. I view the fundamental issue with the post is the logic, which is what I addressed. You are correct in the criticism of the overall code I wrote.
I did delete an answer that was a short example using "or" because I don't think what I wrote would work and I didn't feel like going through the effort to see if I could write the code using or.
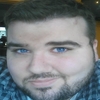
Marcus Parsons
15,719 PointsYour answer does not have the proper logic, Theodore. You should examine the actual challenge more closely, and then re-evaluate your answer. I always look back to the original challenge to ensure that I'm not modifying the overall goal and logic of the challenge.
And you cannot write this logic using or logic because it violates the logic of the challenge. Each original alert is meant for the day of "Friday" and the amount of money available except for the very last else statement which is for if it's any other day of the week.
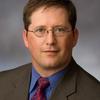
Ted Sumner
Courses Plus Student 17,967 PointsI don't see how the logic is incorrect. I agree the first and last if are redundant. The first if could be <=10 and drop the last if or drop the first if and leave the last as is. Otherwise I think the logic is sound. Please tell me if there is a flaw in the logic other than the redundant lines.
Your practice of going to the challenge is a good one that I should do in future.
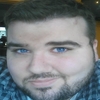
Marcus Parsons
15,719 PointsYou're not taking into account the original case presented when the money is greater than or equal to 100. There is no need for the first-if statement (which leaves out if money is 9 or 10 anyways). The last else-if takes care of scenarios when money is 10 or less. Redundancies in logic are incorrect because they do not belong. They waste valuable time and efficiency by being present in the code. This is all constructive criticism to learn from.
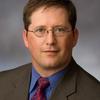
Ted Sumner
Courses Plus Student 17,967 PointsLike I said in the beginning, I used Heriberto's code for the numbers. I did not go back to the original problem.
Likewise, I acknowledged the redundancy issue and asked if there was something OTHER THAN the redundancy that is a logic error (not value compliance with the challenge). I don't see one and am interested in if you think there is an error OTHER THAN the redundancy.
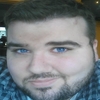
Marcus Parsons
15,719 PointsI plainly said your answer does not have the proper logic, Theodore. The keyword there is proper. I didn't say it didn't work or that it is incorrect. I even originally said "Although your code passes the challenge" implying that it works. I then went on to say that redundancies are incorrect because they waste time and efficiency. This conversation is becoming redundant because I've said all this before, and I'm not going to say it again.