Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial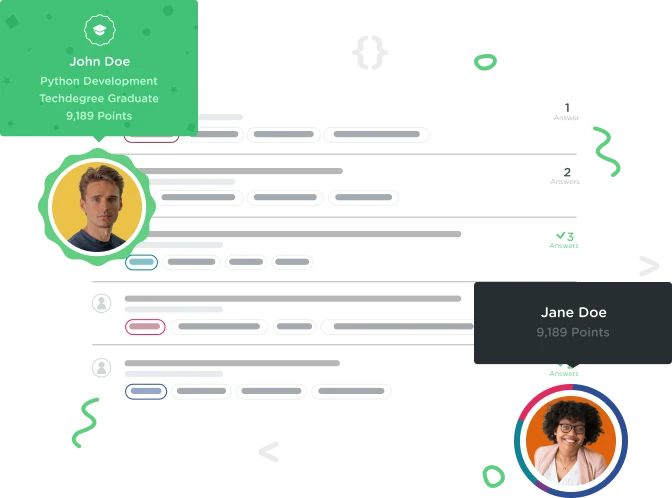
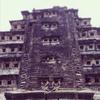
Polo Moreno
11,782 PointsDon't understand this. who make in this challenge ?
this challenge say this
Use a for or while loop to iterate through the values in the temperatures array from the first item -- 100 -- to the last -- 10. Inside the loop, log the current array value to the console.
can you help me please?
var temperatures = [100,90,99,80,70,65,30,10];
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
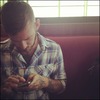
Erik McClintock
45,783 PointsJosue,
For this challenge, you'll need to recall two separate things from your courses: for (or while) loops, and the console.log statement.
Let's see how this would look using both types of loops:
("for" loop - the easier loop)
// declare your condition, which is that you have a variable called "i" that equals 0, and for as long as that variable is equal to less than the length of your temperatures array (which is 8), you will run the code inside, and then increment the value of "i" by 1, then run the condition again
for(var i = 0; i < temperatures.length; i++) {
// here, we are using the console.log statement to print the value held at temperatures[i] to the console
console.log(temperatures[i]);
}
("while" loop - the more difficult loop, because it's easy to forget to increment your counter, which can lead to browser-crashing infinite loops)
// declare your counter variable and initialize it to a value of 0
var i = 0;
// declare your condition, which states that for as long as your counter is less than the length of your temperatures array, you will run the code inside this code block, then check back again to see if the counter has yet matched the length of your temperatures array
while(i < temperatures.length) {
// here, we are using the console.log statement to print the value held at temperatures[i] to the console
console.log(temperatures[i]);
// THE MOST IMPORTANT PART OF YOUR WHILE LOOP IS THIS. without incrementing your counter variable's value, you will create an infinite loop that will crash your browser/app/whathaveyou
i++;
}
Hopefully this helps to refresh your memory!
Happy coding!
Erik

AYMERIC FIGEROU
3,748 PointsWe have exactly the same code, but it returns the following for me: There was an error with your code: TypeError: 'undefined' is not an object (evaluating 'console.log._args[i]')
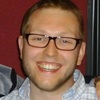
Victor Learned
6,976 PointsWhich part are you struggling with? Is it the creation of the loop? I would suggest just creating a for loop that prints out a message 10 times. Then from that change the parameters of the loop to depend on the length of the array. Remember how you can use the length-1 of an array within a for loop to iterate of the entire loop.