Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial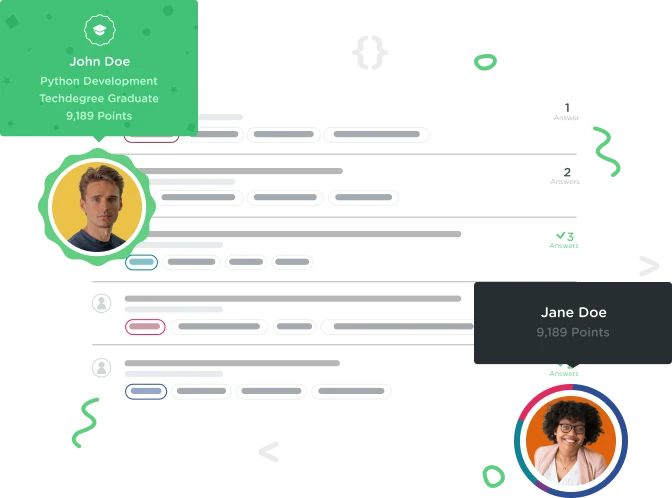

Mandy Rintala
9,046 PointsDo...While Objective
Having trouble with the objective for the Do...While Loop. I have tried every possiblity without having to add variables for it to make sense. JavaScript became very dense all of a sudden for me and cannot understand this language....I need help:(
2 Answers

Erik Nuber
20,629 Pointsvar secret;
do {
secret = prompt("what is the secret password?");
} while (secret !== "sesame");
document.write("You know the secret password. Welcome.");
A do while loop does what is inside the loop one time at least. This is different than other loops that check a condition and only if the condition is met will it execute the code inside.
So for this challenge, you declare the variable and, since it executes the code inside one time, within the do/while loop you prompt for the password.
The while loop itself still checks the condition after it goes thru the code one time and, if the condition is met it goes through it over again and checks etc...
If the condition is no longer met it moves on.
This is why here we use the !== operator as we are running the prompt while secret isn't equal to sesame.

nathanielcusano
9,808 Pointsvar secret = " ";
do {
secret = prompt("What is the secret password?");
}
while ( secret !== "sesame" ) {
}
document.write("You know the secret password. Welcome."); ```

nathanielcusano
9,808 Pointssetting the variable ,secret, to an empty string has no value. This allows the while loop to not equal sesame until the person enters "sesame" in the prompt.
Eric Thompson
9,858 PointsEric Thompson
9,858 PointsHello Mandy. JavaScript gets easier the more you are exposed to it. Trust me! This is actually my second time going through this course after completing the entire Front End Developer track. Everything is making more sense to me second time around (just went through Javascript Basics again last week).
As for the do while loop, I highly suggest you re-watch this video from the beginning. Dave lays it out in a very detailed way.
Think of your facebook account: The "do" part of the code is facebook asking you for your username and password. Then the "while" part kicks in:
While ("while") your login details do not match what fb has in their database, fb will continue to ask you over and over again until you provide the correct info (actually, after so many attempts fb may "break" out of the code and lock you out of your account, hahaha).
Once your login details match what fb has in their database, you then break out of the while loop and are let into the site.
With Dave's code example in the video, you are making JavaScript generate a random number and asking for a visitor (you in this case) to enter a number to see if it matches the random number generated. This is the "do" part of the code, and it will always run at least once.
The "while" part comes into play when the number you enter into the prompt box is not equal to the random number generated. It will keep running over, and over, and over again until your number and the random generated number match. At that point, the code breaks, and goes to the document.write part.
Don't let the "!correctGuess" part trip you up. Remember at the top of the code var correctGuess is set to "false". Basically, !correctGuess is saying "while correctGuess is not equal to false". Meaning, after the "do" part runs and gets to the "while" part, the code will ask "is correctGuess not equal to false (lets say you did not guess the random generated number this time around)?" If it is false (remember, var correctGuess = false), the code will continue to do the "do" loop.
Once your guess is the exact same number as the random generated number, !correctGuess is now "true" because var correctGuess is not equal to "false" anymore (see the part where Dave talks about "flags" around 04:40 in the video). The code breaks out of the loop and jumps down to the document.write part.
Does this help you understand what is going on a little better?