Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial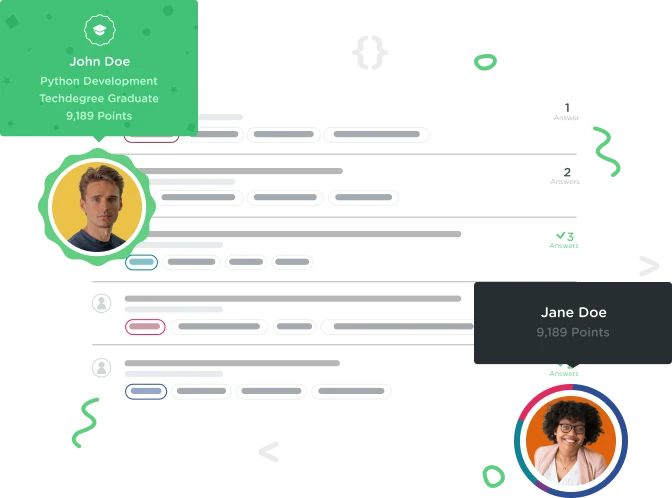

Anton Sorokin
Python Web Development Techdegree Student 2,173 PointsDramatically, I have "Bummer!" on submitting the code. What is wrong? I successfully tested function in Workspace.
The code is below:
def just_right(some_str): ls = len(some_str) if ls < 5: print("Your string is too short") elif ls > 6: print("Your string is too long") else: return True
def just_right(some_str):
ls = len(some_str)
if ls < 5:
print("Your string is too short")
elif ls > 6:
print("Your string is too long")
else:
return True
2 Answers

andren
28,558 PointsThere are two issues:
In your elif statement you are checking if the string length is greater than 6, the task is to check if it is greater than 5. For this specific task it actually won't matter whether you use 6 or 5 for this test, as the challenge accepts either. But your function will now accept both 5 and 6 as just right, which is technically not what the challenge intended.
The challenge wants you to return a string, not print it. For simple tasks like this return and print might seem to do the same thing (especially in the REPL where returned values are automatically printed) but they are actually quite different things. You will learn more about returning values later but for now just know that it is not the same as printing something.
If you fix those two issues like this:
def just_right(some_str):
ls = len(some_str)
if ls < 5:
return "Your string is too short"
elif ls > 5:
return "Your string is too long"
else:
return True
Then your code will pass.
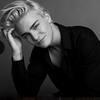
leonardbode
Courses Plus Student 4,011 PointsHey Anton,
Here is your code with my comments:
def just_right(some_str):
ls = len(some_str)
if ls < 5:
# You are printing instead of using result
print("Your string is too short")
# Task asks to check if string is greater than 5
elif ls > 6:
# Here again, you should return instead of print
print("Your string is too long")
else:
return True
Here is your code, edited:
def just_right(some_str):
ls = len(some_str)
if ls < 5:
return "Your string is too short"
elif ls > 5:
return "Your string is too long"
else:
return True
If you have any other questions I will update my answer, if you do not have any other questions:
Remember to upvote and to choose the best answer so that your question receives a checkmark in forums.
Kind regards,
Leo

Anton Sorokin
Python Web Development Techdegree Student 2,173 PointsThank you. I've got my mistake.